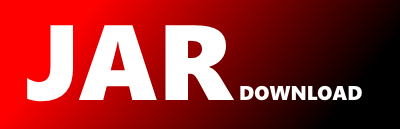
it.unimi.dsi.util.Intervals Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dsi-utils Show documentation
Show all versions of dsi-utils Show documentation
Blazegraph Modifications to the DSI utils. This are forked from version 1.10.0 under LGPLv2.1.
package it.unimi.dsi.util;
/*
* DSI utilities
*
* Copyright (C) 2003-2009 Paolo Boldi and Sebastiano Vigna
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as published by the Free
* Software Foundation; either version 2.1 of the License, or (at your option)
* any later version.
*
* This library is distributed in the hope that it will be useful, but
* WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY
* or FITNESS FOR A PARTICULAR PURPOSE. See the GNU Lesser General Public License
* for more details.
*
* You should have received a copy of the GNU Lesser General Public License
* along with this program; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place - Suite 330, Boston, MA 02111-1307, USA.
*
*/
import java.util.Comparator;
/** A class providing static methods and objects that do useful things with intervals.
*
* @see Interval
*/
public class Intervals {
private Intervals() {}
public final static Interval[] EMPTY_ARRAY = {};
/** An empty (singleton) interval. */
public final static Interval EMPTY_INTERVAL = new Interval( 1, 0 );
/** A singleton located at −&infty;. */
public final static Interval MINUS_INFINITY = new Interval( Integer.MIN_VALUE, Integer.MIN_VALUE );
/** A comparator between intervals defined as follows:
* [a..b] is less than [a'..b'] iff
* the first interval starts before or prolongs the second one, that is,
* iff a < a' or a=a' and b' < b.
*/
public final static Comparator STARTS_BEFORE_OR_PROLONGS = new Comparator() {
public int compare( final Interval i1, final Interval i2 ) {
if ( i1.left != i2.left ) return i1.left < i2.left ? -1 : 1;
if ( i1.right != i2.right ) return i2.right < i1.right ? -1 : 1;
return 0;
}
};
/** A comparator between intervals defined as follows:
* [a..b] is less than [a'..b'] iff
* the first interval ends before or is a suffix of the second one, that is,
* iff b < b' or b=b' and a' < a.
*/
public final static Comparator ENDS_BEFORE_OR_IS_SUFFIX = new Comparator() {
public int compare( final Interval i1, final Interval i2 ) {
if ( i1.right != i2.right ) return i1.right < i2.right ? -1 : 1;
if ( i1.left != i2.left ) return i2.left < i1.left ? -1 : 1;
return 0;
}
};
/** A comparator between intervals defined as follows:
* [a..b] is less than [a'..b']
* iff the first interval starts after the second one, that is,
* iff a' < a.
*/
public final static Comparator STARTS_AFTER = new Comparator() {
public int compare( final Interval i1, final Interval i2 ) {
if ( i1.left != i2.left ) return i2.left < i1.left ? -1 : 1;
return 0;
}
};
/** A comparator between intervals defined as follows:
* [a..b] is less than [a'..b']
* iff the first interval starts before the second one, that is,
* iff a' > a.
*/
public final static Comparator STARTS_BEFORE = new Comparator() {
public int compare( final Interval i1, final Interval i2 ) {
if ( i1.left != i2.left ) return i2.left < i1.left ? 1 : -1;
return 0;
}
};
/** A comparator between intervals defined as follows:
* [a..b] is less than [a'..b']
* iff the first interval ends after the second one, that is,
* iff b' < b.
*/
public final static Comparator ENDS_AFTER = new Comparator() {
public int compare( final Interval i1, final Interval i2 ) {
if ( i1.right != i2.right ) return i2.right < i1.right ? -1 : 1;
return 0;
}
};
/** A comparator between intervals defined as follows:
* [a..b] is less than [a'..b']
* iff the first interval ends before the second one, that is,
* iff b' > b.
*/
public final static Comparator ENDS_BEFORE = new Comparator() {
public int compare( final Interval i1, final Interval i2 ) {
if ( i1.right != i2.right ) return i2.right < i1.right ? 1 : -1;
return 0;
}
};
/** A comparator between intervals based on their length. */
public final static Comparator LENGTH_COMPARATOR = new Comparator() {
public int compare( final Interval i1, final Interval i2 ) {
return i1.length() - i2.length();
}
};
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy