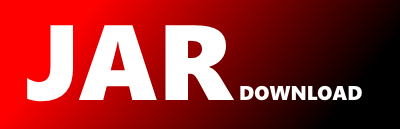
groovy.stream.MapStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of groovy-stream Show documentation
Show all versions of groovy-stream Show documentation
A collection of classes to give a fluent builder for Streams (Lazy Groovy Generators).
/*
* Copyright 2012 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package groovy.stream ;
import groovy.lang.Closure ;
import java.util.ArrayList ;
import java.util.HashMap ;
import java.util.Iterator ;
import java.util.LinkedHashMap ;
import java.util.List ;
import java.util.Map ;
import java.util.Set ;
import org.codehaus.groovy.runtime.typehandling.DefaultTypeTransformation ;
/**
* @author Tim Yates
*/
class MapStream> extends AbstractStream {
private Map iterators ;
private List keys ;
protected MapStream( Closure definition, Closure condition, Closure transform, LinkedHashMap using ) {
super( definition, condition, transform, using ) ;
}
@Override
protected void initialise() {
initial = this.definition.call() ;
iterators = new HashMap() ;
for( Map.Entry e : initial.entrySet() ) {
iterators.put( e.getKey(), e.getValue().iterator() ) ;
}
keys = new ArrayList( initial.keySet() ) ;
}
@SuppressWarnings("unchecked")
private T cloneMap( Map m ) {
return (T)new LinkedHashMap( m ) ;
}
@Override
public T next() {
T ret = cloneMap( (Map)current ) ;
transform.setDelegate( generateMapDelegate( using, (Map)current ) ) ;
loadNext() ;
this.streamIndex++ ;
return transform.call( ret ) ;
}
@SuppressWarnings("unchecked")
private T getFirst() {
Map newMap = new LinkedHashMap() ;
for( String key : keys ) {
newMap.put( key, iterators.get( key ).next() ) ;
}
return (T)newMap ;
}
@Override
@SuppressWarnings("unchecked")
protected void loadNext() {
while( !exhausted ) {
if( current == null ) {
current = getFirst() ;
}
else {
for( int i = keys.size() - 1 ; i >= 0 ; i-- ) {
String key = keys.get( i ) ;
if( iterators.get( key ).hasNext() ) {
((Map)current).put( key, iterators.get( key ).next() ) ;
break ;
}
else if( i > 0 ) {
iterators.put( key, initial.get( key ).iterator() ) ;
((Map)current).put( key, iterators.get( key ).next() ) ;
}
else {
exhausted = true ;
}
}
}
condition.setDelegate( generateMapDelegate( using, stopDelegate, (Map)current ) ) ;
Object cond = condition.call( current ) ;
if( cond == StreamStopper.getInstance() ) {
exhausted = true ;
}
else if( DefaultTypeTransformation.castToBoolean( cond ) ) {
break ;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy