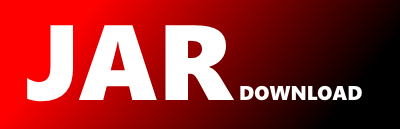
com.bronto.api.request.BrontoReadPager Maven / Gradle / Ivy
The newest version!
package com.bronto.api.request;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import com.bronto.api.BrontoApi;
import com.bronto.api.BrontoClientException;
public class BrontoReadPager implements Iterator {
private Iterator objects;
private BrontoApi client;
private BrontoReadRequest read;
public BrontoReadPager(BrontoApi client, BrontoReadRequest read) {
this.client = client;
this.read = read.reset();
this.objects = this.getCurrentObjects();
}
public int getCurrentPage() {
return read.getCurrentPage();
}
private Iterator getCurrentObjects() {
try {
return ((List) client.invoke(read)).iterator();
} catch (BrontoClientException brontoException) {
/*
* Error code 116 is an end of result error. Since all the data's
* been read, return an empty list. All other exceptions should be
* handled by the existing exception-handling logic.
*/
if (brontoException.getCode() == 116) {
return Collections.emptyListIterator();
} else {
throw brontoException;
}
}
}
@Override
public boolean hasNext() {
if (objects.hasNext()) {
return true;
} else {
read.next();
objects = getCurrentObjects();
return objects.hasNext();
}
}
@Override
public T next() {
return objects.next();
}
@Override
public void remove() {
objects.remove();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy