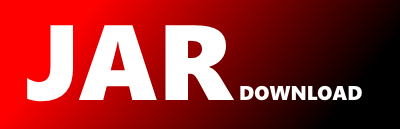
com.bnorm.infinite.StateMachineBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of infinite Show documentation
Show all versions of infinite Show documentation
Infinite - A hierarchical finite state machine for Java
package com.bnorm.infinite;
import java.util.Collections;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Set;
/**
* The base implementation of a state machine.
*
* @param the class type of the states.
* @param the class type of the events.
* @param the class type of the context.
* @author Brian Norman
* @version 1.0
* @since 1.0
*/
public class StateMachineBase implements StateMachine {
/** The state machine transition listeners. */
private final Set> listeners;
/** The state to internal state map. */
private final Map> states;
/** The event to transition map. */
private final Map>> transitions;
/** The current state of the state machine. */
private S state;
/** The context of the state machine. */
private final C context;
/**
* Constructs a new state machine from the specified state map, transition map, starting state, and context.
*
* @param states the states of the state machine.
* @param transitions the transitions of the state machine.
* @param starting the starting state of the state machine.
* @param context the state machine context.
*/
protected StateMachineBase(Map> states, Map>> transitions,
S starting, C context) {
this.listeners = new LinkedHashSet<>();
this.states = states;
this.transitions = transitions;
setState(starting);
this.context = context;
}
@Override
public void setState(S state) {
this.state = state;
}
@Override
public S getState() {
return state;
}
@Override
public C getContext() {
return context;
}
@Override
public InternalState getInternalState(S state) {
return states.get(state);
}
@Override
public Set> getTransitions(E event) {
return Collections.unmodifiableSet(transitions.getOrDefault(event, Collections.emptySet()));
}
@Override
public Set> getTransitionListeners() {
return Collections.unmodifiableSet(listeners);
}
@Override
public void addTransitionListener(TransitionListener super S, ? super E, ? super C> listener) {
listeners.add(listener);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy