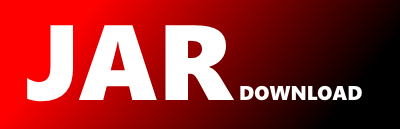
com.bmuschko.gradle.docker.utils.MainClassFinder.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gradle-docker-plugin Show documentation
Show all versions of gradle-docker-plugin Show documentation
Gradle plugin for managing Docker images and containers.
package com.bmuschko.gradle.docker.utils
import groovy.transform.CompileStatic
import org.objectweb.asm.AnnotationVisitor
import org.objectweb.asm.ClassReader
import org.objectweb.asm.ClassVisitor
import org.objectweb.asm.MethodVisitor
import org.objectweb.asm.Opcodes
import org.objectweb.asm.Type
/**
* Original source from Spring Boot's loader tools licensed under Apache License Version 2.0.
*/
@CompileStatic
class MainClassFinder {
private static final String DOT_CLASS = ".class"
private static final String MAIN_METHOD_NAME = "main"
private static final Type STRING_ARRAY_TYPE = Type.getType(String[].class)
private static final Type MAIN_METHOD_TYPE = Type.getMethodType(Type.VOID_TYPE, STRING_ARRAY_TYPE)
static String findSingleMainClass(File rootFolder, String annotationName) throws IOException {
SingleMainClassCallback callback = new SingleMainClassCallback(annotationName)
MainClassFinder.doWithMainClasses(rootFolder, callback)
callback.getMainClassName()
}
static final class MainClass {
private final String name
private final Set annotationNames
MainClass(String name, Set annotationNames) {
this.name = name
this.annotationNames = annotationNames.asImmutable()
}
String getName() {
return this.name
}
Set getAnnotationNames() {
return this.annotationNames
}
@Override
boolean equals(Object obj) {
if (this == obj) {
return true
}
if (obj == null) {
return false
}
if (getClass() != obj.getClass()) {
return false
}
MainClass other = (MainClass) obj
if (!this.name.equals(other.name)) {
return false
}
return true
}
@Override
int hashCode() {
return this.name.hashCode()
}
@Override
String toString() {
return this.name
}
}
interface MainClassCallback {
T doWith(MainClass mainClass)
}
private static final class SingleMainClassCallback implements MainClassCallback
© 2015 - 2025 Weber Informatics LLC | Privacy Policy