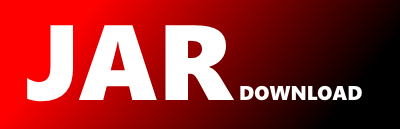
main.java.soot.Body Maven / Gradle / Ivy
/* Soot - a J*va Optimization Framework
* Copyright (C) 1997-1999 Raja Vallee-Rai
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
* Boston, MA 02111-1307, USA.
*/
/*
* Modified by the Sable Research Group and others 1997-1999.
* See the 'credits' file distributed with Soot for the complete list of
* contributors. (Soot is distributed at http://www.sable.mcgill.ca/soot)
*/
package soot;
import java.io.ByteArrayOutputStream;
import java.io.OutputStreamWriter;
import java.io.PrintWriter;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import soot.jimple.CaughtExceptionRef;
import soot.jimple.DefinitionStmt;
import soot.jimple.IdentityStmt;
import soot.jimple.InstanceInvokeExpr;
import soot.jimple.InvokeExpr;
import soot.jimple.InvokeStmt;
import soot.jimple.ParameterRef;
import soot.jimple.ThisRef;
import soot.options.Options;
import soot.tagkit.AbstractHost;
import soot.tagkit.Tag;
import soot.toolkits.exceptions.PedanticThrowAnalysis;
import soot.toolkits.graph.ExceptionalUnitGraph;
import soot.toolkits.graph.UnitGraph;
import soot.toolkits.scalar.FlowSet;
import soot.toolkits.scalar.InitAnalysis;
import soot.toolkits.scalar.LocalDefs;
import soot.toolkits.scalar.SimpleLiveLocals;
import soot.toolkits.scalar.SmartLocalDefs;
import soot.util.Chain;
import soot.util.EscapedWriter;
import soot.util.HashChain;
/**
* Abstract base class that models the body (code attribute) of a Java method.
* Classes that implement an Intermediate Representation for a method body should subclass it.
* In particular the classes GrimpBody, JimpleBody and BafBody all extend this
* class. This class provides methods that are common to any IR, such as methods
* to get the body's units (statements), traps, and locals.
*
* @see soot.grimp.GrimpBody
* @see soot.jimple.JimpleBody
* @see soot.baf.BafBody
*/
public abstract class Body extends AbstractHost implements Serializable
{
/** The method associated with this Body. */
protected transient SootMethod method = null;
/** The chain of locals for this Body. */
protected Chain localChain = new HashChain();
/** The chain of traps for this Body. */
protected Chain trapChain = new HashChain();
// RoboVM note: Added
protected List localVariables = new ArrayList<>();
/** The chain of units for this Body. */
protected PatchingChain unitChain = new PatchingChain(new HashChain());
/** Creates a deep copy of this Body. */
abstract public Object clone();
/** Creates a Body associated to the given method. Used by subclasses during initialization.
* Creation of a Body is triggered by e.g. Jimple.v().newBody(options).
*/
protected Body(SootMethod m)
{
this.method = m;
}
/** Creates an extremely empty Body. The Body is not associated to any method. */
protected Body()
{
}
/**
* Returns the method associated with this Body.
* @return the method that owns this body.
*/
public SootMethod getMethod()
{
if(method == null)
throw new RuntimeException("no method associated w/ body");
return method;
}
/**
* Sets the method associated with this Body.
* @param method the method that owns this body.
*
*/
public void setMethod(SootMethod method)
{
this.method = method;
}
/** Returns the number of locals declared in this body. */
public int getLocalCount()
{
return localChain.size();
}
/** Copies the contents of the given Body into this one. */
public Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy