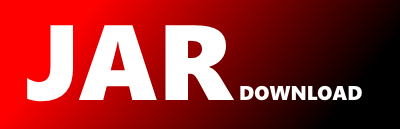
com.bluecatcode.common.collections.Collections3 Maven / Gradle / Ivy
package com.bluecatcode.common.collections;
import com.google.common.annotations.Beta;
import com.google.common.base.Function;
import com.google.common.collect.ImmutableMap;
import java.util.*;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.collect.Iterables.getOnlyElement;
public class Collections3 {
/**
* Combine two lists into a map. Lists must have the same size.
*
* @param keys the key list
* @param values the value list
* @param the key type
* @param the value type
* @return the resulting map
* @throws IllegalArgumentException if lists size differ
* @throws NullPointerException if any of the lists is null
*/
@Beta
public static Map zip(List keys, List values) {
checkArgument(keys != null, "Expected non-null keys");
checkArgument(values != null, "Expected non-null values");
checkArgument(keys.size() == values.size(), "Expected equal size of lists, got %s and %s", keys.size(), values.size());
ImmutableMap.Builder builder = ImmutableMap.builder();
for (int i = 0; i < keys.size(); i++) {
builder.put(keys.get(i), values.get(i));
}
return builder.build();
}
/**
* Convert a {@link Dictionary} to a {@link Map}
*
* @param dictionary the dictionary to convert
* @param the key type
* @param the value type
* @return the resulting map
*/
@Beta
public static Map fromDictionary(Dictionary dictionary) {
checkArgument(dictionary != null, "Expected non-null dictionary");
ImmutableMap.Builder builder = ImmutableMap.builder();
for (Enumeration keys = dictionary.keys(); keys.hasMoreElements(); ) {
K key = keys.nextElement();
V value = dictionary.get(key);
builder.put(key, value);
}
return builder.build();
}
/**
* Convert a {@link Dictionary} to a {@link Map} using a {@link Function}
*
* @param dictionary the dictionary to convert
* @param transformer the dictionary value transformer
* @param the key type
* @param the value type
* @return the resulting map
*/
@Beta
@SuppressWarnings("ConstantConditions")
public static Map fromDictionary(Dictionary dictionary, Function
© 2015 - 2024 Weber Informatics LLC | Privacy Policy