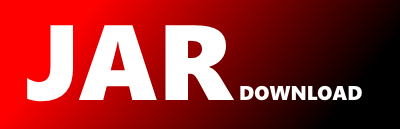
com.bigdata.service.DefaultClientDelegate Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bigdata-core Show documentation
Show all versions of bigdata-core Show documentation
Blazegraph(TM) DB Core Platform. It contains all Blazegraph DB dependencies other than Blueprints.
package com.bigdata.service;
import java.io.IOException;
import java.util.UUID;
import org.apache.log4j.Logger;
import com.bigdata.counters.AbstractStatisticsCollector;
import com.bigdata.counters.ICounterSetAccess;
import com.bigdata.counters.httpd.CounterSetHTTPD;
import com.bigdata.util.httpd.AbstractHTTPD;
/**
* Default {@link IFederationDelegate} implementation used by a standard client.
* This may be extended or replaced using
* {@link AbstractClient#setDelegate(IFederationDelegate)}.
*
* @author Bryan Thompson
* @version $Id$
*/
public class DefaultClientDelegate implements IFederationDelegate {
private static final Logger log = Logger.getLogger(DefaultClientDelegate.class);
private final UUID uuid = UUID.randomUUID();
private final IBigdataClient client;
private final T clientOrService;
/**
*
* @param client
* The client (before it connects to the federation).
* @param clientOrService
* The client or service connected to the federation. This MAY be
* null
but then {@link #getService()} will also
* return null
.
*/
public DefaultClientDelegate(final IBigdataClient client,
final T clientOrService) {
if (client == null)
throw new IllegalArgumentException();
if (clientOrService == null)
log.warn("Client or service not specified: " + this);
this.client = client;
this.clientOrService = clientOrService;
}
public T getService() {
return clientOrService;
}
/**
* Returns a stable but randomly assigned {@link UUID}.
*/
public UUID getServiceUUID() {
return uuid;
}
/**
* Return a stable identifier for this client based on the name of the
* implementation class, the hostname, and the hash code of the client
* (readable and likely to be unique, but uniqueness is not guaranteed).
*/
public String getServiceName() {
return client.getClass().getName() + "@"
+ AbstractStatisticsCollector.fullyQualifiedHostName + "#"
+ client.hashCode();
}
public Class getServiceIface() {
return client.getClass();
}
/**
* NOP.
*/
public void reattachDynamicCounters() {
}
/**
* Returns true
.
*/
public boolean isServiceReady() {
return true;
}
/**
* NOP
*/
public void didStart() {
}
/** NOP */
public void serviceJoin(IService service, UUID serviceUUID) {
}
/** NOP */
public void serviceLeave(UUID serviceUUID) {
}
public AbstractHTTPD newHttpd(final int httpdPort,
final ICounterSetAccess access) throws IOException {
return new CounterSetHTTPD(httpdPort, access);
// {
//
// public Response doGet(String uri, String method, Properties header,
// LinkedHashMap> parms)
// throws Exception {
//
// try {
//
// reattachDynamicCounters();
//
// } catch (Exception ex) {
//
// /*
// * Typically this is because the live journal has been
// * concurrently closed during the request.
// */
//
// log.warn("Could not re-attach dynamic counters: " + ex, ex);
//
// }
//
// return super.doGet(uri, method, header, parms);
//
// }
//
// };
}
}