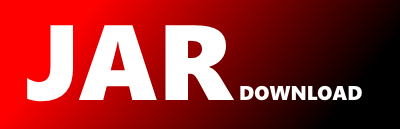
com.blazemeter.csv.RandomBufferedReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jmeter-plugins-random-csv-data-set Show documentation
Show all versions of jmeter-plugins-random-csv-data-set Show documentation
Config item that allows reading CSV files in random order
package com.blazemeter.csv;
import java.io.*;
import java.nio.channels.Channels;
public class RandomBufferedReader extends BufferedReader {
private final RandomAccessFile raf;
private InputStream is;
private BufferedReader reader;
private String encoding;
private long markedPosition;
public RandomBufferedReader(Reader in, RandomAccessFile raf, String encoding) throws UnsupportedEncodingException {
super(in);
this.raf = raf;
this.encoding = encoding;
this.is = Channels.newInputStream(raf.getChannel());
initBufferedReader();
}
private void initBufferedReader() throws UnsupportedEncodingException {
InputStreamReader isr = new InputStreamReader(is, encoding);
reader = new BufferedReader(isr);
}
public void seek(long pos) throws IOException {
this.raf.seek(pos);
initBufferedReader();
}
@Override
public int read() throws IOException {
return reader.read();
}
@Override
public void mark(int readAheadLimit) throws IOException {
markedPosition = raf.getFilePointer();
}
@Override
public void reset() throws IOException {
raf.seek(markedPosition);
}
@Override
public void close() throws IOException {
super.close();
is.close();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy