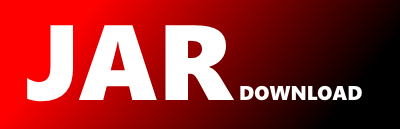
com.bluenimble.platform.query.impls.EventedQueryCompiler Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.bluenimble.platform.query.impls;
import java.util.Iterator;
import java.util.List;
import com.bluenimble.platform.query.CompiledQuery;
import com.bluenimble.platform.query.Condition;
import com.bluenimble.platform.query.Filter;
import com.bluenimble.platform.query.GroupBy;
import com.bluenimble.platform.query.OrderBy;
import com.bluenimble.platform.query.OrderByField;
import com.bluenimble.platform.query.Query;
import com.bluenimble.platform.query.QueryCompiler;
import com.bluenimble.platform.query.QueryException;
import com.bluenimble.platform.query.Select;
import com.bluenimble.platform.query.Query.Conjunction;
public abstract class EventedQueryCompiler implements QueryCompiler {
private static final long serialVersionUID = -4226119119102167983L;
protected enum Timing {
start,
end
}
@Override
public CompiledQuery compile (Query query) throws QueryException {
onQuery (Timing.start, query);
// where
processSelect (query.select ());
// where
processFilter (query.where (), Conjunction.and, true);
// group by
processGroupBy (query.groupBy ());
// order by
processOrderBy (query.orderBy ());
onQuery (Timing.end, query);
return done ();
}
private void processSelect (Select select) throws QueryException {
onSelect (Timing.start, select);
if (select == null || select.isEmpty ()) {
onSelect (Timing.end, select);
return;
}
for (int i = 0; i < select.count (); i++) {
onSelectField (select.get (i), select.count (), i);
}
onSelect (Timing.end, select);
}
private void processFilter (Filter filter, Conjunction conjunction, boolean isWhere) throws QueryException {
onFilter (Timing.start, filter, conjunction, isWhere);
if (filter == null || filter.isEmpty ()) {
return;
}
Iterator fields = filter.conditions ();
if (fields == null) {
return;
}
int counter = 0;
while (fields.hasNext ()) {
String field = fields.next ();
Object condition = filter.get (field);
if (List.class.isAssignableFrom (condition.getClass ())) {
// check if it's 'and' or 'or'
Conjunction subConjunction = null;
try {
subConjunction = Conjunction.valueOf (field);
} catch (Exception ex) {
// ignore
continue;
}
@SuppressWarnings("unchecked")
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy