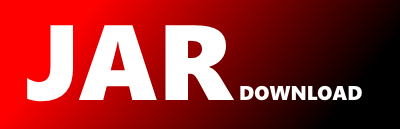
com.bloxbean.cardano.gql.fragment.EpochFragment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cardano-client-backend-gql Show documentation
Show all versions of cardano-client-backend-gql Show documentation
Cardano Client Library - GraphQL Backend
// AUTO-GENERATED FILE. DO NOT MODIFY.
//
// This class was automatically generated by Apollo GraphQL plugin from the GraphQL queries it found.
// It should not be modified by hand.
//
package com.bloxbean.cardano.gql.fragment;
import com.apollographql.apollo.api.GraphqlFragment;
import com.apollographql.apollo.api.ResponseField;
import com.apollographql.apollo.api.internal.ResponseFieldMapper;
import com.apollographql.apollo.api.internal.ResponseFieldMarshaller;
import com.apollographql.apollo.api.internal.ResponseReader;
import com.apollographql.apollo.api.internal.ResponseWriter;
import com.apollographql.apollo.api.internal.Utils;
import com.bloxbean.cardano.gql.type.CustomType;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.Collections;
import org.jetbrains.annotations.NotNull;
public class EpochFragment implements GraphqlFragment {
static final ResponseField[] $responseFields = {
ResponseField.forString("__typename", "__typename", null, false, Collections.emptyList()),
ResponseField.forInt("number", "number", null, false, Collections.emptyList()),
ResponseField.forCustomType("startedAt", "startedAt", null, false, CustomType.DATETIME, Collections.emptyList()),
ResponseField.forCustomType("lastBlockTime", "lastBlockTime", null, false, CustomType.DATETIME, Collections.emptyList()),
ResponseField.forString("blocksCount", "blocksCount", null, false, Collections.emptyList()),
ResponseField.forString("transactionsCount", "transactionsCount", null, false, Collections.emptyList()),
ResponseField.forString("output", "output", null, false, Collections.emptyList()),
ResponseField.forCustomType("fees", "fees", null, false, CustomType.BIGINT, Collections.emptyList())
};
public static final String FRAGMENT_DEFINITION = "fragment EpochFragment on Epoch {\n"
+ " __typename\n"
+ " number\n"
+ " startedAt\n"
+ " lastBlockTime\n"
+ " blocksCount\n"
+ " transactionsCount\n"
+ " output\n"
+ " fees\n"
+ "}";
final @NotNull String __typename;
final int number;
final @NotNull Object startedAt;
final @NotNull Object lastBlockTime;
final @NotNull String blocksCount;
final @NotNull String transactionsCount;
final @NotNull String output;
final @NotNull Object fees;
private transient volatile String $toString;
private transient volatile int $hashCode;
private transient volatile boolean $hashCodeMemoized;
public EpochFragment(@NotNull String __typename, int number, @NotNull Object startedAt,
@NotNull Object lastBlockTime, @NotNull String blocksCount, @NotNull String transactionsCount,
@NotNull String output, @NotNull Object fees) {
this.__typename = Utils.checkNotNull(__typename, "__typename == null");
this.number = number;
this.startedAt = Utils.checkNotNull(startedAt, "startedAt == null");
this.lastBlockTime = Utils.checkNotNull(lastBlockTime, "lastBlockTime == null");
this.blocksCount = Utils.checkNotNull(blocksCount, "blocksCount == null");
this.transactionsCount = Utils.checkNotNull(transactionsCount, "transactionsCount == null");
this.output = Utils.checkNotNull(output, "output == null");
this.fees = Utils.checkNotNull(fees, "fees == null");
}
public @NotNull String __typename() {
return this.__typename;
}
public int number() {
return this.number;
}
public @NotNull Object startedAt() {
return this.startedAt;
}
public @NotNull Object lastBlockTime() {
return this.lastBlockTime;
}
public @NotNull String blocksCount() {
return this.blocksCount;
}
public @NotNull String transactionsCount() {
return this.transactionsCount;
}
public @NotNull String output() {
return this.output;
}
public @NotNull Object fees() {
return this.fees;
}
@SuppressWarnings({"rawtypes", "unchecked"})
public ResponseFieldMarshaller marshaller() {
return new ResponseFieldMarshaller() {
@Override
public void marshal(ResponseWriter writer) {
writer.writeString($responseFields[0], __typename);
writer.writeInt($responseFields[1], number);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[2], startedAt);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[3], lastBlockTime);
writer.writeString($responseFields[4], blocksCount);
writer.writeString($responseFields[5], transactionsCount);
writer.writeString($responseFields[6], output);
writer.writeCustom((ResponseField.CustomTypeField) $responseFields[7], fees);
}
};
}
@Override
public String toString() {
if ($toString == null) {
$toString = "EpochFragment{"
+ "__typename=" + __typename + ", "
+ "number=" + number + ", "
+ "startedAt=" + startedAt + ", "
+ "lastBlockTime=" + lastBlockTime + ", "
+ "blocksCount=" + blocksCount + ", "
+ "transactionsCount=" + transactionsCount + ", "
+ "output=" + output + ", "
+ "fees=" + fees
+ "}";
}
return $toString;
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof EpochFragment) {
EpochFragment that = (EpochFragment) o;
return this.__typename.equals(that.__typename)
&& this.number == that.number
&& this.startedAt.equals(that.startedAt)
&& this.lastBlockTime.equals(that.lastBlockTime)
&& this.blocksCount.equals(that.blocksCount)
&& this.transactionsCount.equals(that.transactionsCount)
&& this.output.equals(that.output)
&& this.fees.equals(that.fees);
}
return false;
}
@Override
public int hashCode() {
if (!$hashCodeMemoized) {
int h = 1;
h *= 1000003;
h ^= __typename.hashCode();
h *= 1000003;
h ^= number;
h *= 1000003;
h ^= startedAt.hashCode();
h *= 1000003;
h ^= lastBlockTime.hashCode();
h *= 1000003;
h ^= blocksCount.hashCode();
h *= 1000003;
h ^= transactionsCount.hashCode();
h *= 1000003;
h ^= output.hashCode();
h *= 1000003;
h ^= fees.hashCode();
$hashCode = h;
$hashCodeMemoized = true;
}
return $hashCode;
}
public static final class Mapper implements ResponseFieldMapper {
@Override
public EpochFragment map(ResponseReader reader) {
final String __typename = reader.readString($responseFields[0]);
final int number = reader.readInt($responseFields[1]);
final Object startedAt = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[2]);
final Object lastBlockTime = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[3]);
final String blocksCount = reader.readString($responseFields[4]);
final String transactionsCount = reader.readString($responseFields[5]);
final String output = reader.readString($responseFields[6]);
final Object fees = reader.readCustomType((ResponseField.CustomTypeField) $responseFields[7]);
return new EpochFragment(__typename, number, startedAt, lastBlockTime, blocksCount, transactionsCount, output, fees);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy