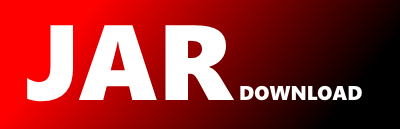
com.bloxbean.cardano.gql.fragment.BlockFragment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cardano-client-backend-gql Show documentation
Show all versions of cardano-client-backend-gql Show documentation
Cardano Client Library - GraphQL Backend
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package com.bloxbean.cardano.gql.fragment;
import com.apollographql.apollo.api.GraphqlFragment;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.Utils;
import com.bloxbean.cardano.gql.type.CustomType;
import java.lang.Integer;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Collections;
import org.jetbrains.annotations.NotNull;
import org.jetbrains.annotations.Nullable;
public class BlockFragment implements GraphqlFragment {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("forgedAt", "forgedAt", null, false, CustomType.DATETIME, Collections.emptyList()),
ResponseField.forInt("number", "number", null, true, Collections.emptyList()),
ResponseField.forCustomType("hash", "hash", null, false, CustomType.HASH32HEX, Collections.emptyList()),
ResponseField.forInt("slotNo", "slotNo", null, true, Collections.emptyList()),
ResponseField.forObject("epoch", "epoch", null, true, Collections.emptyList()),
ResponseField.forInt("slotInEpoch", "slotInEpoch", null, true, Collections.emptyList()),
ResponseField.forObject("slotLeader", "slotLeader", null, false, Collections.emptyList()),
ResponseField.forCustomType("size", "size", null, false, CustomType.BIGINT, Collections.emptyList()),
ResponseField.forString("transactionsCount", "transactionsCount", null, false, Collections.emptyList()),
ResponseField.forCustomType("fees", "fees", null, false, CustomType.BIGINT, Collections.emptyList()),
ResponseField.forCustomType("vrfKey", "vrfKey", null, true, CustomType.VRFVERIFICATIONKEY, Collections.emptyList()),
ResponseField.forObject("previousBlock", "previousBlock", null, true, Collections.emptyList()),
ResponseField.forObject("nextBlock", "nextBlock", null, true, Collections.emptyList())
};
public static final String FRAGMENT_DEFINITION = "fragment BlockFragment on Block {\n"
+ " __typename\n"
+ " forgedAt\n"
+ " number\n"
+ " hash\n"
+ " slotNo\n"
+ " epoch {\n"
+ " __typename\n"
+ " number\n"
+ " }\n"
+ " slotInEpoch\n"
+ " slotLeader {\n"
+ " __typename\n"
+ " hash\n"
+ " }\n"
+ " size\n"
+ " transactionsCount\n"
+ " fees\n"
+ " vrfKey\n"
+ " previousBlock {\n"
+ " __typename\n"
+ " hash\n"
+ " }\n"
+ " nextBlock {\n"
+ " __typename\n"
+ " hash\n"
+ " }\n"
+ "}";
final @NotNull String __typename;
final @NotNull Object forgedAt;
final @Nullable Integer number;
final @NotNull Object hash;
final @Nullable Integer slotNo;
final @Nullable Epoch epoch;
final @Nullable Integer slotInEpoch;
final @NotNull SlotLeader slotLeader;
final @NotNull Object size;
final @NotNull String transactionsCount;
final @NotNull Object fees;
final @Nullable Object vrfKey;
final @Nullable PreviousBlock previousBlock;
final @Nullable NextBlock nextBlock;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public BlockFragment(@NotNull String __typename, @NotNull Object forgedAt,
@Nullable Integer number, @NotNull Object hash, @Nullable Integer slotNo,
@Nullable Epoch epoch, @Nullable Integer slotInEpoch, @NotNull SlotLeader slotLeader,
@NotNull Object size, @NotNull String transactionsCount, @NotNull Object fees,
@Nullable Object vrfKey, @Nullable PreviousBlock previousBlock,
@Nullable NextBlock nextBlock) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.forgedAt = Utils.checkNotNull(forgedAt, "forgedAt == null");
this.number = number;
this.hash = Utils.checkNotNull(hash, "hash == null");
this.slotNo = slotNo;
this.epoch = epoch;
this.slotInEpoch = slotInEpoch;
this.slotLeader = Utils.checkNotNull(slotLeader, "slotLeader == null");
this.size = Utils.checkNotNull(size, "size == null");
this.transactionsCount = Utils.checkNotNull(transactionsCount, "transactionsCount == null");
this.fees = Utils.checkNotNull(fees, "fees == null");
this.vrfKey = vrfKey;
this.previousBlock = previousBlock;
this.nextBlock = nextBlock;
}
public @NotNull String __typename() {
return this.__typename;
}
public @NotNull Object forgedAt() {
return this.forgedAt;
}
public @Nullable Integer number() {
return this.number;
}
public @NotNull Object hash() {
return this.hash;
}
/**
* Ouroboros Classic Epoch Boundary blocks (EBB) do not have a slot number
*/
public @Nullable Integer slotNo() {
return this.slotNo;
}
/**
* Genesis block does not belong to the 0th epoch, therefore it could be null
*/
public @Nullable Epoch epoch() {
return this.epoch;
}
public @Nullable Integer slotInEpoch() {
return this.slotInEpoch;
}
public @NotNull SlotLeader slotLeader() {
return this.slotLeader;
}
public @NotNull Object size() {
return this.size;
}
public @NotNull String transactionsCount() {
return this.transactionsCount;
}
public @NotNull Object fees() {
return this.fees;
}
public @Nullable Object vrfKey() {
return this.vrfKey;
}
/**
* Ouroboros Classic Epoch Boundary blocks (EBB) do not have a slot number
*/
public @Nullable PreviousBlock previousBlock() {
return this.previousBlock;
}
public @Nullable NextBlock nextBlock() {
return this.nextBlock;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], forgedAt);
writer.writeInt($responseFields[2], number);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[3], hash);
writer.writeInt($responseFields[4], slotNo);
writer.writeObject($responseFields[5], epoch != null ? epoch.marshaller() : null);
writer.writeInt($responseFields[6], slotInEpoch);
writer.writeObject($responseFields[7], slotLeader.marshaller());
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[8], size);
writer.writeString($responseFields[9], transactionsCount);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[10], fees);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[11], vrfKey);
writer.writeObject($responseFields[12], previousBlock != null ? previousBlock.marshaller() : null);
writer.writeObject($responseFields[13], nextBlock != null ? nextBlock.marshaller() : null);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "BlockFragment{"
+ "__typename=" + __typename + ", "
+ "forgedAt=" + forgedAt + ", "
+ "number=" + number + ", "
+ "hash=" + hash + ", "
+ "slotNo=" + slotNo + ", "
+ "epoch=" + epoch + ", "
+ "slotInEpoch=" + slotInEpoch + ", "
+ "slotLeader=" + slotLeader + ", "
+ "size=" + size + ", "
+ "transactionsCount=" + transactionsCount + ", "
+ "fees=" + fees + ", "
+ "vrfKey=" + vrfKey + ", "
+ "previousBlock=" + previousBlock + ", "
+ "nextBlock=" + nextBlock
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof BlockFragment) {
BlockFragment that = (BlockFragment) o;
return this.__typename.equals(that.__typename)
&& this.forgedAt.equals(that.forgedAt)
&& ((this.number == null) ? (that.number == null) : this.number.equals(that.number))
&& this.hash.equals(that.hash)
&& ((this.slotNo == null) ? (that.slotNo == null) : this.slotNo.equals(that.slotNo))
&& ((this.epoch == null) ? (that.epoch == null) : this.epoch.equals(that.epoch))
&& ((this.slotInEpoch == null) ? (that.slotInEpoch == null) : this.slotInEpoch.equals(that.slotInEpoch))
&& this.slotLeader.equals(that.slotLeader)
&& this.size.equals(that.size)
&& this.transactionsCount.equals(that.transactionsCount)
&& this.fees.equals(that.fees)
&& ((this.vrfKey == null) ? (that.vrfKey == null) : this.vrfKey.equals(that.vrfKey))
&& ((this.previousBlock == null) ? (that.previousBlock == null) : this.previousBlock.equals(that.previousBlock))
&& ((this.nextBlock == null) ? (that.nextBlock == null) : this.nextBlock.equals(that.nextBlock));
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= forgedAt.hashCode();
h *= 1000003;
h ^= (number == null) ? 0 : number.hashCode();
h *= 1000003;
h ^= hash.hashCode();
h *= 1000003;
h ^= (slotNo == null) ? 0 : slotNo.hashCode();
h *= 1000003;
h ^= (epoch == null) ? 0 : epoch.hashCode();
h *= 1000003;
h ^= (slotInEpoch == null) ? 0 : slotInEpoch.hashCode();
h *= 1000003;
h ^= slotLeader.hashCode();
h *= 1000003;
h ^= size.hashCode();
h *= 1000003;
h ^= transactionsCount.hashCode();
h *= 1000003;
h ^= fees.hashCode();
h *= 1000003;
h ^= (vrfKey == null) ? 0 : vrfKey.hashCode();
h *= 1000003;
h ^= (previousBlock == null) ? 0 : previousBlock.hashCode();
h *= 1000003;
h ^= (nextBlock == null) ? 0 : nextBlock.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
final Epoch.Mapper epochFieldMapper = new Epoch.Mapper();
final SlotLeader.Mapper slotLeaderFieldMapper = new SlotLeader.Mapper();
final PreviousBlock.Mapper previousBlockFieldMapper = new PreviousBlock.Mapper();
final NextBlock.Mapper nextBlockFieldMapper = new NextBlock.Mapper();
@Override
public BlockFragment map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Object forgedAt = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
final Integer number = reader.readInt($responseFields[2]);
final Object hash = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[3]);
final Integer slotNo = reader.readInt($responseFields[4]);
final Epoch epoch = reader.readObject($responseFields[5], new ResponseReader.ObjectReader() {
@Override
public Epoch read(ResponseReader reader) {
return epochFieldMapper.map(reader);
}
});
final Integer slotInEpoch = reader.readInt($responseFields[6]);
final SlotLeader slotLeader = reader.readObject($responseFields[7], new ResponseReader.ObjectReader() {
@Override
public SlotLeader read(ResponseReader reader) {
return slotLeaderFieldMapper.map(reader);
}
});
final Object size = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[8]);
final String transactionsCount = reader.readString($responseFields[9]);
final Object fees = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[10]);
final Object vrfKey = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[11]);
final PreviousBlock previousBlock = reader.readObject($responseFields[12], new ResponseReader.ObjectReader() {
@Override
public PreviousBlock read(ResponseReader reader) {
return previousBlockFieldMapper.map(reader);
}
});
final NextBlock nextBlock = reader.readObject($responseFields[13], new ResponseReader.ObjectReader() {
@Override
public NextBlock read(ResponseReader reader) {
return nextBlockFieldMapper.map(reader);
}
});
return new BlockFragment(__typename, forgedAt, number, hash, slotNo, epoch, slotInEpoch, slotLeader, size, transactionsCount, fees, vrfKey, previousBlock, nextBlock);
}
}
public static class Epoch {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forInt("number", "number", null, false, Collections.emptyList())
};
final @NotNull String __typename;
final int number;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public Epoch(@NotNull String __typename, int number) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.number = number;
}
public @NotNull String __typename() {
return this.__typename;
}
public int number() {
return this.number;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeInt($responseFields[1], number);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "Epoch{"
+ "__typename=" + __typename + ", "
+ "number=" + number
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Epoch) {
Epoch that = (Epoch) o;
return this.__typename.equals(that.__typename)
&& this.number == that.number;
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= number;
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public Epoch map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final int number = reader.readInt($responseFields[1]);
return new Epoch(__typename, number);
}
}
}
public static class SlotLeader {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("hash", "hash", null, false, CustomType.HASH28HEX, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull Object hash;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public SlotLeader(@NotNull String __typename, @NotNull Object hash) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.hash = Utils.checkNotNull(hash, "hash == null");
}
public @NotNull String __typename() {
return this.__typename;
}
public @NotNull Object hash() {
return this.hash;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], hash);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "SlotLeader{"
+ "__typename=" + __typename + ", "
+ "hash=" + hash
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof SlotLeader) {
SlotLeader that = (SlotLeader) o;
return this.__typename.equals(that.__typename)
&& this.hash.equals(that.hash);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= hash.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public SlotLeader map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Object hash = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
return new SlotLeader(__typename, hash);
}
}
}
public static class PreviousBlock {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("hash", "hash", null, false, CustomType.HASH32HEX, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull Object hash;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public PreviousBlock(@NotNull String __typename, @NotNull Object hash) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.hash = Utils.checkNotNull(hash, "hash == null");
}
public @NotNull String __typename() {
return this.__typename;
}
public @NotNull Object hash() {
return this.hash;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], hash);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "PreviousBlock{"
+ "__typename=" + __typename + ", "
+ "hash=" + hash
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof PreviousBlock) {
PreviousBlock that = (PreviousBlock) o;
return this.__typename.equals(that.__typename)
&& this.hash.equals(that.hash);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= hash.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public PreviousBlock map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Object hash = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
return new PreviousBlock(__typename, hash);
}
}
}
public static class NextBlock {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forCustomType("hash", "hash", null, false, CustomType.HASH32HEX, Collections.emptyList())
};
final @NotNull String __typename;
final @NotNull Object hash;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public NextBlock(@NotNull String __typename, @NotNull Object hash) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.hash = Utils.checkNotNull(hash, "hash == null");
}
public @NotNull String __typename() {
return this.__typename;
}
public @NotNull Object hash() {
return this.hash;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[1], hash);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "NextBlock{"
+ "__typename=" + __typename + ", "
+ "hash=" + hash
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof NextBlock) {
NextBlock that = (NextBlock) o;
return this.__typename.equals(that.__typename)
&& this.hash.equals(that.hash);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= hash.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public NextBlock map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final Object hash = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[1]);
return new NextBlock(__typename, hash);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy