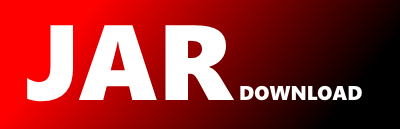
com.bloxbean.cardano.client.coinselection.UtxoSelector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cardano-client-coinselection Show documentation
Show all versions of cardano-client-coinselection Show documentation
Cardano Client Lib - Coinselection Module
package com.bloxbean.cardano.client.coinselection;
import com.bloxbean.cardano.client.api.exception.ApiException;
import com.bloxbean.cardano.client.api.model.Utxo;
import java.util.List;
import java.util.Optional;
import java.util.Set;
import java.util.function.Predicate;
/**
* Implement this interface to find Utxo
by predicate
*/
public interface UtxoSelector {
/**
* Find the first utxo matching the predicate
* @param address Script address
* @param predicate Predicate to filter utxos
* @return An optional with matching Utxo
* @throws ApiException if error
*/
Optional findFirst(String address, Predicate predicate) throws ApiException;
/**
* Find the first utxo matching the predicate
* @param address Script address
* @param predicate Predicate to filter utxos
* @param excludeUtxos Utxos to exclude
* @return An optional with matching Utxo
* @throws ApiException if error
*/
Optional findFirst(String address, Predicate predicate, Set excludeUtxos) throws ApiException;
/**
* Find all utxos matching the predicate
* @param address Script address
* @param predicate Predicate to filter utxos
* @return List of Utxos
* @throws ApiException if error
*/
List findAll(String address, Predicate predicate) throws ApiException;
/**
* Find all utxos matching the predicate
* @param address ScriptAddress Script address
* @param predicate Predicate Predicate to filter utxos
* @param excludeUtxos Utxos to exclude
* @return List of Utxos
* @throws ApiException if error
*/
List findAll(String address, Predicate predicate, Set excludeUtxos) throws ApiException;
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy