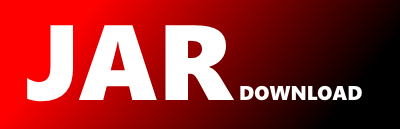
com.braintree.org.bouncycastle.util.io.Streams Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of encryption Show documentation
Show all versions of encryption Show documentation
Client side encryption for Braintree integrations on Android.
package com.braintree.org.bouncycastle.util.io;
import java.io.ByteArrayOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
public final class Streams
{
private static int BUFFER_SIZE = 512;
public static void drain(InputStream inStr)
throws IOException
{
byte[] bs = new byte[BUFFER_SIZE];
while (inStr.read(bs, 0, bs.length) >= 0)
{
}
}
public static byte[] readAll(InputStream inStr)
throws IOException
{
ByteArrayOutputStream buf = new ByteArrayOutputStream();
pipeAll(inStr, buf);
return buf.toByteArray();
}
public static byte[] readAllLimited(InputStream inStr, int limit)
throws IOException
{
ByteArrayOutputStream buf = new ByteArrayOutputStream();
pipeAllLimited(inStr, limit, buf);
return buf.toByteArray();
}
public static int readFully(InputStream inStr, byte[] buf)
throws IOException
{
return readFully(inStr, buf, 0, buf.length);
}
public static int readFully(InputStream inStr, byte[] buf, int off, int len)
throws IOException
{
int totalRead = 0;
while (totalRead < len)
{
int numRead = inStr.read(buf, off + totalRead, len - totalRead);
if (numRead < 0)
{
break;
}
totalRead += numRead;
}
return totalRead;
}
public static void pipeAll(InputStream inStr, OutputStream outStr)
throws IOException
{
byte[] bs = new byte[BUFFER_SIZE];
int numRead;
while ((numRead = inStr.read(bs, 0, bs.length)) >= 0)
{
outStr.write(bs, 0, numRead);
}
}
public static long pipeAllLimited(InputStream inStr, long limit, OutputStream outStr)
throws IOException
{
long total = 0;
byte[] bs = new byte[BUFFER_SIZE];
int numRead;
while ((numRead = inStr.read(bs, 0, bs.length)) >= 0)
{
total += numRead;
if (total > limit)
{
throw new StreamOverflowException("Data Overflow");
}
outStr.write(bs, 0, numRead);
}
return total;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy