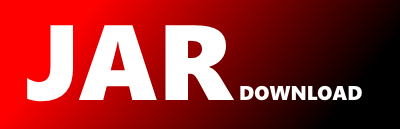
com.brambolt.lang.reflect.Reflection Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of brambolt-rt Show documentation
Show all versions of brambolt-rt Show documentation
Small utilities and convenience wrappers.
package com.brambolt.lang.reflect;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
/**
* Porcelain for single line reflection without declared exceptions.
*/
public class Reflection {
/**
* Instantiates an object of the parameter class with the default constructor.
*
* @param The type of the object to instantiate
* @param className The class to instantiate
* @param description The description to prefix exception messages with
* @return The instantiated object
* @throws IllegalStateException In case instantiation fails
*/
public static T instantiate(String className, String description) {
return instantiate(className, new Class>[] {}, new Object[] {}, description);
}
/**
* Instantiates an object of the parameter class with the constructor
* identified by the parameter types, using the parameter objects.
*
* @param The type of the object to instantiate
* @param className The class to instantiate
* @param parameterTypes The parameter types of the constructor to use
* @param parameters The parameter objects to pass
* @param description The description to prefix exception messages with
* @return The instantiated object
* @throws IllegalStateException In case instantiation fails
*/
public static T instantiate(
String className, Class>[] parameterTypes, Object[] parameters, String description) {
try {
@SuppressWarnings("unchecked")
Class clss = (Class) Class.forName(className);
Constructor constructor = clss.getConstructor(parameterTypes);
return constructor.newInstance(parameters);
} catch (ClassNotFoundException x) {
throw new IllegalStateException(
description + " class [" + className + "] not available", x);
} catch (NoSuchMethodException x) {
throw new IllegalStateException("" +
description + " constructor [" + className + "] not available", x);
} catch (IllegalAccessException | InstantiationException | InvocationTargetException x) {
throw new IllegalStateException(
description + " [" + className + "] not instantiated", x);
}
}
/**
* Invokes a static method on a class as identified by the parameters.
*
* @param The return type for the method to invoke
* @param className The class to invoke
* @param methodName The static method to invoke
* @param parameterTypes The parameter types of the method
* @param parameters The parameter objects to pass to the method
* @param description The description to prefix exception messages with
* @return The return value
* @throws IllegalArgumentException If one or more parameters are missing
* @throws IllegalStateException In case invocation fails
*/
public static T invoke(
String className, String methodName,
Class>[] parameterTypes, Object[] parameters,
String description) {
return invoke(className, methodName, null, parameterTypes, parameters, description);
}
/**
* Invokes a method on an object as identified by the parameters.
*
* @param The return type for the method to invoke
* @param className The class of the object to invoke
* @param methodName The method to invoke
* @param object The object to dispatch the method on
* @param parameterTypes The parameter types of the constructor to use
* @param parameters The parameter objects to pass
* @param description The description to prefix exception messages with
* @return The instantiated object
* @throws IllegalArgumentException If one or more parameters are missing
* @throws IllegalStateException In case instantiation fails
*/
public static T invoke(
String className, String methodName, Object object,
Class>[] parameterTypes, Object[] parameters,
String description) {
if (null == className || className.trim().isEmpty())
throw new IllegalArgumentException("No class name provided");
if (null == methodName || methodName.trim().isEmpty())
throw new IllegalArgumentException("No method name provided");
try {
Class> clss = Class.forName(className);
Method method = clss.getDeclaredMethod(methodName, parameterTypes);
//noinspection unchecked
return (T) method.invoke(object, parameters);
} catch (ClassNotFoundException x) {
throw new IllegalStateException(
description + " class [" + className + "] not available", x);
} catch (NoSuchMethodException x) {
throw new IllegalStateException("" +
description + " constructor [" + className + "] not available", x);
} catch (IllegalAccessException | InvocationTargetException x) {
throw new IllegalStateException(
description + " [" + className + "] not instantiated", x);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy