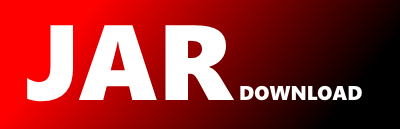
com.bloxbean.cardano.client.coinselection.UtxoSelectionStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cardano-client-coinselection Show documentation
Show all versions of cardano-client-coinselection Show documentation
Cardano Client Lib - Coinselection Module
package com.bloxbean.cardano.client.coinselection;
import com.bloxbean.cardano.client.api.exception.ApiException;
import com.bloxbean.cardano.client.api.model.Amount;
import com.bloxbean.cardano.client.api.model.Utxo;
import com.bloxbean.cardano.client.coinselection.config.CoinselectionConfig;
import com.bloxbean.cardano.client.plutus.spec.PlutusData;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Set;
/**
* Implement this interface to provide custom UtxoSelection Strategy
*/
public interface UtxoSelectionStrategy {
//TODO - cleanup
@Deprecated
default List selectUtxos(String address, String unit, BigInteger amount, Set utxosToExclude) throws ApiException {
return this.selectUtxos(address, unit, amount, null, utxosToExclude);
}
@Deprecated
default List selectUtxos(String address, String unit, BigInteger amount, String datumHash, Set utxosToExclude) throws ApiException {
Set selected = select(address, new Amount(unit, amount), datumHash, utxosToExclude);
return selected != null ? new ArrayList<>(selected) : Collections.emptyList();
}
default Set select(String address, Amount outputAmount, Set utxosToExclude) {
return select(address, Collections.singletonList(outputAmount), utxosToExclude, CoinselectionConfig.INSTANCE.getCoinSelectionLimit());
}
default Set select(String address, Amount outputAmount, Set utxosToExclude, int maxUtxoSelectionLimit) {
return select(address, Collections.singletonList(outputAmount), utxosToExclude, maxUtxoSelectionLimit);
}
default Set select(String address, List outputAmounts, Set utxosToExclude) {
return this.select(address, outputAmounts, utxosToExclude, CoinselectionConfig.INSTANCE.getCoinSelectionLimit());
}
default Set select(String address, List outputAmounts, Set utxosToExclude, int maxUtxoSelectionLimit) {
return this.select(address, outputAmounts, null, null, utxosToExclude, maxUtxoSelectionLimit);
}
@Deprecated
default Set select(String address, Amount outputAmount, String datumHash, Set utxosToExclude) {
return select(address, outputAmount, datumHash, utxosToExclude, CoinselectionConfig.INSTANCE.getCoinSelectionLimit());
}
@Deprecated
default Set select(String address, Amount outputAmount, String datumHash, Set utxosToExclude, int maxUtxoSelectionLimit) {
return select(address, Collections.singletonList(outputAmount), datumHash, null, utxosToExclude, maxUtxoSelectionLimit);
}
@Deprecated
default Set select(String address, List outputAmounts, String datumHash, Set utxosToExclude) {
return this.select(address, outputAmounts, datumHash, null, utxosToExclude, CoinselectionConfig.INSTANCE.getCoinSelectionLimit());
}
default Set selectByDatumHash(String address, Amount outputAmount, String datumHash, Set utxosToExclude) {
return selectByDatumHash(address, Collections.singletonList(outputAmount), datumHash, utxosToExclude, CoinselectionConfig.INSTANCE.getCoinSelectionLimit());
}
default Set selectByDatumHash(String address, Amount outputAmount, String datumHash, Set utxosToExclude, int maxUtxoSelectionLimit) {
return selectByDatumHash(address, Collections.singletonList(outputAmount), datumHash, utxosToExclude, maxUtxoSelectionLimit);
}
default Set selectByDatumHash(String address, List outputAmounts, String datumHash, Set utxosToExclude) {
return this.selectByDatumHash(address, outputAmounts, datumHash, utxosToExclude, CoinselectionConfig.INSTANCE.getCoinSelectionLimit());
}
default Set selectByDatumHash(String address, List outputAmounts, String datumHash, Set utxosToExclude, int maxUtxoSelectionLimit) {
return select(address, outputAmounts, datumHash, null, utxosToExclude, maxUtxoSelectionLimit);
}
default Set selectByInlineDatum(String address, Amount outputAmount, PlutusData inlineDatum, Set utxosToExclude) {
return selectByInlineDatum(address, Collections.singletonList(outputAmount), inlineDatum, utxosToExclude, CoinselectionConfig.INSTANCE.getCoinSelectionLimit());
}
default Set selectByInlineDatum(String address, Amount outputAmount, PlutusData inlineDatum, Set utxosToExclude, int maxUtxoSelectionLimit) {
return selectByInlineDatum(address, Collections.singletonList(outputAmount), inlineDatum, utxosToExclude, maxUtxoSelectionLimit);
}
default Set selectByInlineDatum(String address, List outputAmounts, PlutusData inlineDatum, Set utxosToExclude) {
return selectByInlineDatum(address, outputAmounts, inlineDatum, utxosToExclude, CoinselectionConfig.INSTANCE.getCoinSelectionLimit());
}
default Set selectByInlineDatum(String address, List outputAmounts, PlutusData inlineDatum, Set utxosToExclude, int maxUtxoSelectionLimit) {
return select(address, outputAmounts, null, inlineDatum, utxosToExclude, maxUtxoSelectionLimit);
}
default Set select(String address, Amount outputAmount, String datumHash, PlutusData inlineDatum, Set utxosToExclude, int maxUtxoSelectionLimit) {
return select(address, Collections.singletonList(outputAmount), datumHash, inlineDatum, utxosToExclude, maxUtxoSelectionLimit);
}
Set select(String address, List outputAmounts, String datumHash, PlutusData inlineDatum, Set utxosToExclude, int maxUtxoSelectionLimit);
UtxoSelectionStrategy fallback();
void setIgnoreUtxosWithDatumHash(boolean ignoreUtxosWithDatumHash);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy