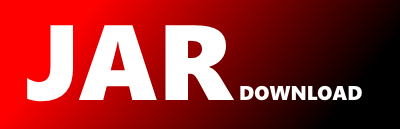
com.bigdata.rdf.graph.impl.bd.TestGather Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bigdata-rdf-test Show documentation
Show all versions of bigdata-rdf-test Show documentation
Blazegraph(TM) RDF Test Suites
/**
Copyright (C) SYSTAP, LLC DBA Blazegraph 2006-2016. All rights reserved.
Contact:
SYSTAP, LLC DBA Blazegraph
2501 Calvert ST NW #106
Washington, DC 20008
[email protected]
This program is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; version 2 of the License.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package com.bigdata.rdf.graph.impl.bd;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.Set;
import org.openrdf.model.Statement;
import org.openrdf.model.Value;
import com.bigdata.rdf.graph.EdgesEnum;
import com.bigdata.rdf.graph.Factory;
import com.bigdata.rdf.graph.FrontierEnum;
import com.bigdata.rdf.graph.IGASContext;
import com.bigdata.rdf.graph.IGASEngine;
import com.bigdata.rdf.graph.IGASScheduler;
import com.bigdata.rdf.graph.IGASState;
import com.bigdata.rdf.graph.IGraphAccessor;
import com.bigdata.rdf.graph.impl.BaseGASProgram;
import com.bigdata.rdf.graph.impl.GASStats;
import com.bigdata.rdf.model.BigdataValueFactory;
import com.bigdata.rdf.model.StatementEnum;
import com.bigdata.rdf.spo.SPO;
/**
* Test class for GATHER.
*
* @author Bryan Thompson
*/
@SuppressWarnings("rawtypes")
public class TestGather extends AbstractBigdataGraphTestCase {
public TestGather() {
}
public TestGather(String name) {
super(name);
}
/**
* Mock gather class uses a UNION for SUM to test the GATHER semantics.
* The gathered edge set is then APPLYed to the vertex and becomes the
* state of that vertex.
*
* @author Bryan Thompson
*/
private static class MockGASProgram extends
BaseGASProgram, Set, Set> {
private final EdgesEnum gatherEdges;
MockGASProgram(final EdgesEnum gatherEdges) {
this.gatherEdges = gatherEdges;
}
@Override
public FrontierEnum getInitialFrontierEnum() {
return FrontierEnum.SingleVertex;
}
@Override
public EdgesEnum getGatherEdges() {
return gatherEdges;
}
@Override
public EdgesEnum getScatterEdges() {
return EdgesEnum.NoEdges;
}
@Override
public Factory> getVertexStateFactory() {
return new Factory>() {
@Override
public Set initialValue(Value value) {
return new LinkedHashSet();
}
};
}
@Override
public Factory> getEdgeStateFactory() {
return null;
}
@Override
public void initVertex(
IGASContext, Set, Set> ctx,
IGASState, Set, Set> state,
Value u) {
// NOP
}
/**
* Return the edge as a singleton set.
*/
@Override
public Set gather(
final IGASState, Set, Set> state,
final Value u, final Statement e) {
return Collections.singleton(e);
}
/**
* Set UNION over the GATHERed edges.
*/
@Override
public Set sum(
final IGASState, Set, Set> state,
final Set left, final Set right) {
/*
* Note: This happens to preserve the visitation order. That is not
* essential, but it is nice.
*/
final Set tmp = new LinkedHashSet(left);
tmp.addAll(right);
return tmp;
}
/**
* UNION the gathered edges with those already decorating the vertex.
*/
@Override
public Set apply(
final IGASState, Set, Set> state,
final Value u, final Set sum) {
if (sum != null) {
// Get the state for that vertex.
final Set us = state.getState(u);
// UNION with the accumulant.
us.addAll(sum);
return us;
}
// No change.
return null;
}
@Override
public boolean isChanged(
IGASState, Set, Set> state, Value u) {
return true;
}
@Override
public void scatter(IGASState, Set, Set> state,
final IGASScheduler sch, Value u, Statement e) {
throw new UnsupportedOperationException();
}
@Override
public boolean nextRound(IGASContext ctx) {
return true;
}
};
public void testGather_inEdges() throws Exception {
final SmallGraphProblem p = setupSmallGraphProblem();
// gather no-edges for :mike
{
final Set expected = set();
doGatherTest(EdgesEnum.NoEdges, expected, p.getMike()/* startingVertex */);
}
final BigdataValueFactory vf = (BigdataValueFactory) getGraphFixture()
.getSail().getValueFactory();
// gather in-edges for :mike
{
final Set expected = set(//
(Statement) new SPO(p.getBryan(), p.getFoafKnows(), p.getMike(),
StatementEnum.Explicit)//
);
doGatherTest(EdgesEnum.InEdges, expected, p.getMike()/* startingVertex */);
}
// gather out-edges for :mike
{
final Set expected = set(//
(Statement) new SPO(p.getMike(), p.getRdfType(), p.getFoafPerson(),
StatementEnum.Explicit),//
(Statement) new SPO(p.getMike(), p.getFoafKnows(), p.getBryan(),
StatementEnum.Explicit)//
);
doGatherTest(EdgesEnum.OutEdges, expected, p.getMike()/* startingVertex */);
}
// gather all-edges for :mike
{
final Set expected = set(//
(Statement) new SPO(p.getBryan(), p.getFoafKnows(), p.getMike(),
StatementEnum.Explicit),//
(Statement) new SPO(p.getMike(), p.getRdfType(), p.getFoafPerson(),
StatementEnum.Explicit),//
(Statement) new SPO(p.getMike(), p.getFoafKnows(), p.getBryan(),
StatementEnum.Explicit)//
);
doGatherTest(EdgesEnum.AllEdges, expected, p.getMike()/* startingVertex */);
}
}
/**
* Start on a known vertex. Do one iteration. Verify that the GATHER
* populated the data structures on the mock object with the appropriate
* collections.
* @throws Exception
*/
protected void doGatherTest(final EdgesEnum gatherEdges,
final Set extends Statement> expected, final Value startingVertex)
throws Exception {
final IGASEngine gasEngine = getGraphFixture()
.newGASEngine(1/* nthreads */);
try {
final IGraphAccessor graphAccessor = getGraphFixture()
.newGraphAccessor(null/* connectionIsIgnored */);
final IGASContext, Set, Set> gasContext = gasEngine
.newGASContext(graphAccessor, new MockGASProgram(
gatherEdges));
final IGASState, Set, Set> gasState = gasContext
.getGASState();
// Initialize the froniter.
gasState.setFrontier(gasContext, startingVertex);
// Do one round.
gasContext.doRound(new GASStats());
/*
* Lookup the state for the starting vertex (this should be the only
* vertex whose state was modified since we did only one round).
*/
final Set actual = gasState.getState(startingVertex);
// Verify against the expected state.
assertSameEdges(expected, actual);
} finally {
gasEngine.shutdownNow();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy