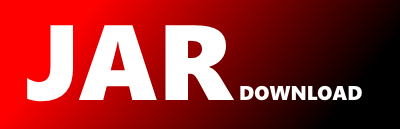
com.bluejeans.utils.zookeeper.ZookeeperUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of zookeeper-utils Show documentation
Show all versions of zookeeper-utils Show documentation
Bluejeans Zookeeper utilities
The newest version!
package com.bluejeans.utils.zookeeper;
import java.io.IOException;
import java.util.List;
import org.apache.curator.framework.CuratorFramework;
import org.apache.curator.framework.recipes.cache.PathChildrenCache;
import org.apache.curator.framework.recipes.cache.PathChildrenCache.StartMode;
import org.apache.curator.framework.recipes.cache.PathChildrenCacheListener;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ZookeeperUtils {
private static final Logger logger = LoggerFactory.getLogger(ZookeeperUtils.class);
public static PathChildrenCache getPathChildrenCache(CuratorFramework client, String path) {
return getPathChildrenCache(client, path, false, null);
}
public static PathChildrenCache getPathChildrenCache(CuratorFramework client, String path, boolean cacheData) {
return getPathChildrenCache(client, path, cacheData, null);
}
public static PathChildrenCache getPathChildrenCache(CuratorFramework client, String path, boolean cacheData,
PathChildrenCacheListener listener) {
// SG : Is a pool required ?
// ExecutorService pool = Executors.newFixedThreadPool(5, new
// ThreadFactory() {
// @Override
// public Thread newThread(Runnable runnable) {
// Thread thread = Executors.defaultThreadFactory().newThread(runnable);
// thread.setDaemon(true);
// return thread;
// }
// });
PathChildrenCache cache = new PathChildrenCache(client, path, cacheData);
if (listener != null) {
cache.getListenable().addListener(listener);
}
try {
cache.start(StartMode.BUILD_INITIAL_CACHE);
} catch (Exception e) {
logger.error("Failed to create PathChildrenCache for {}", e);
}
return cache;
}
public static void closePathChildrenCache(PathChildrenCache pathChildrenCache) {
if (pathChildrenCache != null) {
try {
pathChildrenCache.close();
} catch (IOException e) {
logger.error("IOException in closing pathChildrenCache {}", e);
}
}
}
public static byte[] getData(CuratorFramework client, String path) {
try {
return client.getData().forPath(path);
} catch (Exception e) {
logger.error("Failed to get data for {}", path, e);
}
return null;
}
public static List getChildren(CuratorFramework client, String path) {
try {
return client.getChildren().forPath(path);
} catch (Exception e) {
logger.error("Failed to get children for {}", path, e);
}
return null;
}
// public List sendSynchronousListRequest(String subPath) {
// try {
// return client.getChildren().forPath(path.concat(subPath));
// } catch (Exception e) {
// logger.error("Exception while fetching list from zookeeper: {} ", e);
// }
// return null;
// }
//
// public String sendSynchronousRequest(String subPath) {
// try {
// String data = new String(client.getData().forPath(path.concat(subPath)));
// return extractAddress(data);
// } catch (Exception e) {
// logger.error("Exception while fetching address from zookeeper: {}", e);
// }
// return null;
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy