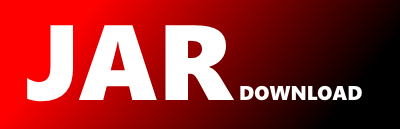
com.bloxbean.cardano.client.backend.gql.GqlAssetService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cardano-client-backend-gql Show documentation
Show all versions of cardano-client-backend-gql Show documentation
Cardano Client Library - GraphQL Backend
package com.bloxbean.cardano.client.backend.gql;
import com.bloxbean.cardano.client.backend.api.AssetService;
import com.bloxbean.cardano.client.api.common.OrderEnum;
import com.bloxbean.cardano.client.backend.model.Asset;
import com.bloxbean.cardano.client.backend.model.AssetAddress;
import com.bloxbean.cardano.client.backend.model.PolicyAsset;
import com.bloxbean.cardano.client.api.model.Result;
import com.bloxbean.cardano.gql.AssetQuery;
import okhttp3.OkHttpClient;
import java.math.BigInteger;
import java.util.List;
import java.util.Map;
public class GqlAssetService extends BaseGqlService implements AssetService {
public GqlAssetService(String gqlUrl) {
super(gqlUrl);
}
public GqlAssetService(String gqlUrl, Map headers) {
super(gqlUrl, headers);
}
public GqlAssetService(String gqlUrl, OkHttpClient client) {
super(gqlUrl, client);
}
@Override
public Result getAsset(String unit) {
AssetQuery query = new AssetQuery(unit);
AssetQuery.Data data = execute(query);
if (data == null)
return (Result) Result.error("No asset found for assetId: " + unit);
List assets = data.assets();
if (assets.size() == 0)
return (Result) Result.error("No asset found for assetId: " + unit);
AssetQuery.Asset gqlAsset = assets.get(0);
Asset asset = new Asset();
asset.setPolicyId(String.valueOf(gqlAsset.policyId()));
asset.setAssetName(String.valueOf(gqlAsset.assetName()));
asset.setFingerprint(String.valueOf(gqlAsset.fingerprint()));
BigInteger quantity = BigInteger.ZERO;
String initialMintHash;
try {
for (AssetQuery.TokenMint tokenMint : gqlAsset.tokenMints()) {
quantity = quantity.add(new BigInteger(tokenMint.quantity()));
}
initialMintHash = String.valueOf(gqlAsset.tokenMints().get(0).transaction().hash());
asset.setQuantity(quantity.toString());
asset.setInitialMintTxHash(initialMintHash);
} catch (Exception ignored) {
}
return processSuccessResult(asset);
}
@Override
public Result> getAssetAddresses(String asset, int count, int page, OrderEnum order) {
throw new UnsupportedOperationException();
}
@Override
public Result> getAssetAddresses(String asset, int count, int page) {
throw new UnsupportedOperationException();
}
@Override
public Result> getPolicyAssets(String policyId, int count, int page, OrderEnum order) {
throw new UnsupportedOperationException();
}
@Override
public Result> getPolicyAssets(String policyId, int count, int page) {
throw new UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy