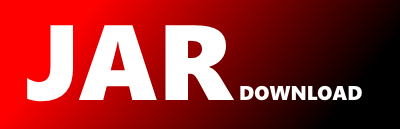
com.buession.httpclient.HttpClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of buession-httpclient Show documentation
Show all versions of buession-httpclient Show documentation
Buession Framework Http Client
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one or more contributor license agreements.
* See the NOTICE file distributed with this work for additional information regarding copyright ownership.
* The ASF licenses this file to you under the Apache License, Version 2.0 (the "License"); you may not
* use this file except in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is
* distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and limitations under the License.
*
* =========================================================================================================
*
* This software consists of voluntary contributions made by many individuals on behalf of the
* Apache Software Foundation. For more information on the Apache Software Foundation, please see
* .
*
* +-------------------------------------------------------------------------------------------------------+
* | License: http://www.apache.org/licenses/LICENSE-2.0.txt |
* | Author: Yong.Teng |
* | Copyright @ 2013-2022 Buession.com Inc. |
* +-------------------------------------------------------------------------------------------------------+
*/
package com.buession.httpclient;
import com.buession.httpclient.core.Header;
import com.buession.httpclient.core.RequestBody;
import com.buession.httpclient.core.RequestMethod;
import com.buession.httpclient.core.Response;
import com.buession.httpclient.exception.RequestException;
import java.io.IOException;
import java.net.URI;
import java.net.URL;
import java.util.List;
import java.util.Map;
/**
* Http 客户端
*
* @author Yong.Teng
*/
public interface HttpClient extends IBaseHttpClient {
/**
* GET 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response get(String url) throws IOException, RequestException{
return get(URI.create(url));
}
/**
* GET 请求
*
* @param uri
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URI uri) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response get(URL url) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response get(String url, Map parameters) throws IOException, RequestException{
return get(URI.create(url), parameters);
}
/**
* GET 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URI uri, Map parameters) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response get(URL url, Map parameters) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response get(String url, List headers) throws IOException, RequestException{
return get(URI.create(url), headers);
}
/**
* GET 请求
*
* @param uri
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URI uri, List headers) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response get(URL url, List headers) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response get(String url, Map parameters, List headers)
throws IOException, RequestException{
return get(URI.create(url), parameters, headers);
}
/**
* GET 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URI uri, Map parameters, List headers) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response get(URL url, Map parameters, List headers) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response get(String url, int readTimeout) throws IOException, RequestException{
return get(URI.create(url), readTimeout);
}
/**
* GET 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URI uri, int readTimeout) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URL url, int readTimeout) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response get(String url, int readTimeout, Map parameters)
throws IOException, RequestException{
return get(URI.create(url), readTimeout, parameters);
}
/**
* GET 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URI uri, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URL url, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response get(String url, int readTimeout, List headers) throws IOException, RequestException{
return get(URI.create(url), readTimeout, headers);
}
/**
* GET 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URI uri, int readTimeout, List headers) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URL url, int readTimeout, List headers) throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response get(String url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException{
return get(URI.create(url), readTimeout, parameters, headers);
}
/**
* GET 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URI uri, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* GET 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response get(URL url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response post(String url) throws IOException, RequestException{
return post(URI.create(url));
}
/**
* POST 请求
*
* @param uri
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response post(URL url) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response post(String url, Map parameters) throws IOException, RequestException{
return post(URI.create(url), parameters);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, Map parameters) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response post(URL url, Map parameters) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response post(String url, List headers) throws IOException, RequestException{
return post(URI.create(url), headers);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, List headers) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response post(URL url, List headers) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response post(String url, Map parameters, List headers)
throws IOException, RequestException{
return post(URI.create(url), parameters, headers);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, Map parameters, List headers) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response post(URL url, Map parameters, List headers) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response post(String url, RequestBody> data) throws IOException, RequestException{
return post(URI.create(url), data);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, RequestBody> data) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response post(URL url, RequestBody> data) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response post(String url, RequestBody> data, Map parameters)
throws IOException, RequestException{
return post(URI.create(url), data, parameters);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, RequestBody> data, Map parameters) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response post(URL url, RequestBody> data, Map parameters) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response post(String url, RequestBody> data, List headers) throws IOException, RequestException{
return post(URI.create(url), data, headers);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, RequestBody> data, List headers) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response post(URL url, RequestBody> data, List headers) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response post(String url, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException{
return post(URI.create(url), data, parameters, headers);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response post(URL url, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response post(String url, int readTimeout) throws IOException, RequestException{
return post(URI.create(url), readTimeout);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, int readTimeout) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URL url, int readTimeout) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response post(String url, int readTimeout, Map parameters)
throws IOException, RequestException{
return post(URI.create(url), readTimeout, parameters);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URL url, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response post(String url, int readTimeout, List headers) throws IOException, RequestException{
return post(URI.create(url), readTimeout, headers);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, int readTimeout, List headers) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URL url, int readTimeout, List headers) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response post(String url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException{
return post(URI.create(url), readTimeout, parameters, headers);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URL url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response post(String url, int readTimeout, RequestBody> data) throws IOException, RequestException{
return post(URI.create(url), readTimeout, data);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, int readTimeout, RequestBody> data) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URL url, int readTimeout, RequestBody> data) throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response post(String url, int readTimeout, RequestBody> data, Map parameters)
throws IOException, RequestException{
return post(URI.create(url), readTimeout, data, parameters);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, int readTimeout, RequestBody> data, Map parameters)
throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URL url, int readTimeout, RequestBody> data, Map parameters)
throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response post(String url, int readTimeout, RequestBody> data, List headers)
throws IOException, RequestException{
return post(URI.create(url), readTimeout, data, headers);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, int readTimeout, RequestBody> data, List headers)
throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URL url, int readTimeout, RequestBody> data, List headers)
throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response post(String url, int readTimeout, RequestBody> data, Map parameters,
List headers) throws IOException, RequestException{
return post(URI.create(url), readTimeout, data, parameters, headers);
}
/**
* POST 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URI uri, int readTimeout, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException;
/**
* POST 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response post(URL url, int readTimeout, RequestBody> data, Map parameters,
List headers) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response put(String url) throws IOException, RequestException{
return put(URI.create(url));
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response put(URL url) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response put(String url, Map parameters) throws IOException, RequestException{
return put(URI.create(url), parameters);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, Map parameters) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response put(URL url, Map parameters) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response put(String url, List headers) throws IOException, RequestException{
return put(URI.create(url), headers);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, List headers) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response put(URL url, List headers) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response put(String url, Map parameters, List headers)
throws IOException, RequestException{
return put(URI.create(url), parameters, headers);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, Map parameters, List headers) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response put(URL url, Map parameters, List headers) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response put(String url, RequestBody> data) throws IOException, RequestException{
return put(URI.create(url), data);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, RequestBody> data) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response put(URL url, RequestBody> data) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response put(String url, RequestBody> data, Map parameters)
throws IOException, RequestException{
return put(URI.create(url), data, parameters);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, RequestBody> data, Map parameters) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response put(URL url, RequestBody> data, Map parameters) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response put(String url, RequestBody> data, List headers) throws IOException, RequestException{
return put(URI.create(url), data, headers);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, RequestBody> data, List headers) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response put(URL url, RequestBody> data, List headers) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response put(String url, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException{
return put(URI.create(url), data, parameters, headers);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response put(URL url, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response put(String url, int readTimeout) throws IOException, RequestException{
return put(URI.create(url), readTimeout);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, int readTimeout) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URL url, int readTimeout) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response put(String url, int readTimeout, Map parameters)
throws IOException, RequestException{
return put(URI.create(url), readTimeout, parameters);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URL url, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response put(String url, int readTimeout, List headers) throws IOException, RequestException{
return put(URI.create(url), readTimeout, headers);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, int readTimeout, List headers) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URL url, int readTimeout, List headers) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response put(String url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException{
return put(URI.create(url), readTimeout, parameters, headers);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URL url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response put(String url, int readTimeout, RequestBody> data) throws IOException, RequestException{
return put(URI.create(url), readTimeout, data);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, int readTimeout, RequestBody> data) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URL url, int readTimeout, RequestBody> data) throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response put(String url, int readTimeout, RequestBody> data, Map parameters)
throws IOException, RequestException{
return put(URI.create(url), readTimeout, data, parameters);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, int readTimeout, RequestBody> data, Map parameters)
throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URL url, int readTimeout, RequestBody> data, Map parameters)
throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response put(String url, int readTimeout, RequestBody> data, List headers)
throws IOException, RequestException{
return put(URI.create(url), readTimeout, data, headers);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, int readTimeout, RequestBody> data, List headers)
throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URL url, int readTimeout, RequestBody> data, List headers) throws IOException,
RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response put(String url, int readTimeout, RequestBody> data, Map parameters,
List headers) throws IOException, RequestException{
return put(URI.create(url), readTimeout, data, parameters, headers);
}
/**
* PUT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URI uri, int readTimeout, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException;
/**
* PUT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response put(URL url, int readTimeout, RequestBody> data, Map parameters,
List headers) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response patch(String url) throws IOException, RequestException{
return patch(URI.create(url));
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response patch(URL url) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response patch(String url, Map parameters) throws IOException, RequestException{
return patch(URI.create(url), parameters);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, Map parameters) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response patch(URL url, Map parameters) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response patch(String url, List headers) throws IOException, RequestException{
return patch(URI.create(url), headers);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, List headers) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response patch(URL url, List headers) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response patch(String url, Map parameters, List headers)
throws IOException, RequestException{
return patch(URI.create(url), parameters, headers);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, Map parameters, List headers) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response patch(URL url, Map parameters, List headers) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response patch(String url, RequestBody> data) throws IOException, RequestException{
return patch(URI.create(url), data);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, RequestBody> data) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response patch(URL url, RequestBody> data) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response patch(String url, RequestBody> data, Map parameters)
throws IOException, RequestException{
return patch(URI.create(url), data, parameters);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, RequestBody> data, Map parameters) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response patch(URL url, RequestBody> data, Map parameters) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response patch(String url, RequestBody> data, List headers) throws IOException, RequestException{
return patch(URI.create(url), data, headers);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, RequestBody> data, List headers) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response patch(URL url, RequestBody> data, List headers) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response patch(String url, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException{
return patch(URI.create(url), data, parameters, headers);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response patch(URL url, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response patch(String url, int readTimeout) throws IOException, RequestException{
return patch(URI.create(url), readTimeout);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, int readTimeout) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URL url, int readTimeout) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response patch(String url, int readTimeout, Map parameters)
throws IOException, RequestException{
return patch(URI.create(url), readTimeout, parameters);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URL url, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response patch(String url, int readTimeout, List headers) throws IOException, RequestException{
return patch(URI.create(url), readTimeout, headers);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, int readTimeout, List headers) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URL url, int readTimeout, List headers) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response patch(String url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException{
return patch(URI.create(url), readTimeout, parameters, headers);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URL url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response patch(String url, int readTimeout, RequestBody> data) throws IOException, RequestException{
return patch(URI.create(url), readTimeout, data);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, int readTimeout, RequestBody> data) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URL url, int readTimeout, RequestBody> data) throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response patch(String url, int readTimeout, RequestBody> data, Map parameters)
throws IOException, RequestException{
return patch(URI.create(url), readTimeout, data, parameters);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, int readTimeout, RequestBody> data, Map parameters)
throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URL url, int readTimeout, RequestBody> data, Map parameters)
throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response patch(String url, int readTimeout, RequestBody> data, List headers)
throws IOException, RequestException{
return patch(URI.create(url), readTimeout, data, headers);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, int readTimeout, RequestBody> data, List headers)
throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URL url, int readTimeout, RequestBody> data, List headers)
throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response patch(String url, int readTimeout, RequestBody> data, Map parameters,
List headers) throws IOException, RequestException{
return patch(URI.create(url), readTimeout, data, parameters, headers);
}
/**
* PATCH 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URI uri, int readTimeout, RequestBody> data, Map parameters, List headers)
throws IOException, RequestException;
/**
* PATCH 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param data
* 请求数据
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response patch(URL url, int readTimeout, RequestBody> data, Map parameters,
List headers) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response delete(String url) throws IOException, RequestException{
return delete(URI.create(url));
}
/**
* DELETE 请求
*
* @param uri
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URI uri) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response delete(URL url) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response delete(String url, Map parameters) throws IOException, RequestException{
return delete(URI.create(url), parameters);
}
/**
* DELETE 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URI uri, Map parameters) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response delete(URL url, Map parameters) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response delete(String url, List headers) throws IOException, RequestException{
return delete(URI.create(url), headers);
}
/**
* DELETE 请求
*
* @param uri
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URI uri, List headers) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response delete(URL url, List headers) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response delete(String url, Map parameters, List headers)
throws IOException, RequestException{
return delete(URI.create(url), parameters, headers);
}
/**
* DELETE 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URI uri, Map parameters, List headers) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response delete(URL url, Map parameters, List headers) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response delete(String url, int readTimeout) throws IOException, RequestException{
return delete(URI.create(url), readTimeout);
}
/**
* DELETE 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URI uri, int readTimeout) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URL url, int readTimeout) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response delete(String url, int readTimeout, Map parameters)
throws IOException, RequestException{
return delete(URI.create(url), readTimeout, parameters);
}
/**
* DELETE 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URI uri, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URL url, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response delete(String url, int readTimeout, List headers) throws IOException, RequestException{
return delete(URI.create(url), readTimeout, headers);
}
/**
* DELETE 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URI uri, int readTimeout, List headers) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URL url, int readTimeout, List headers) throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response delete(String url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException{
return delete(URI.create(url), readTimeout, parameters, headers);
}
/**
* DELETE 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URI uri, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* DELETE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response delete(URL url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response connect(String url) throws IOException, RequestException{
return connect(URI.create(url));
}
/**
* CONNECT 请求
*
* @param uri
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URI uri) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response connect(URL url) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response connect(String url, Map parameters) throws IOException, RequestException{
return connect(URI.create(url), parameters);
}
/**
* CONNECT 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URI uri, Map parameters) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response connect(URL url, Map parameters) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response connect(String url, List headers) throws IOException, RequestException{
return connect(URI.create(url), headers);
}
/**
* CONNECT 请求
*
* @param uri
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URI uri, List headers) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response connect(URL url, List headers) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response connect(String url, Map parameters, List headers)
throws IOException, RequestException{
return connect(URI.create(url), parameters, headers);
}
/**
* CONNECT 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URI uri, Map parameters, List headers)
throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response connect(URL url, Map parameters, List headers)
throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response connect(String url, int readTimeout) throws IOException, RequestException{
return connect(URI.create(url), readTimeout);
}
/**
* CONNECT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URI uri, int readTimeout) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URL url, int readTimeout) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response connect(String url, int readTimeout, Map parameters)
throws IOException, RequestException{
return connect(URI.create(url), readTimeout, parameters);
}
/**
* CONNECT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URI uri, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URL url, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response connect(String url, int readTimeout, List headers) throws IOException, RequestException{
return connect(URI.create(url), readTimeout, headers);
}
/**
* CONNECT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URI uri, int readTimeout, List headers) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URL url, int readTimeout, List headers) throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response connect(String url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException{
return connect(URI.create(url), readTimeout, parameters, headers);
}
/**
* CONNECT 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URI uri, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* CONNECT 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response connect(URL url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response trace(String url) throws IOException, RequestException{
return trace(URI.create(url));
}
/**
* TRACE 请求
*
* @param uri
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URI uri) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response trace(URL url) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response trace(String url, Map parameters) throws IOException, RequestException{
return trace(URI.create(url), parameters);
}
/**
* TRACE 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URI uri, Map parameters) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response trace(URL url, Map parameters) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response trace(String url, List headers) throws IOException, RequestException{
return trace(URI.create(url), headers);
}
/**
* TRACE 请求
*
* @param uri
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URI uri, List headers) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response trace(URL url, List headers) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response trace(String url, Map parameters, List headers)
throws IOException, RequestException{
return trace(URI.create(url), parameters, headers);
}
/**
* TRACE 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URI uri, Map parameters, List headers) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response trace(URL url, Map parameters, List headers) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response trace(String url, int readTimeout) throws IOException, RequestException{
return trace(URI.create(url), readTimeout);
}
/**
* TRACE 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URI uri, int readTimeout) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URL url, int readTimeout) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response trace(String url, int readTimeout, Map parameters)
throws IOException, RequestException{
return trace(URI.create(url), readTimeout, parameters);
}
/**
* TRACE 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URI uri, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URL url, int readTimeout, Map parameters) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response trace(String url, int readTimeout, List headers) throws IOException, RequestException{
return trace(URI.create(url), readTimeout, headers);
}
/**
* TRACE 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URI uri, int readTimeout, List headers) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URL url, int readTimeout, List headers) throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
default Response trace(String url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException{
return trace(URI.create(url), readTimeout, parameters, headers);
}
/**
* TRACE 请求
*
* @param uri
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URI uri, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* TRACE 请求
*
* @param url
* 请求 URL
* @param readTimeout
* 读取超时时间
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response trace(URL url, int readTimeout, Map parameters, List headers)
throws IOException, RequestException;
/**
* COPY 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response copy(String url) throws IOException, RequestException{
return copy(URI.create(url));
}
/**
* COPY 请求
*
* @param uri
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response copy(URI uri) throws IOException, RequestException;
/**
* COPY 请求
*
* @param url
* 请求 URL
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response copy(URL url) throws IOException, RequestException;
/**
* COPY 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response copy(String url, Map parameters) throws IOException, RequestException{
return copy(URI.create(url), parameters);
}
/**
* COPY 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response copy(URI uri, Map parameters) throws IOException, RequestException;
/**
* COPY 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response copy(URL url, Map parameters) throws IOException, RequestException;
/**
* COPY 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response copy(String url, List headers) throws IOException, RequestException{
return copy(URI.create(url), headers);
}
/**
* COPY 请求
*
* @param uri
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response copy(URI uri, List headers) throws IOException, RequestException;
/**
* COPY 请求
*
* @param url
* 请求 URL
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
Response copy(URL url, List headers) throws IOException, RequestException;
/**
* COPY 请求
*
* @param url
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
*/
default Response copy(String url, Map parameters, List headers)
throws IOException, RequestException{
return copy(URI.create(url), parameters, headers);
}
/**
* COPY 请求
*
* @param uri
* 请求 URL
* @param parameters
* 请求参数
* @param headers
* 请求头
*
* @return Response {@link Response}
*
* @throws IOException
* IO 异常
* @throws RequestException
* 请求异常
* @since 2.3.0
*/
Response copy(URI uri, Map parameters, List