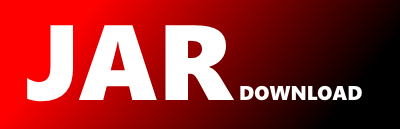
org.butor.dbauth.model.DefaultUserModel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of butor-auth-model Show documentation
Show all versions of butor-auth-model Show documentation
Butor Framework is a framework that enables fast and easy creation of HTTP services. (asynchronous and synchronous). It consists of several modules for different layers, such as data access, services, JSON serialization and test utilities.
It sits on top of popular and proven libraries such as Google Guava and Spring.
The project is released under APL 2.0
This project is an authentication module (Model).
package org.butor.dbauth.model;
import static com.google.common.base.Strings.isNullOrEmpty;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.UUID;
import java.util.concurrent.TimeUnit;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.butor.attrset.common.AttrSet;
import org.butor.attrset.dao.AttrSetDao;
import org.butor.attrset.util.Attributes;
import org.butor.attrset.util.Attributes.AttributesBuilder;
import org.butor.auth.common.AuthMessageID;
import org.butor.auth.common.SecurityConstants;
import org.butor.auth.common.user.ListUserCriteria;
import org.butor.auth.common.user.User;
import org.butor.auth.common.user.UserKey;
import org.butor.auth.common.user.UserModel;
import org.butor.auth.common.user.UserQuestions;
import org.butor.auth.common.user.UserServices;
import org.butor.auth.dao.UserDao;
import org.butor.checksum.CommonChecksumFunction;
import org.butor.json.CommonRequestArgs;
import org.butor.json.JsonHelper;
import org.butor.json.service.Context;
import org.butor.json.service.ResponseHandler;
import org.butor.ldap.LdapUserModel;
import org.butor.mail.EmailTemplate;
import org.butor.mail.IMailer;
import org.butor.utils.ApplicationException;
import org.butor.utils.CommonMessageID;
import org.butor.utils.StringUtil;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class DefaultUserModel implements UserServices, UserModel{
public static final String EMAIL_REGEX = "^[a-zA-Z0-9\\w\\.-]+@[a-zA-Z0-9\\w\\.-]+\\.[a-zA-Z0-9\\w\\.-]+$";
public static final Pattern emailPattern = Pattern.compile(EMAIL_REGEX);
protected UserDao userDao;
private Logger logger = LoggerFactory.getLogger(getClass());
protected IMailer mailer;
protected String fromRecipient;
protected AttrSetDao attrSetDao;
@Override
public void readUser(Context ctx, String id, String func) {
ResponseHandler rh = ctx.getResponseHandler();
CommonRequestArgs cra = ctx.getRequest();
User user = readUser(id, func, cra);
if (user == null) {
rh.addMessage(CommonMessageID.NOT_FOUND.getMessage());
return;
}
rh.addRow(user);
}
@Override
public void insertUser(Context ctx, User user) {
ResponseHandler rh = ctx.getResponseHandler();
CommonRequestArgs cra = ctx.getRequest();
validateUser(user);
if(isNullOrEmpty(user.getFullName())) {
user.setFullName(user.getDisplayName());
}
if (!isNullOrEmpty(user.getNewPwd())) {
user.setPwd(CommonChecksumFunction.SHA512.generateChecksum(user.getNewPwd()));
}
UserKey uk = userDao.insertUser(user, cra);
if (uk == null) {
rh.addMessage(CommonMessageID.SERVICE_FAILURE.getMessage());
return;
}
rh.addRow(uk);
}
@Override
public void updateUser(Context ctx, User user) {
ResponseHandler rh = ctx.getResponseHandler();
if (isNullOrEmpty(user.getEmail())) {
rh.addMessage(CommonMessageID.MISSING_ARG.getMessage("Email"));
return;
}
if (isNullOrEmpty(user.getId())) {
rh.addMessage(CommonMessageID.MISSING_ARG.getMessage("ID"));
return;
}
CommonRequestArgs cra = ctx.getRequest();
validateUser(user);
if(isNullOrEmpty(user.getFullName())) {
user.setFullName(user.getDisplayName());
}
if (!isNullOrEmpty(user.getNewPwd())) {
user.setPwd(CommonChecksumFunction.SHA512.generateChecksum(user.getNewPwd()));
}
UserKey uk = userDao.updateUser(user, cra);
if (uk == null) {
rh.addMessage(CommonMessageID.NOT_FOUND.getMessage());
return;
}
rh.addRow(uk);
}
@Override
public void deleteUser(Context ctx, UserKey userKey) {
CommonRequestArgs cra = ctx.getRequest();
User user = userDao.readUser(userKey.getId(), null, cra);
if (user == null) {
ApplicationException.exception(AuthMessageID.USER_NOT_FOUND.getMessage());
}
if (user.isActive()) {
ApplicationException.exception(AuthMessageID.USER_SHOULD_BE_INACTIVE_TO_BE_DELETED.getMessage());
}
userDao.deleteUser(userKey, cra);
}
@Override
public void listUser(Context ctx, ListUserCriteria criteria, String func) {
CommonRequestArgs cra = ctx.getRequest();
ResponseHandler rh = ctx.getResponseHandler();
List list = listUser(criteria, func, cra);
Iterator it = list.iterator();
while (it.hasNext()) {
User u = it.next();
u.setPwd(null);
rh.addRow(u);
}
}
protected List listUser(ListUserCriteria criteria, String func, CommonRequestArgs cra) {
return userDao.listUser(criteria, func, cra);
}
@Override
public void readQuestions(Context ctx, String id) {
ResponseHandler rh = ctx.getResponseHandler();
CommonRequestArgs cra = ctx.getRequest();
UserQuestions uq = userDao.readQuestions(id, cra);
if (uq == null) {
rh.addMessage(CommonMessageID.NOT_FOUND.getMessage());
return;
}
rh.addRow(uq);
}
@Override
public void updateQuestions(Context ctx, UserQuestions questions) {
ResponseHandler rh = ctx.getResponseHandler();
CommonRequestArgs cra = ctx.getRequest();
UserKey uk = userDao.updateQuestions(questions, cra);
if (uk == null) {
rh.addMessage(CommonMessageID.NOT_FOUND.getMessage());
return;
}
rh.addRow(uk);
}
@Override
public void updateState(Context ctx, User user) {
ResponseHandler rh = ctx.getResponseHandler();
CommonRequestArgs cra = ctx.getRequest();
UserKey uk = userDao.updateState(user, cra);
if (uk == null) {
rh.addMessage(CommonMessageID.NOT_FOUND.getMessage());
return;
}
rh.addRow(uk);
}
@Override
public void resetLogin(Context ctx, String id, String domain, boolean resetAndSendLink) {
ResponseHandler rh = ctx.getResponseHandler();
CommonRequestArgs cra = ctx.getRequest();
if (mailer == null && resetAndSendLink) {
logger.warn("Mailer in not set!");
throw ApplicationException.exception(AuthMessageID.RESET_PWD_FAILED.getMessage());
}
if (StringUtil.isEmpty(id)) {
logger.warn("Missing credential id arg");
throw ApplicationException.exception(AuthMessageID.RESET_PWD_FAILED.getMessage());
}
User user = userDao.readUser(id, SecurityConstants.SEC_FUNC_USERS, cra);
if (user == null) {
logger.warn("Unknown user {}", id);
ApplicationException.exception(AuthMessageID.RESET_PWD_FAILED.getMessage());
}
if (StringUtil.isEmpty(user.getEmail())) {
logger.warn(String.format("No user defined for id %s", id));
ApplicationException.exception(AuthMessageID.RESET_PWD_FAILED.getMessage());
}
String tokSeed = UUID.randomUUID().toString();
// gen token for reset
String resetToken = CommonChecksumFunction.SHA256.generateChecksumWithTTL(tokSeed, 2,
TimeUnit.HOURS);
user.setPwd(resetToken);
user.setActive(false);
user.setResetInProgress(true);
user.setMissedLogin(0);
/*
* if (!CommonChecksumFunction.SHA512.validateChecksum(cred.getPwd(), user.getPwd())) {
* logger.warn(String.format("Password do not match for user=%s", cred.getEmail())); throw
* ApplicationException.exception(PortalMessageID.RESET_PWD_FAILED.getMessage()); }
*/
UserKey uk = userDao.updateUser(user, cra);
if (uk.getId() == null) {
logger.warn(String.format("Failed to save new password for user=%s", id));
ApplicationException.exception(AuthMessageID.RESET_PWD_FAILED.getMessage());
}
String url = domain;
if (isNullOrEmpty(url)) {
url = cra.getDomain();
}
url += "/reset?t=" + resetToken + "&id=" + user.getId();
String lang = getUserLang(user, cra);
EmailTemplate et = getEmailTemplate("reset-login", lang, cra);
String msg = et.getMessage();
msg = msg.replace("{username}", user.getDisplayName());
msg = msg.replace("{link}", url);
String fromRecipient = et.getFromRecipient();
if (isNullOrEmpty(fromRecipient)) {
fromRecipient = this.fromRecipient;
}
logger.info(String.format("Sending reset link via email for user=%s\n%s", id, msg));
if (resetAndSendLink) {
mailer.sendMail(user.getEmail(), et.getSubject(), msg, fromRecipient);
} else {
rh.addRow(url);
}
}
@Override
public User readUser(String id, String func, CommonRequestArgs cra) {
return userDao.readUser(id, func, cra);
}
protected void validateUser(User user) {
if (user == null) {
ApplicationException.exception(CommonMessageID.MISSING_ARG.getMessage("User"));
}
if (isNullOrEmpty(user.getId())) {
ApplicationException.exception(CommonMessageID.MISSING_ARG.getMessage("ID"));
}
if (isNullOrEmpty(user.getEmail())) {
ApplicationException.exception(CommonMessageID.MISSING_ARG.getMessage("Email"));
}
Matcher matcher = emailPattern.matcher(user.getEmail());
if (!matcher.find()) {
ApplicationException.exception(CommonMessageID.INVALID_ARG.getMessage("Email"));
}
if (isNullOrEmpty(user.getFirstName())) {
ApplicationException.exception(CommonMessageID.MISSING_ARG.getMessage("First name"));
}
if (isNullOrEmpty(user.getLastName())) {
ApplicationException.exception(CommonMessageID.MISSING_ARG.getMessage("Last name"));
}
if (isNullOrEmpty(user.getDisplayName())) {
ApplicationException.exception(CommonMessageID.MISSING_ARG.getMessage("Display name"));
}
if (user.getFirmId() <= 0) {
ApplicationException.exception(CommonMessageID.MISSING_ARG.getMessage("Firm"));
}
if (!isNullOrEmpty(user.getNewPwd()) || !isNullOrEmpty(user.getNewPwdConf())) {
if (isNullOrEmpty(user.getNewPwd())) {
ApplicationException.exception(CommonMessageID.MISSING_ARG.getMessage("New password"));
}
if (isNullOrEmpty(user.getNewPwdConf())) {
ApplicationException.exception(CommonMessageID.MISSING_ARG.getMessage("Confirm new password"));
}
if (!user.getNewPwd().equals(user.getNewPwdConf())) {
ApplicationException.exception(CommonMessageID.INVALID_ARG.getMessage("new passwords do not match"));
}
}
validateId(user);
}
protected void validateId(User user) {
String id = user.getId();
//force id to lower case
user.setId(id.toLowerCase());
boolean emailId = emailPattern.matcher(id).find();
if (!emailId) {
ApplicationException.exception(AuthMessageID.USER_ID_SHOULD_BE_EMAIL.getMessage());
}
}
protected String getUserLang(User user, CommonRequestArgs cra) {
String lang = user.getLanguage();
if (isNullOrEmpty(lang)) {
// user lang is in his profile
Attributes attrs = new AttributesBuilder().setType("user").setId(user.getId())
.setAttrSetDao(attrSetDao).setCommonRequestArgs(cra).build();
if (attrs != null) {
lang = attrs.get("language", ".");
}
}
return lang;
}
protected EmailTemplate getEmailTemplate(String type, String lang, CommonRequestArgs cra) {
Attributes attrs = new AttributesBuilder().setType(type).setId("email-template")
.setAttrSetDao(attrSetDao).setCommonRequestArgs(cra).build();
if (isNullOrEmpty(lang)) {
lang = cra.getLang();
}
EmailTemplate det = null;
EmailTemplate et = null;
Collection list = attrs.list();
for (AttrSet as : list) {
if (as.getK1().equalsIgnoreCase(cra.getDomain()) &&
as.getK2().equalsIgnoreCase(lang)) {
et = new JsonHelper().deserialize(as.getValue(), EmailTemplate.class);
break;
}
// any default ?
if (as.getK1().equals("*") &&
as.getK2().equalsIgnoreCase(lang)) {
det = new JsonHelper().deserialize(as.getValue(), EmailTemplate.class);
}
}
if (et == null) {
et = det;
if (et == null) {
et = new EmailTemplate();
et.setFromRecipient(fromRecipient);
if (lang.equals("fr")) {
et.setSubject("Reinitialisation login portail");
et.setMessage("Votre login au portail a été reinitialisé à votre demande.\n" +
"SVP cliquer sur le lien plus bas et suivre les instructions.\n\n" +
"{link}");
} else {
et.setSubject("Portal login reset");
et.setMessage("Your login to the portal has been reset as you requested.\n" +
"Please click on the link bellow and follow the instructions.\n\n" +
"{link}");
}
}
}
return et;
}
public void setUserDao(UserDao userDao) {
this.userDao = userDao;
}
public void setLdapUserModel(LdapUserModel ldapUserModel) {
logger.warn("LdapUserModel is not used!");
}
public void setMailer(IMailer mailer) {
this.mailer = mailer;
}
public void setFromRecipient(String fromRecipient) {
this.fromRecipient = fromRecipient;
}
public void setAttrSetDao(AttrSetDao attrSetDao) {
this.attrSetDao = attrSetDao;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy