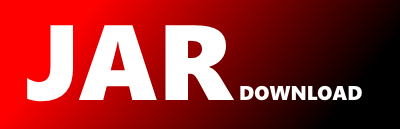
com.blazemeter.jmeter.TestRandomCSVAction Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jmeter-plugins-random-csv-data-set Show documentation
Show all versions of jmeter-plugins-random-csv-data-set Show documentation
Config item that allows reading CSV files in random order
package com.blazemeter.jmeter;
import org.apache.jmeter.engine.util.CompoundVariable;
import org.apache.jmeter.functions.InvalidVariableException;
import org.apache.jmeter.threads.JMeterContextService;
import org.apache.jmeter.threads.JMeterVariables;
import org.apache.jorphan.logging.LoggingManager;
import org.apache.jorphan.util.JMeterStopThreadException;
import org.apache.log.Logger;
import javax.swing.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class TestRandomCSVAction implements ActionListener {
private static final Logger LOGGER = LoggingManager.getLoggerForClass();
private static final int PREVIEW_MAX_SIZE = 20;
private final RandomCSVDataSetConfigGui randomCSVConfigGui;
public TestRandomCSVAction(RandomCSVDataSetConfigGui randomCSVConfigGui) {
this.randomCSVConfigGui = randomCSVConfigGui;
}
@Override
public void actionPerformed(ActionEvent event) {
final RandomCSVDataSetConfig config = (RandomCSVDataSetConfig) randomCSVConfigGui.createTestElement();
config.setRewindOnTheEndOfList(false);
config.setIndependentListPerThread(false);
JTextArea checkArea = randomCSVConfigGui.getCheckArea();
try {
config.setFilename(compoundValue(config.getFilename()));
config.setVariableNames(compoundValue(config.getVariableNames()));
config.setFileEncoding(compoundValue(config.getFileEncoding()));
config.setDelimiter(compoundValue(config.getDelimiter()));
JMeterVariables jMeterVariables = new JMeterVariables();
JMeterContextService.getContext().setVariables(jMeterVariables);
final List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy