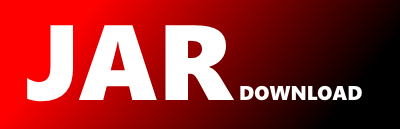
com.braintree.org.bouncycastle.asn1.DERT61String Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of encryption Show documentation
Show all versions of encryption Show documentation
Client side encryption for Braintree integrations on Android.
The newest version!
package com.braintree.org.bouncycastle.asn1;
import java.io.IOException;
/**
* DER T61String (also the teletex string)
*/
public class DERT61String
extends ASN1Object
implements DERString
{
String string;
/**
* return a T61 string from the passed in object.
*
* @exception IllegalArgumentException if the object cannot be converted.
*/
public static DERT61String getInstance(
Object obj)
{
if (obj == null || obj instanceof DERT61String)
{
return (DERT61String)obj;
}
throw new IllegalArgumentException("illegal object in getInstance: " + obj.getClass().getName());
}
/**
* return an T61 String from a tagged object.
*
* @param obj the tagged object holding the object we want
* @param explicit true if the object is meant to be explicitly
* tagged false otherwise.
* @exception IllegalArgumentException if the tagged object cannot
* be converted.
*/
public static DERT61String getInstance(
ASN1TaggedObject obj,
boolean explicit)
{
DERObject o = obj.getObject();
if (explicit)
{
return getInstance(o);
}
else
{
return new DERT61String(ASN1OctetString.getInstance(o).getOctets());
}
}
/**
* basic constructor - with bytes.
*/
public DERT61String(
byte[] string)
{
char[] cs = new char[string.length];
for (int i = 0; i != cs.length; i++)
{
cs[i] = (char)(string[i] & 0xff);
}
this.string = new String(cs);
}
/**
* basic constructor - with string.
*/
public DERT61String(
String string)
{
this.string = string;
}
public String getString()
{
return string;
}
public String toString()
{
return string;
}
void encode(
DEROutputStream out)
throws IOException
{
out.writeEncoded(T61_STRING, this.getOctets());
}
public byte[] getOctets()
{
char[] cs = string.toCharArray();
byte[] bs = new byte[cs.length];
for (int i = 0; i != cs.length; i++)
{
bs[i] = (byte)cs[i];
}
return bs;
}
boolean asn1Equals(
DERObject o)
{
if (!(o instanceof DERT61String))
{
return false;
}
return this.getString().equals(((DERT61String)o).getString());
}
public int hashCode()
{
return this.getString().hashCode();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy