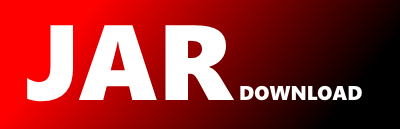
com.bigdata.rdf.sparql.ast.eval.TestTicket1007 Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bigdata-rdf-test Show documentation
Show all versions of bigdata-rdf-test Show documentation
Blazegraph(TM) RDF Test Suites
/**
Copyright (C) SYSTAP, LLC DBA Blazegraph 2013. All rights reserved.
Contact:
SYSTAP, LLC DBA Blazegraph
2501 Calvert ST NW #106
Washington, DC 20008
[email protected]
This program is free software; you can redistribute it and/or modify
it under the terms of the GNU General Public License as published by
the Free Software Foundation; version 2 of the License.
This program is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU General Public License for more details.
You should have received a copy of the GNU General Public License
along with this program; if not, write to the Free Software
Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package com.bigdata.rdf.sparql.ast.eval;
/**
* Various tests covering different constellations where values are constructed
* using BIND and reused in other parts of the query, such as
*
* - testing inlined vs. non-inlined configuration - using BIND prior to vs.
* after joining/filtering the variable - BINDing to values that are in the
* dictionary vs. BINDing to values that are not in the dictionary
*
* @see Ticket 1007: Using bound
* variables to refer to a graph
*
* @author Michael Schmidt
*/
public class TestTicket1007 extends AbstractDataDrivenSPARQLTestCase {
public TestTicket1007() {
}
public TestTicket1007(String name) {
super(name);
}
/**************************************************************************
*********************** ORIGINAL TICKET TESTS ****************************
**************************************************************************/
/**
* Original query as defined in bug report, reusing a URI constructed in a
* BIND clause in a join:
PREFIX :
SELECT * WHERE
{
GRAPH
{
:aProperty ?literal .
BIND (URI(CONCAT("http://www.interition.net/graphs/",?literal))
AS ?graph) .
}
GRAPH ?graph {
?s ?p ?o .
}
}
*
* Note: originally this query failed.
* @throws Exception
*/
public void test_ticket_1007() throws Exception {
new TestHelper("ticket-1007",// testURI,
"ticket-1007.rq",// queryFileURL
"ticket-1007.trig",// dataFileURL
"ticket-1007.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
* Modified query with join "on top", enforced through outer VALUES clause.
*
*
PREFIX :
SELECT * WHERE
{
GRAPH
{
:aProperty ?literal .
BIND (URI(CONCAT("http://www.interition.net/graphs/",?literal))
AS ?graph) .
}
}
VALUES ?graph { }
*
* @throws Exception
*/
public void test_ticket_1007b() throws Exception {
new TestHelper("ticket-1007b",// testURI,
"ticket-1007b.rq",// queryFileURL
"ticket-1007.trig",// dataFileURL
"ticket-1007b.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**************************************************************************
***************************** CUSTOM TESTS *******************************
**************************************************************************/
/*
* Dataset (trig) used in the tests defined in this section:
*
@prefix : .
@prefix rdf: .
@prefix rdfs: .
@prefix foaf: .
@prefix xsd: .
: {
:s :untypedString "untypedString" .
:s :typedString "typedString"^^xsd:unsignedByte .
:s :int "10"^^xsd:int .
:s :integer "10"^^xsd:integer .
:s :double "10.0"^^xsd:double .
:s :boolean "true"^^xsd:boolean .
:c :p5 "5"^^xsd:integer .
}
*
*/
/**
SELECT ?o
WHERE
{
?s ?p ?o
BIND (10 AS ?o)
}
*/
public void test_ticket_1007_number1() throws Exception {
new TestHelper("ticket-1007-number1",// testURI,
"ticket-1007-number1.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-number-integer.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
{
?s ?o
BIND ("10.00"^^ AS ?o)
}
*/
public void test_ticket_1007_number2() throws Exception {
new TestHelper("ticket-1007-number2",// testURI,
"ticket-1007-number2.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-number-double.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
{
?s ?o
BIND (10 AS ?o)
}
*/
public void test_ticket_1007_number3() throws Exception {
new TestHelper("ticket-1007-number3",// testURI,
"ticket-1007-number3.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-number-integer.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
{
?s ?o
BIND (2*5 AS ?o)
}
*/
public void test_ticket_1007_number4() throws Exception {
new TestHelper("ticket-1007-number4",// testURI,
"ticket-1007-number4.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-number-integer.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
{
?s ?o
BIND (xsd:integer("10") AS ?o)
}
*
* Note: originally this query failed.
*/
public void test_ticket_1007_number5() throws Exception {
new TestHelper("ticket-1007-number5",// testURI,
"ticket-1007-number5.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-number-integer.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
{
?s ?o .
?v .
BIND (?v*2 AS ?o)
}
*/
public void test_ticket_1007_number6() throws Exception {
new TestHelper("ticket-1007-number6",// testURI,
"ticket-1007-number6.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-number-integer.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
WHERE
{
?s ?p ?o
BIND ("untypedString" AS ?o)
}
*/
public void test_ticket_1007_string1() throws Exception {
new TestHelper("ticket-1007-string1",// testURI,
"ticket-1007-string1.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-string.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
WHERE
{
?s ?p ?o
BIND (CONCAT("untyped","String") AS ?o)
}
*
* Note: originally this query failed.
*/
public void test_ticket_1007_string2() throws Exception {
new TestHelper("ticket-1007-string2",// testURI,
"ticket-1007-string2.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-string.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
WHERE
{
?s ?p ?o
BIND (STRAFTER("XuntypedString","X") AS ?o)
}
*
* Note: originally this query failed.
*/
public void test_ticket_1007_string3() throws Exception {
new TestHelper("ticket-1007-string3",// testURI,
"ticket-1007-string3.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-string.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
WHERE
{
?s ?p ?o
{
SELECT ?o
WHERE
{
BIND (CONCAT("untyped","String") AS ?o)
}
}
}
*
* Note: originally this query failed.
*/
public void test_ticket_1007_string4() throws Exception {
new TestHelper("ticket-1007-string4",// testURI,
"ticket-1007-string4.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-string.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
WHERE
{
BIND (CONCAT("untyped","String") AS ?o)
}
*
* Note: originally this query failed.
*/
public void test_ticket_1007_string5() throws Exception {
new TestHelper("ticket-1007-string5",// testURI,
"ticket-1007-string5.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-string.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
WHERE
{
?s ?p ?o
BIND (URI("http://untypedString") AS ?o)
}
*/
public void test_ticket_1007_empty1() throws Exception {
new TestHelper("ticket-1007-empty1",// testURI,
"ticket-1007-empty1.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-empty.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
WHERE
{
?s ?p ?o
BIND ("10" AS ?o)
}
*/
public void test_ticket_1007_empty2() throws Exception {
new TestHelper("ticket-1007-empty2",// testURI,
"ticket-1007-empty2.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-empty.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT DISTINCT ?z
WHERE
{
?s ?p ?o
BIND (URI("http://untypedUri") AS ?z)
}
*/
public void test_ticket_1007_freshUri() throws Exception {
new TestHelper("ticket-1007-freshUri",// testURI,
"ticket-1007-freshUri.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-freshUri.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
WHERE
{
?s ?o
BIND (?s=?s AS ?o)
}
*/
public void test_ticket_1007_boolean1() throws Exception {
new TestHelper("ticket-1007-boolean1",// testURI,
"ticket-1007-boolean1.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-boolean.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
SELECT ?o
WHERE
{
?s ?o
BIND (?s= AS ?o)
}
*/
public void test_ticket_1007_boolean2() throws Exception {
new TestHelper("ticket-1007-boolean2",// testURI,
"ticket-1007-boolean2.rq",// queryFileURL
"ticket-1007-custom.trig",// dataFileURL
"ticket-1007-boolean.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
/**
* Test problems with BIND inside and reuse of variable outside of
* subquery.
*
*
SELECT DISTINCT *
{
{
SELECT ?annotatedSource WHERE {
hint:SubQuery hint:runOnce true .
?s ?p ?o .
FILTER(strstarts(?o,"annotated"))
BIND(concat(substr(?o,1,9),"Source") as ?annotatedSource)
}
}
?ss ?pp ?annotatedSource
} LIMIT 20
*
* @see here
* for more details
*/
public void test_ticket_1007_subquery() throws Exception {
new TestHelper("ticket-1007-subquery",// testURI,
"ticket-1007-subquery.rq",// queryFileURL
"ticket-1007-subquery.trig",// dataFileURL
"ticket-1007-subquery.srx",// resultFileURL
false // checkOrder (because only one solution)
).runTest();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy