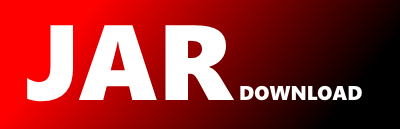
com.bluejeans.common.utils.BigQueue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of utils Show documentation
Show all versions of utils Show documentation
Bluejeans common utilities
/*
* Copyright Blue Jeans Network.
*/
package com.bluejeans.common.utils;
import java.io.Serializable;
import java.util.Collection;
import java.util.List;
import org.apache.commons.lang.SerializationUtils;
/**
* Big queue extension
*
* @author Dinesh Ilindra
* @param
* the entity type
*/
public class BigQueue extends com.bluejeans.common.bigqueue.BigQueue {
private Class entityType;
public BigQueue(final String queueDir, final String queueName, final int pageSize) {
super(queueDir, queueName, pageSize);
}
public BigQueue(final String queueDir, final String queueName) {
super(queueDir, queueName);
}
public BigQueue(final String queueDir, final String queueName, final int pageSize, final Class entityType) {
super(queueDir, queueName, pageSize);
this.entityType = entityType;
}
public BigQueue(final String queueDir, final String queueName, final Class entityType) {
super(queueDir, queueName);
this.entityType = entityType;
}
@SuppressWarnings("unchecked")
public void push(final E element) {
if (entityType == null) {
synchronized (this) {
if (entityType == null) {
entityType = (Class) element.getClass();
}
}
}
if (entityType.equals(byte[].class)) {
enqueue((byte[]) element);
} else if (entityType.equals(String.class)) {
enqueue(((String) element).getBytes());
} else {
enqueue(SerializationUtils.serialize((Serializable) element));
}
}
public E pop() {
return element(dequeue());
}
@SuppressWarnings("unchecked")
public E element(final byte[] data) {
if (data != null) {
if (entityType == null) {
synchronized (this) {
if (entityType == null) {
entityType = (Class) SerializationUtils.deserialize(data).getClass();
}
}
}
if (entityType.equals(byte[].class)) {
return (E) data;
} else if (entityType.equals(String.class)) {
return (E) new String(data);
} else {
return (E) SerializationUtils.deserialize(data);
}
} else {
return null;
}
}
public int drainTo(final Collection super E> c, final int maxElements) {
if (c == null) {
throw new NullPointerException();
}
if (c == this) {
throw new IllegalArgumentException();
}
final List elements = dequeueMulti(maxElements);
for (final byte[] data : elements) {
c.add(element(data));
}
return elements.size();
}
public int peekTo(final Collection super E> c, final int maxElements) {
if (c == null) {
throw new NullPointerException();
}
if (c == this) {
throw new IllegalArgumentException();
}
final List elements = peekMulti(maxElements);
for (final byte[] data : elements) {
c.add(element(data));
}
return elements.size();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy