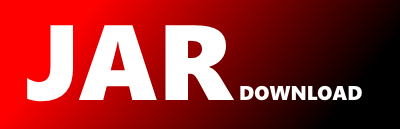
com.botronsoft.cmj.spitools.impl.transformation.parsers.MultiValueArgumentParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of configuration-manager-spi-tools Show documentation
Show all versions of configuration-manager-spi-tools Show documentation
Configuration Manager Service Provider Interface Tools
package com.botronsoft.cmj.spitools.impl.transformation.parsers;
import java.util.List;
import java.util.Optional;
import java.util.regex.Pattern;
import com.botronsoft.cmj.spitools.impl.transformation.configuration.Configuration;
import com.google.common.collect.Lists;
/**
* Takes care of parsing a multi-value property. The value will be split using the separator and quotes will be removed.
*/
public class MultiValueArgumentParser {
public Optional> parse(String value, Configuration configuration) {
if ((value == null) || configuration.getLiteralValues().contains(value) || !configuration.getSeparator().isPresent()) {
return Optional.empty();
}
List result = Lists.newArrayList();
if (!value.isEmpty()) {
String separator = configuration.getSeparator().get();
String regex = Pattern.quote(separator) // the separator to split
+ "(?x) " // enable comments, ignore white spaces
+ "(?= " // start positive look ahead
+ " ( " // start group 1
+ " [^\"]* " // match "other than quote" zero or more times
+ " \" " // match quote
+ " [^\"]* " // match "other than quote" zero or more times
+ " \" " // match quote
+ " )* " // end group 1 and repeat it 0 or more times
+ " [^\"]* " // match "other than quote"
+ " $ " // match the end of the string
+ ") ";// stop positive lookahead
for (String singleValue : value.split(regex)) {
result.add(singleValue.trim().replaceAll("\"", ""));
}
}
return Optional.of(result);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy