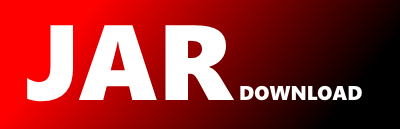
com.boozallen.aiops.mda.pattern.NativeInboundAndOutboundAsync Maven / Gradle / Ivy
package com.boozallen.aiops.mda.pattern;
/*-
* #%L
* aiSSEMBLE::Test::MDA::Data Delivery Spark
* %%
* Copyright (C) 2021 Booz Allen
* %%
* This software package is licensed under the Booz Allen Public License. All Rights Reserved.
* #L%
*/
import org.apache.spark.sql.Dataset;
import org.apache.spark.sql.Row;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
import javax.enterprise.context.ApplicationScoped;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import io.smallrye.mutiny.Multi;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.CompletionStage;
import java.time.Instant;
import com.boozallen.aissemble.core.metadata.MetadataModel;
/**
* The Reactive Messaging connector consumes one message at a time - this class writes
* multiple source data records within one message so that they can be ingested
* in batches.
*
* Because this class is {@link ApplicationScoped}, exactly one managed singleton instance will exist
* in any deployment.
*
* GENERATED STUB CODE - PLEASE ***DO*** MODIFY
*
* Originally generated from: templates/data-delivery-spark/asynchronous.processor.impl.java.vm
*/
@ApplicationScoped
public class NativeInboundAndOutboundAsync extends NativeInboundAndOutboundAsyncBase {
private static final Logger logger = LoggerFactory.getLogger(NativeInboundAndOutboundAsync.class);
protected static final int BATCH_SIZE = config.batchSize();
public NativeInboundAndOutboundAsync() {
super("asynchronous", getDataActionDescriptiveLabel());
}
/**
* Provides a descriptive label for the action that can be used for logging (e.g., provenance details).
*
* @return descriptive label
*/
private static String getDataActionDescriptiveLabel() {
// TODO: replace with descriptive label
return "NativeInboundAndOutboundAsync";
}
/**
* {@inheritDoc}
*/
@Override
protected CompletionStage> executeStepImpl(Dataset inbound) {
/* Asynchronous steps return CompletionStage types to support
asynchronous processing. Synchronous results can be returned as CompletableFuture.completedFuture({value})
while asynchronous processing can be achieved with CompletableFuture run/supply async methods or any other
compatible implementation. See https://smallrye.io/smallrye-reactive-messaging/smallrye-reactive-messaging/2/model/model.html
for additional details
*/
// TODO add processing logic here
return CompletableFuture.completedFuture(null);
}
/**
* {@inheritDoc}
*/
@Override
protected MetadataModel createProvenanceMetadata(String resource, String subject, String action) {
// TODO: Add any additional provenance-related metadata here
return new MetadataModel(resource, subject, action, Instant.now());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy