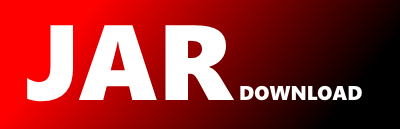
com.bloxbean.cardano.gql.NetworkInfoQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cardano-client-backend-gql Show documentation
Show all versions of cardano-client-backend-gql Show documentation
Cardano Client Library - GraphQL Backend
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package com.bloxbean.cardano.gql;
import com.apollographql.apollo.api.Operation;
import com.apollographql.apollo.api.OperationName;
import com.apollographql.apollo.api.Query;
import com.apollographql.apollo.api.Response;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.ScalarTypeAdapters;
import com.apollographql.apollo.api.internal.OperationRequestBodyComposer;
import com.apollographql.apollo.api.internal.QueryDocumentMinifier;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.SimpleOperationResponseParser;
import com.apollographql.apollo.api.internal.Utils;
import java.io.IOException;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Collections;
import okio.Buffer;
import okio.BufferedSource;
import okio.ByteString;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public final class NetworkInfoQuery implements Query {
public static final String OPERATION_ID = "8887f4f05542efe0d11db4d32320ca600bbf860341e9237a22b93ba45a9c2971";
public static final String QUERY_DOCUMENT = QueryDocumentMinifier.minify(
"query NetworkInfoQuery {\n"
+ " genesis {\n"
+ " __typename\n"
+ " shelley {\n"
+ " __typename\n"
+ " activeSlotsCoeff\n"
+ " epochLength\n"
+ " maxKESEvolutions\n"
+ " maxLovelaceSupply\n"
+ " networkId\n"
+ " networkMagic\n"
+ " securityParam\n"
+ " slotLength\n"
+ " slotsPerKESPeriod\n"
+ " systemStart\n"
+ " updateQuorum\n"
+ " }\n"
+ " }\n"
+ "}"
);
public static final OperationName OPERATION_NAME = new OperationName() {
@Override
public String name() {
return "NetworkInfoQuery";
}
};
private final Operation.Variables variables;
public NetworkInfoQuery() {
this.variables = Operation.EMPTY_VARIABLES;
}
@Override
public String operationId() {
return OPERATION_ID;
}
@Override
public String queryDocument() {
return QUERY_DOCUMENT;
}
@Override
public NetworkInfoQuery.Data wrapData(NetworkInfoQuery.Data data) {
return data;
}
@Override
public Operation.Variables variables() {
return variables;
}
@Override
public ResponseFieldMapper responseFieldMapper() {
return new Data.Mapper();
}
public static Builder builder() {
return new Builder();
}
@Override
public OperationName name() {
return OPERATION_NAME;
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return SimpleOperationResponseParser.parse(source, this, scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString,
@NotNull final ScalarTypeAdapters scalarTypeAdapters) throws IOException {
return parse(new Buffer().write(byteString), scalarTypeAdapters);
}
@Override
@NotNull
public Response parse(@NotNull final BufferedSource source) throws
IOException {
return parse(source, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public Response parse(@NotNull final ByteString byteString) throws
IOException {
return parse(byteString, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(@NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, false, true, scalarTypeAdapters);
}
@NotNull
@Override
public ByteString composeRequestBody() {
return OperationRequestBodyComposer.compose(this, false, true, ScalarTypeAdapters.DEFAULT);
}
@Override
@NotNull
public ByteString composeRequestBody(final boolean autoPersistQueries,
final boolean withQueryDocument, @NotNull final ScalarTypeAdapters scalarTypeAdapters) {
return OperationRequestBodyComposer.compose(this, autoPersistQueries, withQueryDocument, scalarTypeAdapters);
}
public static final class Builder {
Builder() {
}
public NetworkInfoQuery build() {
return new NetworkInfoQuery();
}
}
/**
* Data from the response after executing this GraphQL operation
*/
public static class Data implements Operation.Data {
static final ResponseField[] $responseFields = {
ResponseField.forObject("genesis", "genesis", null, false, Collections.emptyList())
};
final @NotNull Genesis genesis;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Data(@NotNull Genesis genesis) {
this.genesis = Utils.checkNotNull(genesis, "genesis == null");
}
public @NotNull Genesis genesis() {
return this.genesis;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeObject($responseFields[0], genesis.marshaller());
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Data{"
+ "genesis=" + genesis
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Data) {
Data that = (Data) o;
return this.genesis.equals(that.genesis);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= genesis.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Genesis.Mapper genesisFieldMapper = new Genesis.Mapper();
@Override
public Data map(ResponseReader reader) {
final Genesis genesis = reader.readObject($responseFields[0], new ResponseReader.ObjectReader() {
@Override
public Genesis read(ResponseReader reader) {
return genesisFieldMapper.map(reader);
}
});
return new Data(genesis);
}
}
}
public static class Genesis {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forObject("shelley", "shelley", null, true, Collections.emptyList())
};
final @NotNull String __typename;
final @Nullable Shelley shelley;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Genesis(@NotNull String __typename, @Nullable Shelley shelley) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.shelley = shelley;
}
public @NotNull String __typename() {
return this.__typename;
}
public @Nullable Shelley shelley() {
return this.shelley;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeObject($responseFields[1], shelley != null ? shelley.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Genesis{"
+ "__typename=" + __typename + ", "
+ "shelley=" + shelley
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Genesis) {
Genesis that = (Genesis) o;
return this.__typename.equals(that.__typename)
&& ((this.shelley == null) ? (that.shelley == null) : this.shelley.equals(that.shelley));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= (shelley == null) ? 0 : shelley.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Shelley.Mapper shelleyFieldMapper = new Shelley.Mapper();
@Override
public Genesis map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Shelley shelley = reader.readObject($responseFields[1], new ResponseReader.ObjectReader() {
@Override
public Shelley read(ResponseReader reader) {
return shelleyFieldMapper.map(reader);
}
});
return new Genesis(__typename, shelley);
}
}
}
public static class Shelley {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forDouble("activeSlotsCoeff", "activeSlotsCoeff", null, false, Collections.emptyList()),
ResponseField.forInt("epochLength", "epochLength", null, false, Collections.emptyList()),
ResponseField.forInt("maxKESEvolutions", "maxKESEvolutions", null, false, Collections.emptyList()),
ResponseField.forString("maxLovelaceSupply", "maxLovelaceSupply", null, false, Collections.emptyList()),
ResponseField.forString("networkId", "networkId", null, false, Collections.emptyList()),
ResponseField.forInt("networkMagic", "networkMagic", null, false, Collections.emptyList()),
ResponseField.forInt("securityParam", "securityParam", null, false, Collections.emptyList()),
ResponseField.forInt("slotLength", "slotLength", null, false, Collections.emptyList()),
ResponseField.forInt("slotsPerKESPeriod", "slotsPerKESPeriod", null, false, Collections.emptyList()),
ResponseField.forString("systemStart", "systemStart", null, false, Collections.emptyList()),
ResponseField.forInt("updateQuorum", "updateQuorum", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final double activeSlotsCoeff;
final int epochLength;
final int maxKESEvolutions;
final @NotNull String maxLovelaceSupply;
final @NotNull String networkId;
final int networkMagic;
final int securityParam;
final int slotLength;
final int slotsPerKESPeriod;
final @NotNull String systemStart;
final int updateQuorum;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Shelley(@NotNull String __typename, double activeSlotsCoeff, int epochLength,
int maxKESEvolutions, @NotNull String maxLovelaceSupply, @NotNull String networkId,
int networkMagic, int securityParam, int slotLength, int slotsPerKESPeriod,
@NotNull String systemStart, int updateQuorum) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.activeSlotsCoeff = activeSlotsCoeff;
this.epochLength = epochLength;
this.maxKESEvolutions = maxKESEvolutions;
this.maxLovelaceSupply = Utils.checkNotNull(maxLovelaceSupply, "maxLovelaceSupply == null");
this.networkId = Utils.checkNotNull(networkId, "networkId == null");
this.networkMagic = networkMagic;
this.securityParam = securityParam;
this.slotLength = slotLength;
this.slotsPerKESPeriod = slotsPerKESPeriod;
this.systemStart = Utils.checkNotNull(systemStart, "systemStart == null");
this.updateQuorum = updateQuorum;
}
public @NotNull String __typename() {
return this.__typename;
}
public double activeSlotsCoeff() {
return this.activeSlotsCoeff;
}
public int epochLength() {
return this.epochLength;
}
public int maxKESEvolutions() {
return this.maxKESEvolutions;
}
public @NotNull String maxLovelaceSupply() {
return this.maxLovelaceSupply;
}
public @NotNull String networkId() {
return this.networkId;
}
public int networkMagic() {
return this.networkMagic;
}
public int securityParam() {
return this.securityParam;
}
public int slotLength() {
return this.slotLength;
}
public int slotsPerKESPeriod() {
return this.slotsPerKESPeriod;
}
public @NotNull String systemStart() {
return this.systemStart;
}
public int updateQuorum() {
return this.updateQuorum;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeDouble($responseFields[1], activeSlotsCoeff);
writer.writeInt($responseFields[2], epochLength);
writer.writeInt($responseFields[3], maxKESEvolutions);
writer.writeString($responseFields[4], maxLovelaceSupply);
writer.writeString($responseFields[5], networkId);
writer.writeInt($responseFields[6], networkMagic);
writer.writeInt($responseFields[7], securityParam);
writer.writeInt($responseFields[8], slotLength);
writer.writeInt($responseFields[9], slotsPerKESPeriod);
writer.writeString($responseFields[10], systemStart);
writer.writeInt($responseFields[11], updateQuorum);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Shelley{"
+ "__typename=" + __typename + ", "
+ "activeSlotsCoeff=" + activeSlotsCoeff + ", "
+ "epochLength=" + epochLength + ", "
+ "maxKESEvolutions=" + maxKESEvolutions + ", "
+ "maxLovelaceSupply=" + maxLovelaceSupply + ", "
+ "networkId=" + networkId + ", "
+ "networkMagic=" + networkMagic + ", "
+ "securityParam=" + securityParam + ", "
+ "slotLength=" + slotLength + ", "
+ "slotsPerKESPeriod=" + slotsPerKESPeriod + ", "
+ "systemStart=" + systemStart + ", "
+ "updateQuorum=" + updateQuorum
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Shelley) {
Shelley that = (Shelley) o;
return this.__typename.equals(that.__typename)
&& Double.doubleToLongBits(this.activeSlotsCoeff) == Double.doubleToLongBits(that.activeSlotsCoeff)
&& this.epochLength == that.epochLength
&& this.maxKESEvolutions == that.maxKESEvolutions
&& this.maxLovelaceSupply.equals(that.maxLovelaceSupply)
&& this.networkId.equals(that.networkId)
&& this.networkMagic == that.networkMagic
&& this.securityParam == that.securityParam
&& this.slotLength == that.slotLength
&& this.slotsPerKESPeriod == that.slotsPerKESPeriod
&& this.systemStart.equals(that.systemStart)
&& this.updateQuorum == that.updateQuorum;
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= Double.valueOf(activeSlotsCoeff).hashCode();
h *= 1000003;
h ^= epochLength;
h *= 1000003;
h ^= maxKESEvolutions;
h *= 1000003;
h ^= maxLovelaceSupply.hashCode();
h *= 1000003;
h ^= networkId.hashCode();
h *= 1000003;
h ^= networkMagic;
h *= 1000003;
h ^= securityParam;
h *= 1000003;
h ^= slotLength;
h *= 1000003;
h ^= slotsPerKESPeriod;
h *= 1000003;
h ^= systemStart.hashCode();
h *= 1000003;
h ^= updateQuorum;
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public Shelley map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final double activeSlotsCoeff = reader.readDouble($responseFields[1]);
final int epochLength = reader.readInt($responseFields[2]);
final int maxKESEvolutions = reader.readInt($responseFields[3]);
final String maxLovelaceSupply = reader.readString($responseFields[4]);
final String networkId = reader.readString($responseFields[5]);
final int networkMagic = reader.readInt($responseFields[6]);
final int securityParam = reader.readInt($responseFields[7]);
final int slotLength = reader.readInt($responseFields[8]);
final int slotsPerKESPeriod = reader.readInt($responseFields[9]);
final String systemStart = reader.readString($responseFields[10]);
final int updateQuorum = reader.readInt($responseFields[11]);
return new Shelley(__typename, activeSlotsCoeff, epochLength, maxKESEvolutions, maxLovelaceSupply, networkId, networkMagic, securityParam, slotLength, slotsPerKESPeriod, systemStart, updateQuorum);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy