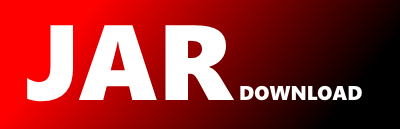
com.bloxbean.cardano.client.backend.api.AssetService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cardano-client-backend Show documentation
Show all versions of cardano-client-backend Show documentation
Cardano Client Lib Backend Api Module
package com.bloxbean.cardano.client.backend.api;
import com.bloxbean.cardano.client.api.common.OrderEnum;
import com.bloxbean.cardano.client.api.exception.ApiException;
import com.bloxbean.cardano.client.backend.model.*;
import com.bloxbean.cardano.client.api.model.Result;
import java.util.List;
public interface AssetService {
/**
* Get Asset
*
* @param unit Concatenation of the policy_id and hex-encoded asset_name
* @return {@link Asset}
* @throws ApiException
*/
Result getAsset(String unit) throws ApiException;
/**
* Asset addresses
* List of addresses containing a specific asset
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @return List of {@link AssetAddress}
*/
Result> getAllAssetAddresses(String asset) throws ApiException;
/**
* Asset addresses
* List of addresses containing a specific asset
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last
* @return List of {@link AssetAddress}
*/
Result> getAssetAddresses(String asset, int count, int page, OrderEnum order) throws ApiException;
/**
* Asset addresses
* List of addresses containing a specific asset ordered ascending from the point of view of the blockchain, not the page listing itself.
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @return List<AssetAddress>
*/
Result> getAssetAddresses(String asset, int count, int page) throws ApiException;
/**
* Assets of a specific policy
* List of asset minted under a specific policy
*
* @param policyId Specific policy_id (required)
* @return List of {@link PolicyAsset}
*/
Result> getAllPolicyAssets(String policyId) throws ApiException;
/**
* Assets of a specific policy
* List of asset minted under a specific policy
*
* @param policyId Specific policy_id (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last.
* @return List<PolicyAsset>>
*/
Result> getPolicyAssets(String policyId, int count, int page, OrderEnum order) throws ApiException;
/**
* Assets of a specific policy
* List of asset minted under a specific policy ordered ascending from the point of view of the blockchain, not the page listing itself.
*
* @param policyId Specific policy_id (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @return List<PolicyAsset>>
*/
Result> getPolicyAssets(String policyId, int count, int page) throws ApiException;
/**
* List of a specific asset transactions
*
* @param asset Concatenation of the policy_id and hex-encoded asset_name (required)
* @param count The number of results displayed on one page. (<=100).
* @param page The page number for listing the results.
* @param order The ordering of items from the point of view of the blockchain, not the page listing itself. By default, we return oldest first, newest last.
* @return a list of a specific asset transactions
* @throws ApiException
*/
Result> getTransactions(String asset, int count, int page, OrderEnum order) throws ApiException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy