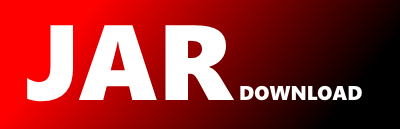
com.bloxbean.cardano.client.coinselection.impl.ExcludeUtxoSelectionStrategy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cardano-client-coinselection Show documentation
Show all versions of cardano-client-coinselection Show documentation
Cardano Client Lib - Coinselection Module
package com.bloxbean.cardano.client.coinselection.impl;
import com.bloxbean.cardano.client.api.model.Amount;
import com.bloxbean.cardano.client.api.model.Utxo;
import com.bloxbean.cardano.client.coinselection.UtxoSelectionStrategy;
import com.bloxbean.cardano.client.plutus.spec.PlutusData;
import com.bloxbean.cardano.client.transaction.spec.TransactionInput;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import java.util.stream.Collectors;
/**
* This is a wrapper {@link UtxoSelectionStrategy} implementation to exclude a list of Utxos from the selection process.
* This is useful when you want to exclude a list of Utxos from the selection process. The actual selection is delegated to the
* underlying {@link UtxoSelectionStrategy} implementation.
*/
public class ExcludeUtxoSelectionStrategy implements UtxoSelectionStrategy {
private UtxoSelectionStrategy utxoSelectionStrategy;
private Set excludeList;
public ExcludeUtxoSelectionStrategy(UtxoSelectionStrategy utxoSelectionStrategy, Set inputsToExclude) {
this.utxoSelectionStrategy = utxoSelectionStrategy;
if (inputsToExclude != null && !inputsToExclude.isEmpty()) {
excludeList = inputsToExclude.stream().map(input -> Utxo.builder()
.txHash(input.getTransactionId())
.outputIndex(input.getIndex())
.build()).collect(Collectors.toSet());
} else {
excludeList = Collections.emptySet();
}
}
@Override
public Set select(String address, List outputAmounts, String datumHash, PlutusData inlineDatum, Set utxosToExclude,
int maxUtxoSelectionLimit) {
Set finalUtxoToExclude;
if (utxosToExclude != null) {
finalUtxoToExclude = new HashSet<>(utxosToExclude);
finalUtxoToExclude.addAll(this.excludeList);
} else {
finalUtxoToExclude = this.excludeList;
}
return utxoSelectionStrategy.select(address, outputAmounts, datumHash, inlineDatum, finalUtxoToExclude, maxUtxoSelectionLimit);
}
@Override
public UtxoSelectionStrategy fallback() {
return utxoSelectionStrategy.fallback();
}
@Override
public void setIgnoreUtxosWithDatumHash(boolean ignoreUtxosWithDatumHash) {
utxoSelectionStrategy.setIgnoreUtxosWithDatumHash(ignoreUtxosWithDatumHash);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy