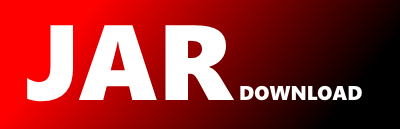
main.java.soot.options.phase_options_dialog.xsl Maven / Gradle / Ivy
/*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the
* Free Software Foundation, Inc., 59 Temple Place - Suite 330,
* Boston, MA 02111-1307, USA.
*
* This class is generated automajically from xml - DO NOT EDIT - as
* changes will be over written
*
* The purpose of this class is to automajically generate a options
* dialog in the event that options change
*
* Taking options away - should not damage the dialog
* Adding new sections of options - should not damage the dialog
* Adding new otpions to sections (of known option type) - should not
* damage the dialog
*
* Adding new option types will break the dialog (option type widgets
* will need to be created)
*
*/
package ca.mcgill.sable.soot.ui;
import org.eclipse.jface.dialogs.MessageDialog;
import org.eclipse.swt.widgets.*;
import org.eclipse.swt.*;
import org.eclipse.swt.layout.*;
import ca.mcgill.sable.soot.SootPlugin;
import java.util.*;
import org.eclipse.swt.events.*;
public class PhaseOptionsDialog extends AbstractOptionsDialog implements SelectionListener {
public PhaseOptionsDialog(Shell parentShell) {
super(parentShell);
}
/**
* each section gets initialize as a stack layer in pageContainer
* the area containing the options
*/
protected void initializePageContainer() {
addOtherPages(getPageContainer());
initializeRadioGroups();
initializeEnableGroups();
}
private void initializeRadioGroups(){
setRadioGroups(new HashMap());
int counter = 0;
ArrayList buttonList;
buttonList = new ArrayList();
if (isEnableButton(" ")) {
buttonList.add(get _widget());
get _widget().getButton().addSelectionListener(this);
}
getRadioGroups().put(new Integer(counter), buttonList);
counter++;
}
private void initializeEnableGroups(){
setEnableGroups(new ArrayList());
makeNewEnableGroup(" ");
addToEnableGroup(" ", get _widget(), " ");
get _widget().getButton().addSelectionListener(this);
makeNewEnableGroup(" ", " ");
addToEnableGroup(" ", " ", get _widget(), " ");
get _widget().getButton().addSelectionListener(this);
addToEnableGroup(" ", " ", get _widget(), " ");
updateAllEnableGroups();
}
public void widgetSelected(SelectionEvent e){
handleWidgetSelected(e);
}
public void widgetDefaultSelected(SelectionEvent e){
}
/**
* all options get saved as (alias, value) pair
*/
protected void okPressed() {
if(createNewConfig())
super.okPressed();
else {
Shell defaultShell = SootPlugin.getDefault().getWorkbench().getActiveWorkbenchWindow().getShell();
String projectName = getSootMainProjectWidget().getText().getText();
MessageDialog.openError(defaultShell, "Unable to find Soot Main Project", "Project "+projectName+" does not exist," +
" is no Java project or is closed.");
}
}
private boolean createNewConfig() {
setConfig(new HashMap());
boolean boolRes = false;
String stringRes = "";
boolean defBoolRes = false;
String defStringRes = "";
StringTokenizer listOptTokens;
String nextListToken;
setSootMainClass(getSootMainClassWidget().getText().getText());
return setSootMainProject(getSootMainProjectWidget().getText().getText());
}
protected HashMap savePressed() {
createNewConfig();
return getConfig();
}
/**
* the initial input of selection tree corresponds to each section
* at some point sections will have sub-sections which will be
* children of branches (ie phase - options)
*/
protected SootOption getInitialInput() {
SootOption root = new SootOption("", "");
SootOption parent;
SootOption subParent;
SootOption subSectParent;
SootOption _branch = new SootOption(" ", " ");
root.addChild( _branch);
parent = _branch;
SootOption _branch = new SootOption(" ", " ");
root.addChild( _branch);
parent = _branch;
//
//
SootOption _branch = new SootOption(" ", " ");
parent.addChild( _branch);
subParent = _branch;
SootOption _ _branch = new SootOption(" ", " ");
subParent.addChild( _ _branch);
subSectParent = _ _branch;
SootOption _ _branch = new SootOption(" ", " ");
subSectParent.addChild( _ _branch);
addOtherBranches(root);
return root;
}
}
Composite Child = Create(getPageContainer());
boolRes = get _widget().getButton().getSelection();
defBoolRes = ;
defBoolRes = false;
if (boolRes != defBoolRes) {
getConfig().put(get _widget().getAlias(), new Boolean(boolRes));
}
stringRes = get _widget().getText().getText();
defStringRes = " ";
defStringRes = "";
if ( (!(stringRes.equals(defStringRes))) && (stringRes != null) && (stringRes.length() != 0)) {
getConfig().put(get _widget().getAlias(), stringRes);
}
stringRes = get _widget().getSelectedAlias();
defStringRes = " ";
if (!stringRes.equals(defStringRes)) {
getConfig().put(get _widget().getAlias(), stringRes);
}
private Composite Create(Composite parent) {
String defKey;
String defaultString;
boolean defaultBool = false;
String defaultArray;
Group editGroup = new Group(parent, SWT.NONE);
GridLayout layout = new GridLayout();
editGroup .setLayout(layout);
editGroup .setText(" ");
editGroup .setData("id", " ");
String desc = " ";
if (desc .length() > 0) {
Label descLabel = new Label(editGroup , SWT.WRAP);
descLabel .setText(desc );
}
OptionData [] data;
defKey = " "+" "+" "+" "+" ";
defKey = defKey.trim();
if (isInDefList(defKey)) {
defaultBool = getBoolDef(defKey);
}
else {
defaultBool = ;
defaultBool = false;
}
set _widget(new BooleanOptionWidget(editGroup , SWT.NONE, new OptionData(" ", " ", " "," ", " ", defaultBool)));
data = new OptionData [] {
new OptionData(" ",
" ",
" ",
true),
false),
};
set _widget(new MultiOptionWidget(editGroup , SWT.NONE, data, new OptionData(" ", " ", " "," ", " ")));
defKey = " "+" "+" "+" "+" ";
defKey = defKey.trim();
if (isInDefList(defKey)) {
defaultString = getStringDef(defKey);
get _widget().setDef(defaultString);
}
defKey = " "+" "+" "+" "+" ";
defKey = defKey.trim();
if (isInDefList(defKey)) {
defaultString = getArrayDef(defKey);
}
else {
defaultString = " ";
defaultString = "";
}
set _widget(new ListOptionWidget(editGroup , SWT.NONE, new OptionData(" ", " ", " "," ", " ", defaultString)));
defKey = " "+" "+" "+" "+" ";
defKey = defKey.trim();
if (isInDefList(defKey)) {
defaultString = getStringDef(defKey);
}
else {
defaultString = " ";
defaultString = "";
}
set _widget(new StringOptionWidget(editGroup , SWT.NONE, new OptionData(" ", " ", " "," ", " ", defaultString)));
return editGroup ;
}
private BooleanOptionWidget _widget;
private void set _widget(BooleanOptionWidget widget) {
_widget = widget;
}
public BooleanOptionWidget get _widget() {
return _widget;
}
private ListOptionWidget _widget;
private void set _widget(ListOptionWidget widget) {
_widget = widget;
}
public ListOptionWidget get _widget() {
return _widget;
}
private StringOptionWidget _widget;
private void set _widget(StringOptionWidget widget) {
_widget = widget;
}
public StringOptionWidget get _widget() {
return _widget;
}
private MultiOptionWidget _widget;
private void set _widget(MultiOptionWidget widget) {
_widget = widget;
}
public MultiOptionWidget get _widget() {
return _widget;
}
\n
ARG
© 2015 - 2025 Weber Informatics LLC | Privacy Policy