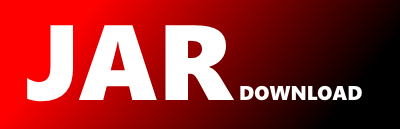
org.butor.json.util.MapJsonProxy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of butor-json Show documentation
Show all versions of butor-json Show documentation
This module enables fast and easy creation of sync., async., req./resp., req./resp. stream HTTP/json services.
/**
* Copyright 2013-2019 Butor Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.butor.json.util;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.google.common.collect.Lists;
import com.google.common.collect.Sets;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
/**
* Maps that hides the details of JSON serialization in a Map
*
* With this class you can serialize any Map and the value will be stored as
* String as JSON in the internal map within this object.
*
* @author tbussier
*
* @param
*/
public class MapJsonProxy implements Map {
final Map internal;
private Class typeOfT;
static final Gson GSON = new GsonBuilder().create();
public MapJsonProxy(Map internal,Class typeOfT) {
this.internal = internal;
this.typeOfT = typeOfT;
}
@Override
public int size() {
return internal.size();
}
@Override
public boolean isEmpty() {
return internal.isEmpty();
}
@Override
public boolean containsKey(Object key) {
return internal.containsKey(key);
}
@Override
public boolean containsValue(Object value) {
return this.values().contains(value);
}
@Override
public T get(Object key) {
String val = internal.get(key);
if (val != null) {
return GSON.fromJson(val, typeOfT);
}
return null;
}
@Override
public T put(String key, T value) {
String val = GSON.toJson(value, typeOfT);
internal.put(key,val);
return value;
}
@Override
public T remove(Object key) {
T t = this.get(key);
String ts = internal.remove(key);
if (ts == null) {
return null;
}
return t;
}
@Override
public void putAll(Map extends String, ? extends T> m) {
for (Entry extends String, ? extends T> e : m.entrySet()) {
this.put(e.getKey(),e.getValue());
}
}
@Override
public void clear() {
internal.clear();
}
@Override
public Set keySet() {
return internal.keySet();
}
@Override
public Collection values() {
List l = Lists.newArrayList();
for (String k : this.keySet()) {
T v = get(k);
if (v != null) {
l.add(v);
}
}
return l;
}
@Override
public Set> entrySet() {
Set> l = Sets.newLinkedHashSet();
for (final String k : internal.keySet()) {
final T v = get(k);
if (v != null) {
l.add(new Entry() {
@Override
public String getKey() {
return k;
}
@Override
public T getValue() {
return v;
}
@Override
public T setValue(T value) {
throw new UnsupportedOperationException("setValue not supported");
}
});
}
}
return l;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy