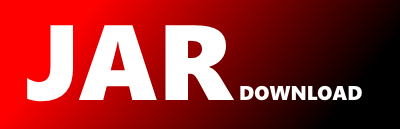
com.brightsparklabs.asanti.data.AsnData Maven / Gradle / Ivy
/*
* Maintained by brightSPARK Labs.
* www.brightsparklabs.com
*
* Refer to LICENSE at repository root for license details.
*/
package com.brightsparklabs.asanti.data;
import com.brightsparklabs.asanti.exception.DecodeException;
import com.brightsparklabs.asanti.schema.AsnPrimitiveType;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import java.util.Optional;
import java.util.regex.Pattern;
/**
* Interface for modeling ASN.1 data which has been mapped against a schema
*
* @author brightSPARK Labs
*/
public interface AsnData {
// -------------------------------------------------------------------------
// PUBLIC METHODS
// -------------------------------------------------------------------------
/**
* Returns the {@link AsnPrimitiveType} of the specified tag
*
* @param tag tag to retrieve the type of
* @return the {@link AsnPrimitiveType} of the specified tag or or {@code Optional.absent()} if
* the tag does not exist in this data.
*/
Optional getPrimitiveType(String tag);
/**
* Returns all tags found in the ASN data as a set of XPath like strings. E.g.
* "/Document/header/published/date", "/Document/header/lastModified/date",
* "/Document/body/prefix/text"
*
* @return all tags in the data
*/
ImmutableSet getTags();
/**
* Returns all tags which match the supplied regular expression
*
* @param regex regular expression to match tags against
* @return all tags which match the supplied regular expression
*/
ImmutableSet getTagsMatching(Pattern regex);
/**
* Returns the tags from the data which could not be mapped using the schema. E.g.
* "/Document/body/content/99", "/Document/0[99]/0[1]/0[1]"
*
* @return all unmapped tags in the data
*/
ImmutableSet getUnmappedTags();
/**
* Determines whether the specified tag is contained in the data
*
* @param tag tag to check
* @return {@code true} if the tag is in the data; {@code false} otherwise
*/
boolean contains(String tag);
/**
* Determines whether the data contains any tags matching the supplied regular expression
*
* @param regex regular expression to match tags against
* @return {@code true} if the tag is in the data; {@code false} otherwise
*/
boolean contains(Pattern regex);
/**
* Gets the data (bytes) associated with the specified tag.
*
* Note that because this method is returning unprocessed bytes it will provide results for
* partially matched tags as well as "raw" tags
*
* @param tag tag associated with the data
* @return data associated with the specified tag or {@code Optional.absent()} if the tag does
* not exist
*/
Optional getBytes(String tag);
/**
* Gets the data (bytes) from all tags matching the supplied regular expression.
*
* @param regex regular expression to match tags against
* @return data associated with the matching tags. Map is of form: {@code tag => data}
*/
ImmutableMap getBytesMatching(Pattern regex);
/**
* Gets the data (bytes) associated with the specified tag as a hex string.
*
* Note that because this method is returning unprocessed bytes it will provide results for
* partially matched tags as well as "raw" tags The sting will not be prepended with
* "0x", as this function is implicitly returning a hex string, using the uppercase alphabet, eg
* "0A12DF".
*
* @param tag tag associated with the data
* @return data associated with the specified tag (e.g. {@code "010203"}) or {@code
* Optional.absent()} if the tag does not exist
*/
Optional getHexString(String tag);
/**
* Gets the data (bytes) from all tags matching the supplied regular expression as hex strings.
*
* @param regex regular expression to match tags against
* @return data associated with the matching tags. Map is of form: {@code tag => data}
*/
ImmutableMap getHexStringsMatching(Pattern regex);
/**
* Gets the data (bytes) associated with the specified tag as a printable string.
*
* Note that because this method needs to process the bytes in a way that requires knowing
* the tags type it will only return results for fully decoded tags
*
* @param tag tag associated with the data
* @return data associated with the specified tag or {@code Optional.absent()} if the tag does
* not exist
* @throws DecodeException if any errors occur decoding the data associated with the tag
*/
Optional getPrintableString(String tag) throws DecodeException;
/**
* Gets the data (bytes) from all tags matching the supplied regular expression as printable
* strings.
*
* Note that because this method needs to process the bytes in a way that requires knowing
* the tags type it will only return results for fully decoded tags
*
* @param regex regular expression to match tags against
* @return data associated with the matching tags. Map is of form: {@code tag => data}
* @throws DecodeException if any errors occur decoding the data associated with the tags
*/
ImmutableMap getPrintableStringsMatching(Pattern regex) throws DecodeException;
/**
* Gets the data associated with the specified tag.
*
* Note that because this method needs to process the bytes in a way that requires knowing
* the tags' type it will only return results for fully decoded tags
*
* @param tag tag associated with the data
* @param classOfT the class of data that is expected for this tag
* @param the type of data that will be returned in the {@link Optional}
* @return data associated with the specified tag or {@code Optional.absent()} if the tag does
* not exist
* The expected Java object type for each ASN.1 schema type is:
*
* - BitString: String
*
- BmpString: String
*
- Boolean: boolean
*
- CharacterString: String
*
- Date: Timestamp
*
- DateTime: Timestamp
*
- Duration: String
*
- EmbeddedPDV: String
*
- Enumerated: String
*
- External: String
*
- GeneralizedTime: Timestamp
*
- GeneralString: String
*
- GraphicString: String
*
- Ia5String: String
*
- InstanceOf: String
*
- Integer: BigInteger
*
- Iri: String
*
- Iso646String: String
*
- Null: String
*
- NumericString: String
*
- ObjectClassField: String
*
- OctetString: byte[]
*
- Oid: String
*
- OidIri: String
*
- Prefixed: String
*
- PrintableString: String
*
- Real: String
*
- RelativeIri: String
*
- RelativeOid: String
*
- RelativeOidIri: String
*
- TeletexString: String
*
- Time: Timestamp
*
- TimeOfDay: Timestamp
*
- UniversalString: String
*
- Utf8String: String
*
- VideotexString: String
*
- VisibleString: String
*
*
* @throws DecodeException if any errors occur decoding the data associated with the tag
* @throws ClassCastException if the type of the tag does not match T
*/
Optional getDecodedObject(String tag, Class classOfT)
throws DecodeException, ClassCastException;
/**
* Gets the data from all tags matching the supplied regular expression as the decoded Java
* object most appropriate to its type.
*
* Note that because this method needs to process the bytes in a way that requires knowing
* the tags type it will only return results for fully decoded tags
*
* @param regex regular expression to match tags against
* @return data associated with the matching tags. Map is of form: {@code tag => data}
* @throws DecodeException if any errors occur decoding the data associated with the tags
*/
ImmutableMap getDecodedObjectsMatching(Pattern regex) throws DecodeException;
}