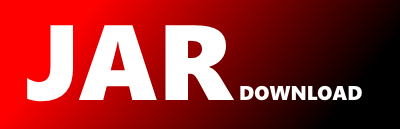
com.bluecatcode.common.concurrent.Try Maven / Gradle / Ivy
package com.bluecatcode.common.concurrent;
import com.bluecatcode.common.exceptions.WrappedException;
import com.bluecatcode.common.functions.Effect;
import com.google.common.base.Function;
import com.google.common.util.concurrent.SimpleTimeLimiter;
import com.google.common.util.concurrent.TimeLimiter;
import javax.annotation.CheckReturnValue;
import java.util.concurrent.Callable;
import java.util.concurrent.TimeUnit;
import static com.bluecatcode.common.base.Eithers.either;
import static com.bluecatcode.common.exceptions.Exceptions.uncheckedException;
import static com.bluecatcode.common.exceptions.Exceptions.unwrapToUncheckedException;
@CheckReturnValue
public class Try {
public static T tryWith(Callable callable) {
return tryWith(callable, unwrapToUncheckedException());
}
public static T tryWith(Callable callable, Function exceptionFunction) {
return either(callable).orThrow(exceptionFunction);
}
public static void tryWith(Effect effect) {
try {
effect.cause();
} catch (Exception e) {
throw uncheckedException().apply(e);
}
}
public static T tryWith(long timeoutDuration, TimeUnit timeoutUnit, Callable callable) {
try {
TimeLimiter limiter = new SimpleTimeLimiter();
//noinspection unchecked
Callable proxy = limiter.newProxy(callable, Callable.class, timeoutDuration, timeoutUnit);
return proxy.call();
} catch (Exception e) {
throw uncheckedException().apply(e);
}
}
public static void tryWith(long timeoutDuration, TimeUnit timeoutUnit, Effect effect) {
try {
TimeLimiter limiter = new SimpleTimeLimiter();
//noinspection unchecked
Effect proxy = limiter.newProxy(effect, Effect.class, timeoutDuration, timeoutUnit);
proxy.cause();
} catch (Exception e) {
throw uncheckedException().apply(e);
}
}
private Try() {
throw new UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy