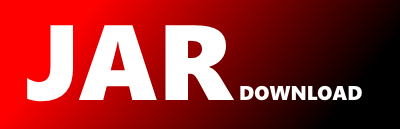
org.butor.dbauth.model.DefaultRoleModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of butor-auth-model Show documentation
Show all versions of butor-auth-model Show documentation
Butor Framework is a framework that enables fast and easy creation of HTTP services. (asynchronous and synchronous). It consists of several modules for different layers, such as data access, services, JSON serialization and test utilities.
It sits on top of popular and proven libraries such as Google Guava and Spring.
The project is released under APL 2.0
This project is an authentication module (Model).
package org.butor.dbauth.model;
import java.util.List;
import org.butor.auth.common.AuthMessageID;
import org.butor.auth.common.desc.Desc;
import org.butor.auth.common.desc.DescKey;
import org.butor.auth.common.func.FuncKey;
import org.butor.auth.common.role.Role;
import org.butor.auth.common.role.RoleItem;
import org.butor.auth.common.role.RoleItemKey;
import org.butor.auth.common.role.RoleKey;
import org.butor.auth.common.role.RoleServices;
import org.butor.auth.dao.AuthDao;
import org.butor.auth.dao.DescDao;
import org.butor.auth.dao.RoleDao;
import org.butor.dao.DAOMessageID;
import org.butor.json.CommonRequestArgs;
import org.butor.json.service.Context;
import org.butor.json.service.ResponseHandler;
import org.butor.json.service.ResponseHandlerHelper;
import org.butor.utils.AccessMode;
import org.butor.utils.ApplicationException;
import org.butor.utils.CommonMessageID;
import org.springframework.transaction.annotation.Transactional;
import com.google.common.base.Strings;
public class DefaultRoleModel implements RoleServices {
private RoleDao roleDao;
private DescDao descDao;
private String systemId;
private String secFunc;
private AuthDao authDao;
@Override
public void listRole(Context ctx, FuncKey criteria, String func) {
CommonRequestArgs cra = ctx.getRequest();
ResponseHandler rh = ctx.getResponseHandler();
List list = roleDao.listRole(criteria, func, cra);
ResponseHandlerHelper.addList(list, rh);
}
@Override
public void readRole(Context ctx, String roleId) {
CommonRequestArgs cra = ctx.getRequest();
if (!authDao.hasAccess(systemId, secFunc, AccessMode.READ, cra)) {
ApplicationException.exception(DAOMessageID.UNAUTHORIZED.getMessage());
}
ResponseHandler rh = ctx.getResponseHandler();
RoleKey rk = new RoleKey(roleId);
Desc desc = descDao.readDesc(rk, cra);
if (desc == null) {
rh.addMessage(CommonMessageID.NOT_FOUND.getMessage());
return;
}
Role role = new Role();
role.setId(roleId);
role.setDescription(desc.getDescription());
role.setRevNo(desc.getRevNo());
role.setStamp(desc.getStamp());
role.setUserId(role.getUserId());
role.setItems(roleDao.readRole(roleId, cra));
rh.addRow(role);
}
@Override
@Transactional
public void createRole(Context ctx, Role role) {
CommonRequestArgs cra = ctx.getRequest();
if (Strings.isNullOrEmpty(role.getId())) {
ApplicationException.exception(
CommonMessageID.MISSING_ARG.getMessage("roleId"));
}
if (Strings.isNullOrEmpty(role.getDescription())) {
ApplicationException.exception(
CommonMessageID.MISSING_ARG.getMessage("description"));
return;
}
List items = role.getItems();
Desc desc = new Desc();
desc.setDescription(role.getDescription());
desc.setId(role.getId());
desc.setIdType("role");
DescKey dk = descDao.insertDesc(desc, cra);
if (dk == null) {
ApplicationException.exception(
CommonMessageID.SERVICE_FAILURE.getMessage());
return;
}
for (RoleItem item : items) {
item.setRoleId(role.getId());
RoleItemKey uk = roleDao.insertItem(item, cra);
if (uk == null) {
ApplicationException.exception(
CommonMessageID.SERVICE_FAILURE.getMessage());
return;
}
}
}
@Override
@Transactional
public void updateRole(Context ctx, Role role) {
CommonRequestArgs cra = ctx.getRequest();
if (Strings.isNullOrEmpty(role.getId())) {
ApplicationException.exception(
CommonMessageID.MISSING_ARG.getMessage("roleId"));
}
if (Strings.isNullOrEmpty(role.getDescription())) {
ApplicationException.exception(
CommonMessageID.MISSING_ARG.getMessage("description"));
return;
}
DescKey dk = descDao.updateDesc(role, cra);
if (dk == null) {
ApplicationException.exception(
CommonMessageID.SERVICE_FAILURE.getMessage());
return;
}
roleDao.updateRole(role, cra);
}
public void setRoleDao(RoleDao roleDao) {
this.roleDao = roleDao;
}
@Override
@Transactional
public void deleteRole(Context ctx, RoleKey roleKey) {
CommonRequestArgs cra = ctx.getRequest();
if (!authDao.hasAccess(systemId, secFunc, AccessMode.WRITE, cra)) {
ApplicationException.exception(DAOMessageID.UNAUTHORIZED.getMessage());
}
roleKey.setIdType("role");
if (roleDao.isRoleRefered(roleKey.getId(), cra)) {
ResponseHandler rh = ctx.getResponseHandler();
rh.addMessage(AuthMessageID.CANNOT_DELETE_REFERED_ROLE.getMessage());
return;
}
descDao.deleteDesc(roleKey, cra);
roleDao.deleteRole(roleKey, cra);
}
public void setDescDao(DescDao descDao) {
this.descDao = descDao;
}
public void setSystemId(String systemId) {
this.systemId = systemId;
}
public void setSecFunc(String secFunc) {
this.secFunc = secFunc;
}
public void setAuthDao(AuthDao authDao) {
this.authDao = authDao;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy