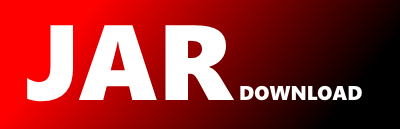
com.bugsnag.android.gradle.BugsnagPluginExtension.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of bugsnag-android-gradle-plugin Show documentation
Show all versions of bugsnag-android-gradle-plugin Show documentation
Gradle plugin to automatically upload ProGuard mapping files to Bugsnag.
package com.bugsnag.android.gradle
import com.bugsnag.android.gradle.internal.listProperty
import com.bugsnag.android.gradle.internal.mapProperty
import com.bugsnag.android.gradle.internal.newInstance
import com.bugsnag.android.gradle.internal.property
import groovy.lang.Closure
import org.gradle.api.Action
import org.gradle.api.model.ObjectFactory
import org.gradle.api.provider.ListProperty
import org.gradle.api.provider.MapProperty
import org.gradle.api.provider.Property
import org.gradle.util.ConfigureUtil
import java.io.File
import java.util.Locale
import javax.inject.Inject
// To make kotlin happy with gradle's nullability
private val NULL_STRING: String? = null
private val NULL_BOOLEAN: Boolean? = null
private val NULL_FILE: File? = null
internal const val UPLOAD_ENDPOINT_DEFAULT: String = "https://upload.bugsnag.com"
/**
* Defines configuration options (Gradle plugin extensions) for the BugsnagPlugin
*/
open class BugsnagPluginExtension @Inject constructor(objects: ObjectFactory) {
val sourceControl: SourceControl = objects.newInstance()
val enabled: Property = objects.property()
.convention(true)
val uploadJvmMappings: Property = objects.property()
.convention(true)
val uploadNdkMappings: Property = objects.property()
.convention(NULL_BOOLEAN)
val uploadNdkUnityLibraryMappings: Property = objects.property()
.convention(NULL_BOOLEAN)
val uploadReactNativeMappings: Property = objects.property()
.convention(NULL_BOOLEAN)
val reportBuilds: Property = objects.property()
.convention(true)
val uploadDebugBuildMappings: Property = objects.property()
.convention(false)
val endpoint: Property = objects.property()
.convention(UPLOAD_ENDPOINT_DEFAULT)
val releasesEndpoint = objects.property()
.convention("https://build.bugsnag.com")
val overwrite: Property = objects.property()
.convention(false)
val retryCount: Property = objects.property()
.convention(0)
val sharedObjectPaths: ListProperty = objects.listProperty()
.convention(emptyList())
val nodeModulesDir: Property = objects.property()
.convention(NULL_FILE)
val projectRoot: Property = objects.property().convention(NULL_STRING)
val failOnUploadError: Property = objects.property()
.convention(true)
val requestTimeoutMs: Property = objects.property()
.convention(60000)
// release API values
val builderName: Property = objects.property().convention(NULL_STRING)
val metadata: MapProperty = objects.mapProperty()
.convention(emptyMap())
val objdumpPaths: MapProperty = objects.mapProperty()
.convention(emptyMap())
val useLegacyNdkSymbolUpload: Property = objects.property()
.convention(false)
// exposes sourceControl as a nested object on the extension,
// see https://docs.gradle.org/current/userguide/custom_gradle_types.html#nested_objects
fun sourceControl(closure: Closure) {
ConfigureUtil.configure(closure, sourceControl)
}
fun sourceControl(action: Action) {
action.execute(sourceControl)
}
internal var filter: Action = Action {
if (it.name.toLowerCase(Locale.ENGLISH).contains("debug")) {
it.setEnabled(false)
}
}
fun variantFilter(action: Action) {
this.filter = action
}
}
interface VariantFilter {
val name: String
fun setEnabled(enabled: Boolean)
}
internal class VariantFilterImpl(override val name: String) : VariantFilter {
internal var variantEnabled: Boolean? = true
override fun setEnabled(enabled: Boolean) {
this.variantEnabled = enabled
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy