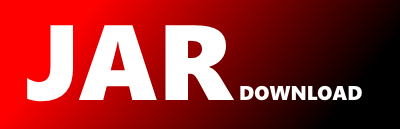
com.buabook.kdb.data.KdbDict Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-kdb-communication Show documentation
Show all versions of java-kdb-communication Show documentation
Java library to publish data into and query data out of kdb+ processes (c) 2017 Sport Trades Ltd
The newest version!
package com.buabook.kdb.data;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Objects;
import java.util.stream.Collectors;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.buabook.kdb.Converters;
import com.buabook.kdb.Types;
import com.buabook.kdb.query.KdbQuery;
import com.buabook.kdb.exceptions.DataOverwriteNotPermittedException;
import com.buabook.kdb.exceptions.DictUnionNotPermittedException;
import com.google.common.collect.Lists;
import com.kx.c.Dict;
/**
* KDB Dictionary Container
* Provides the ability to represent (and generate) kdb dictionaries in a more Java
* friendly way. Objects are not thread-safe.
* (c) 2014 - 2017 Sport Trades Ltd
*
* @author Jas Rajasansir
* @version 1.1.0
* @since 8 Jun 2014
*/
public class KdbDict {
private static final Logger log = LoggerFactory.getLogger(KdbDict.class);
/** The dictionary as stored within Java */
private final Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy