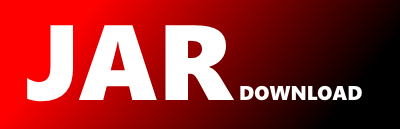
com.bigdata.rdf.sparql.ast.eval.TestTicket1105 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bigdata-rdf-test Show documentation
Show all versions of bigdata-rdf-test Show documentation
Blazegraph(TM) RDF Test Suites
/**
Copyright (C) SYSTAP, LLC DBA Blazegraph 2013. All rights reserved.
Contact:
SYSTAP, LLC DBA Blazegraph
2501 Calvert ST NW #106
Washington, DC 20008
[email protected]
This program is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; version 2 of the License.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package com.bigdata.rdf.sparql.ast.eval;
import java.util.Properties;
import junit.framework.Test;
import junit.framework.TestSuite;
import com.bigdata.rdf.sparql.ast.QuadsOperationInTriplesModeException;
import com.bigdata.rdf.store.AbstractTripleStore;
/**
* Test suite asserting that queries containint quads constructs (named graphs)
* are rejected in triples mode (at parsing phase), but go through in quads
* mode.
*
*
* @see
* SPARQL UPDATE should have nice error messages when namespace
* does not support named graphs
*
* @author Michael Schmidt
*/
public class TestTicket1105 extends AbstractDataDrivenSPARQLTestCase {
public TestTicket1105() {
}
public TestTicket1105(String name) {
super(name);
}
public static Test suite() {
final TestSuite suite = new TestSuite(
AbstractDataDrivenSPARQLTestCase.class.getSimpleName());
suite.addTestSuite(TestTriplesModeAPs.class);
suite.addTestSuite(TestQuadsModeAPs.class);
return suite;
}
/**
* Triples mode test suite.
*/
public static class TestTriplesModeAPs extends TestTicket1105 {
@Override
public Properties getProperties() {
final Properties properties = new Properties(super.getProperties());
// turn off quads.
properties.setProperty(AbstractTripleStore.Options.QUADS, "false");
// turn on triples
properties.setProperty(AbstractTripleStore.Options.TRIPLES_MODE,
"true");
return properties;
}
/**
* Query:
INSERT DATA
{
GRAPH
{ }
}
*
* throws an {@link QuadsOperationInTriplesModeException} in triples mode.
*
* @throws Exception
*/
public void test_ticket_1105_triples_update1() throws Exception {
try {
new UpdateTestHelper("ticket_1105_triples_update1", // testURI,
"ticket_1105_update1.rq",// queryFileURL
"ticket_1105.trig" // dataFileURL
);
} catch (QuadsOperationInTriplesModeException e) {
return; // expected
}
throw new RuntimeException("Exception expected, but not encountered");
}
/**
* Query:
INSERT
{ }
WHERE
{
GRAPH
{ }
}
*
* throws an {@link QuadsOperationInTriplesModeException} in triples mode.
*
* @throws Exception
*/
public void test_ticket_1105_triples_update2() throws Exception {
try {
new UpdateTestHelper("ticket_1105_triples_update2", // testURI,
"ticket_1105_update2.rq",// queryFileURL
"ticket_1105.trig"// dataFileURL
);
} catch (QuadsOperationInTriplesModeException e) {
return; // expected
}
throw new RuntimeException("Exception expected, but not encountered");
}
/**
* Query:
INSERT
{ }
WHERE
{
GRAPH ?g
{ }
}
*
* throws an {@link QuadsOperationInTriplesModeException} in triples mode.
*
* @throws Exception
*/
public void test_ticket_1105_triples_update3() throws Exception {
try {
new UpdateTestHelper("ticket_1105_triples_update3", // testURI,
"ticket_1105_update3.rq",// queryFileURL
"ticket_1105.trig"// dataFileURL
);
} catch (QuadsOperationInTriplesModeException e) {
return; // expected
}
throw new RuntimeException("Exception expected, but not encountered");
}
/**
* Query:
DELETE
{ }
WHERE
{
GRAPH
{ }
}
*
* throws an {@link QuadsOperationInTriplesModeException} in triples mode.
*
* @throws Exception
*/
public void test_ticket_1105_triples_update4() throws Exception {
try {
new UpdateTestHelper("ticket_1105_triples_update4", // testURI,
"ticket_1105_update4.rq",// queryFileURL
"ticket_1105.trig"// dataFileURL
);
} catch (QuadsOperationInTriplesModeException e) {
return; // expected
}
throw new RuntimeException("Exception expected, but not encountered");
}
/**
* Query:
DELETE
{ }
WHERE
{
GRAPH ?g
{ }
}
*
* throws an {@link QuadsOperationInTriplesModeException} in triples mode.
*
* @throws Exception
*/
public void test_ticket_1105_triples_update5() throws Exception {
try {
new UpdateTestHelper("ticket_1105_triples_update5", // testURI,
"ticket_1105_update5.rq",// queryFileURL
"ticket_1105.trig"// dataFileURL
);
} catch (QuadsOperationInTriplesModeException e) {
return; // expected
}
throw new RuntimeException("Exception expected, but not encountered");
}
/**
* Query:
INSERT { }
USING
WHERE { }
*
* throws an {@link QuadsOperationInTriplesModeException} in triples mode.
*
* @throws Exception
*/
public void test_ticket_1105_triples_update6() throws Exception {
try {
new UpdateTestHelper("ticket_1105_triples_update6", // testURI,
"ticket_1105_update6.rq",// queryFileURL
"ticket_1105.trig"// dataFileURL
);
} catch (QuadsOperationInTriplesModeException e) {
return; // expected
}
throw new RuntimeException("Exception expected, but not encountered");
}
/**
* Query:
SELECT ?s ?p ?o
FROM NAMED
WHERE { ?s ?p ?o }
*
* throws an {@link QuadsOperationInTriplesModeException} in triples mode.
*
* @throws Exception
*/
public void test_ticket_1105_triples_select1() throws Exception {
try {
new TestHelper("ticket_1105_triples_select1", // testURI,
"ticket_1105_select1.rq",// queryFileURL
"ticket_1105.trig",// dataFileURL
"ticket_1105.srx"// resultFileURL
).runTest();
} catch (QuadsOperationInTriplesModeException e) {
return; // expected
}
throw new RuntimeException("Exception expected, but not encountered");
}
/**
* Query:
SELECT ?s ?p ?o
WHERE { GRAPH { ?s ?p ?o } }
*
* throws an {@link QuadsOperationInTriplesModeException} in triples mode.
*
* @throws Exception
*/
public void test_ticket_1105_triples_select2() throws Exception {
try {
new TestHelper("ticket_1105_triples_select2", // testURI,
"ticket_1105_select2.rq",// queryFileURL
"ticket_1105.trig",// dataFileURL
"ticket_1105.srx"// resultFileURL
).runTest();
} catch (QuadsOperationInTriplesModeException e) {
return; // expected
}
throw new RuntimeException("Exception expected, but not encountered");
}
/**
* Query:
SELECT ?s ?p ?o
WHERE { GRAPH ?g { ?s ?p ?o } }
*
* throws an {@link QuadsOperationInTriplesModeException} in triples mode.
*
* @throws Exception
*/
public void test_ticket_1105_triples_select3() throws Exception {
try {
new TestHelper("ticket_1105_triples_select3", // testURI,
"ticket_1105_select3.rq",// queryFileURL
"ticket_1105.trig",// dataFileURL
"ticket_1105.srx"// resultFileURL
).runTest();
} catch (QuadsOperationInTriplesModeException e) {
return; // expected
}
throw new RuntimeException("Exception expected, but not encountered");
}
}
/**
* Quads mode test suite.
*/
public static class TestQuadsModeAPs extends TestTicket1105 {
/**
* Query:
INSERT DATA
{
GRAPH
{ }
}
*
* is parsed successfully in quads mode.
*
* @throws Exception
*/
public void test_ticket_1105_quads_update1() throws Exception {
new UpdateTestHelper("ticket_1105_quads_update1", // testURI,
"ticket_1105_update1.rq",// queryFileURL
"ticket_1105.trig" // dataFileURL
);
}
/**
* Query:
INSERT
{ }
WHERE
{
GRAPH
{ }
}
*
* is parsed successfully in quads mode.
*
* @throws Exception
*/
public void test_ticket_1105_quads_update2() throws Exception {
new UpdateTestHelper("ticket_1105_quads_update2", // testURI,
"ticket_1105_update2.rq",// queryFileURL
"ticket_1105.trig"// dataFileURL
);
}
/**
* Query:
INSERT
{ }
WHERE
{
GRAPH ?g
{ }
}
*
* is parsed successfully in quads mode.
*
* @throws Exception
*/
public void test_ticket_1105_quads_update3() throws Exception {
new UpdateTestHelper("ticket_1105_quads_update3", // testURI,
"ticket_1105_update3.rq",// queryFileURL
"ticket_1105.trig"// dataFileURL
);
}
/**
* Query:
DELETE
{ }
WHERE
{
GRAPH
{ }
}
*
* is parsed successfully in quads mode.
*
* @throws Exception
*/
public void test_ticket_1105_quads_update4() throws Exception {
new UpdateTestHelper("ticket_1105_quads_update4", // testURI,
"ticket_1105_update4.rq",// queryFileURL
"ticket_1105.trig"// dataFileURL
);
}
/**
* Query:
DELETE
{ }
WHERE
{
GRAPH ?g
{ }
}
*
* is parsed successfully in quads mode.
*
* @throws Exception
*/
public void test_ticket_1105_quads_update5() throws Exception {
new UpdateTestHelper("ticket_1105_quads_update5", // testURI,
"ticket_1105_update5.rq",// queryFileURL
"ticket_1105.trig"// dataFileURL
);
}
/**
* Query:
INSERT { }
USING
WHERE { }
*
* is parsed successfully in quads mode.
*
* @throws Exception
*/
public void test_ticket_1105_quads_update6() throws Exception {
new UpdateTestHelper("ticket_1105_quads_update6", // testURI,
"ticket_1105_update6.rq",// queryFileURL
"ticket_1105.trig"// dataFileURL
);
}
/**
* Query:
SELECT ?s ?p ?o
FROM NAMED
WHERE { ?s ?p ?o }
*
* runs fine in quads mode.
*
* @throws Exception
*/
public void test_ticket_1105_quads_select1() throws Exception {
new TestHelper("ticket_1105_quads_select1", // testURI,
"ticket_1105_select1.rq",// queryFileURL
"ticket_1105.trig",// dataFileURL
"ticket_1105.srx"// resultFileURL
).runTest();
}
/**
* Query:
SELECT ?s ?p ?o
WHERE { GRAPH { ?s ?p ?o } }
*
* runs fine in quads mode.
*
* @throws Exception
*/
public void test_ticket_1105_quads_select2() throws Exception {
new TestHelper("ticket_1105_quads_select2", // testURI,
"ticket_1105_select2.rq",// queryFileURL
"ticket_1105.trig",// dataFileURL
"ticket_1105.srx"// resultFileURL
).runTest();
}
/**
* Query:
SELECT ?s ?p ?o
WHERE { GRAPH ?g { ?s ?p ?o } }
*
* runs fine in quads mode.
*
* @throws Exception
*/
public void test_ticket_1105_quads_select3() throws Exception {
new TestHelper("ticket_1105_quads_select3", // testURI,
"ticket_1105_select3.rq",// queryFileURL
"ticket_1105.trig",// dataFileURL
"ticket_1105.srx"// resultFileURL
).runTest();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy