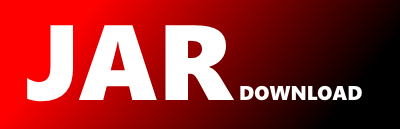
com.bloxbean.cardano.client.coinselection.impl.DefaultUtxoSelector Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cardano-client-coinselection Show documentation
Show all versions of cardano-client-coinselection Show documentation
Cardano Client Lib - Coinselection Module
package com.bloxbean.cardano.client.coinselection.impl;
import com.bloxbean.cardano.client.api.UtxoSupplier;
import com.bloxbean.cardano.client.api.common.OrderEnum;
import com.bloxbean.cardano.client.api.exception.ApiException;
import com.bloxbean.cardano.client.api.model.Utxo;
import com.bloxbean.cardano.client.coinselection.UtxoSelector;
import java.util.*;
import java.util.function.Predicate;
import java.util.stream.Collectors;
/**
* Default implementation of UtxoSelector
*/
public class DefaultUtxoSelector implements UtxoSelector {
private UtxoSupplier utxoSupplier;
public DefaultUtxoSelector(UtxoSupplier utxoSupplier) {
this.utxoSupplier = utxoSupplier;
}
@Override
public Optional findFirst(String address, Predicate predicate) throws ApiException {
return findFirst(address, predicate, Collections.EMPTY_SET);
}
@Override
public Optional findFirst(String address, Predicate predicate, Set excludeUtxos) throws ApiException {
boolean canContinue = true;
int i = 0; //Start with 0 here, for backend service counter start from 1 in DefaultUtxoSupplier
while (canContinue) {
List data = utxoSupplier.getPage(address, getUtxoFetchSize(),
i++, getUtxoFetchOrder());
if (data == null || data.isEmpty())
canContinue = false;
else {
Optional optional = data.stream()
.filter(predicate)
.filter(utxo -> !excludeUtxos.contains(utxo))
.findFirst();
if (optional.isPresent())
return optional;
}
}
return Optional.empty();
}
@Override
public List findAll(String address, Predicate predicate) throws ApiException {
return findAll(address, predicate, Collections.EMPTY_SET);
}
@Override
public List findAll(String address, Predicate predicate, Set excludeUtxos) throws ApiException {
boolean canContinue = true;
int i = 0;
List utxoList = new ArrayList<>();
while (canContinue) {
List data = utxoSupplier.getPage(address, getUtxoFetchSize(),
i++, getUtxoFetchOrder());
if (data == null || data.isEmpty())
canContinue = false;
else {
List filterUtxos = data.stream().filter(predicate)
.filter(utxo -> !excludeUtxos.contains(utxo))
.collect(Collectors.toList());
utxoList.addAll(filterUtxos);
}
}
return utxoList;
}
protected OrderEnum getUtxoFetchOrder() {
return OrderEnum.asc;
}
protected int getUtxoFetchSize() {
return 100;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy