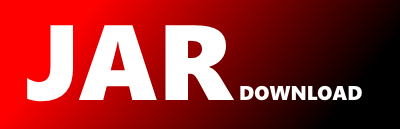
com.blossomproject.core.cache.BlossomCache Maven / Gradle / Ivy
package com.blossomproject.core.cache;
import com.github.benmanes.caffeine.cache.Cache;
import java.util.concurrent.Callable;
import org.springframework.cache.caffeine.CaffeineCache;
public class BlossomCache extends CaffeineCache {
private final CacheConfig configuration;
private boolean enabled;
public BlossomCache(String name, CacheConfig configuration, Cache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy