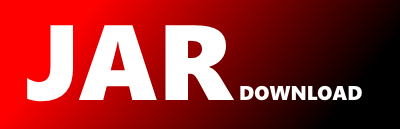
com.buabook.kdb.data.KdbTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-kdb-communication Show documentation
Show all versions of java-kdb-communication Show documentation
Java library to publish data into and query data out of kdb+ processes (c) 2017 Sport Trades Ltd
The newest version!
package com.buabook.kdb.data;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.TreeSet;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.buabook.kdb.Converters;
import com.buabook.kdb.exceptions.DataOverwriteNotPermittedException;
import com.buabook.kdb.exceptions.TableColumnAlreadyExistsException;
import com.buabook.kdb.exceptions.TableSchemaMismatchException;
import com.buabook.kdb.query.KdbQuery;
import com.google.common.base.Strings;
import com.kx.c;
import com.kx.c.Dict;
import com.kx.c.Flip;
/**
* Table Data Container for kdb
* Data container representing a table that can be serialised and sent to a kdb process (via {@link c})
* Also provides the ability to accept a {@link Flip} object received from a kdb process and access it
* within Java, including iterating over it with the {@link KdbDict} object.
* Implementation is not thread-safe.
* (c) 2014 - 2017 Sport Trades Ltd
*
* @see KdbTableIterator
* @see KdbDict
*
* @author Jas Rajasansir
* @version 1.1.0
* @since 1 Apr 2014
*/
public class KdbTable implements Iterable {
private static final Logger log = LoggerFactory.getLogger(KdbTable.class);
/** The table name for the current instance */
private String tableName;
/** The container of the kdb data stored column-wise */
private final Map> data;
private int rowCount;
/**
* Instantiate an empty table structure
* @param tableName The name of the table to create
* @throws IllegalArgumentException If the table name is empty or null
*/
public KdbTable(String tableName) throws IllegalArgumentException {
if(Strings.isNullOrEmpty(tableName))
throw new IllegalArgumentException();
this.tableName = tableName;
this.data = new HashMap<>();
this.rowCount = 0;
}
/**
* Instantiates a new kdb table object with a set of initial data in kdb format
* @param tableName The name of the table to create
* @param initialData The initial data set to populate the new object with
* @throws IllegalArgumentException If the table name is empty or null
* @see #setInitialDataSet(Flip)
*/
public KdbTable(String tableName, Flip initialData) throws DataOverwriteNotPermittedException {
this(tableName);
setInitialDataSet(initialData);
}
/**
* Performs the initial set of data from a kdb {@link Flip} object
* @param initialData The dataset to use to set the object
* @throws DataOverwriteNotPermittedException If there is already data in the current data structure
* @see #doSetOfInitialDataSet(Flip, boolean)
*/
public void setInitialDataSet(Flip initialData) throws DataOverwriteNotPermittedException {
doSetOfInitialDataSet(initialData, false);
}
/**
* Performs the initial (or subsequent) set of data from a kdb {@link Flip} object
* @param initialData The dataset to use to set the object
* @see #doSetOfInitialDataSet(Flip, boolean)
*/
public void forceSetInitialDataSet(Flip initialData) {
doSetOfInitialDataSet(initialData, true);
}
private void doSetOfInitialDataSet(Flip initialData, boolean overwrite) throws DataOverwriteNotPermittedException {
if(! isEmpty()) {
if(! overwrite) {
log.error("Data already exists and overwrite not set [ Table: {} ]", tableName);
throw new DataOverwriteNotPermittedException();
}
}
data.clear();
for(int cCount = 0; cCount < initialData.x.length; cCount++) {
String colName = initialData.x[cCount];
Object[] colData = Converters.arrayToObjectArray(initialData.y[cCount]);
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy