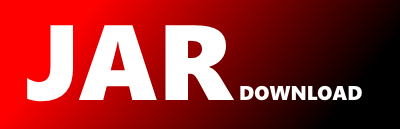
com.bybutter.sisyphus.test.internal.Case.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sisyphus-test Show documentation
Show all versions of sisyphus-test Show documentation
Test framework for testing gRPC apis in Sisyphus Framework
package com.bybutter.sisyphus.test.`internal`
import com.bybutter.sisyphus.collection.contentEquals
import com.bybutter.sisyphus.protobuf.AbstractMutableMessage
import com.bybutter.sisyphus.protobuf.FileSupport
import com.bybutter.sisyphus.protobuf.InternalProtoApi
import com.bybutter.sisyphus.protobuf.Message
import com.bybutter.sisyphus.protobuf.MessageSupport
import com.bybutter.sisyphus.protobuf.MutableMessage
import com.bybutter.sisyphus.protobuf.ProtoSupport
import com.bybutter.sisyphus.protobuf.coded.Reader
import com.bybutter.sisyphus.protobuf.coded.Writer
import com.bybutter.sisyphus.protobuf.primitives.DescriptorProto
import com.bybutter.sisyphus.protobuf.primitives.Duration
import com.bybutter.sisyphus.protobuf.primitives.FileDescriptorProto
import com.bybutter.sisyphus.reflect.uncheckedCast
import com.bybutter.sisyphus.test.TestCase
import com.bybutter.sisyphus.test.TestResult
import com.bybutter.sisyphus.test.TestStep
import kotlin.Any
import kotlin.Array
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.collections.MutableList
import kotlin.collections.MutableMap
import kotlin.lazy
public object CaseMetadata : FileSupport() {
public override val name: String = "sisyphus/test/case.proto"
public override val descriptor: FileDescriptorProto by lazy {
readDescriptor("sisyphus/test/case.pb")
}
public override fun children(): Array> = arrayOf(TestCase, TestStep, TestResult)
}
public interface MutableTestCase : MutableMessage, TestCase {
public override var name: String
public override val metadata: MutableMap
public override val steps: MutableList
public override val asserts: MutableList
public fun clearName(): String?
public fun clearMetadata(): Map
public fun clearSteps(): List
public fun clearAsserts(): List
}
@InternalProtoApi
internal class TestCaseImpl : AbstractMutableMessage(), MutableTestCase {
private var _hasName: Boolean = false
public override var name: String = ""
get() = if(_hasName) field else ""
set(`value`) {
field = value
_hasName = true
}
public override val metadata: MutableMap = mutableMapOf()
public override val steps: MutableList = mutableListOf()
public override val asserts: MutableList = mutableListOf()
public override fun hasName(): Boolean = _hasName
public override fun clearName(): String? {
if (!hasName()) return null
return name.also {
name = ""
_hasName = false
}
}
public override fun hasMetadata(): Boolean = metadata.isNotEmpty()
public override fun clearMetadata(): Map = metadata.toMap().also {
metadata.clear()
}
public override fun hasSteps(): Boolean = steps.isNotEmpty()
public override fun clearSteps(): List = steps.toList().also {
steps.clear()
}
public override fun hasAsserts(): Boolean = asserts.isNotEmpty()
public override fun clearAsserts(): List = asserts.toList().also {
asserts.clear()
}
public override fun support(): MessageSupport = TestCase
public override fun mergeWith(other: TestCase?): Unit {
other ?: return
val proto = other.toProto()
readFrom(Reader(proto.inputStream()), proto.size)
}
@InternalProtoApi
public override fun cloneMutable(): MutableTestCase = TestCaseImpl().apply {
mergeWith(this@TestCaseImpl)
}
public override fun clear(): Unit {
this.clearName()
this.clearMetadata()
this.clearSteps()
this.clearAsserts()
}
public override fun clearFieldInCurrent(fieldName: String): Any? = when(fieldName) {
"name" -> this.clearName()
"metadata" -> this.clearMetadata()
"steps" -> this.clearSteps()
"asserts" -> this.clearAsserts()
else -> clearFieldInExtensions(fieldName)
}
public override fun clearFieldInCurrent(fieldNumber: Int): Any? = when(fieldNumber) {
1 -> this.clearName()
2 -> this.clearMetadata()
3 -> this.clearSteps()
4 -> this.clearAsserts()
else -> clearFieldInExtensions(fieldNumber)
}
public override fun getFieldInCurrent(fieldName: String): T = when(fieldName) {
"name" -> this.name.uncheckedCast()
"metadata" -> this.metadata.uncheckedCast()
"steps" -> this.steps.uncheckedCast()
"asserts" -> this.asserts.uncheckedCast()
else -> getFieldInExtensions(fieldName)
}
public override fun getFieldInCurrent(fieldNumber: Int): T = when(fieldNumber) {
1 -> this.name.uncheckedCast()
2 -> this.metadata.uncheckedCast()
3 -> this.steps.uncheckedCast()
4 -> this.asserts.uncheckedCast()
else -> getFieldInExtensions(fieldNumber)
}
public override fun setFieldInCurrent(fieldName: String, `value`: T): Unit {
when(fieldName) {
"name" -> this.name = value.uncheckedCast()
"metadata" -> {
this.metadata.clear()
this.metadata.putAll(value.uncheckedCast())
}
"steps" -> {
this.steps.clear()
this.steps.addAll(value.uncheckedCast())
}
"asserts" -> {
this.asserts.clear()
this.asserts.addAll(value.uncheckedCast())
}
else -> setFieldInExtensions(fieldName, value)
}
}
public override fun setFieldInCurrent(fieldNumber: Int, `value`: T): Unit {
when(fieldNumber) {
1 -> this.name = value.uncheckedCast()
2 -> {
this.metadata.clear()
this.metadata.putAll(value.uncheckedCast())
}
3 -> {
this.steps.clear()
this.steps.addAll(value.uncheckedCast())
}
4 -> {
this.asserts.clear()
this.asserts.addAll(value.uncheckedCast())
}
else -> setFieldInExtensions(fieldNumber, value)
}
}
public override fun hasFieldInCurrent(fieldName: String): Boolean = when(fieldName) {
"name" -> this.hasName()
"metadata" -> this.hasMetadata()
"steps" -> this.hasSteps()
"asserts" -> this.hasAsserts()
else -> hasFieldInExtensions(fieldName)
}
public override fun hasFieldInCurrent(fieldNumber: Int): Boolean = when(fieldNumber) {
1 -> this.hasName()
2 -> this.hasMetadata()
3 -> this.hasSteps()
4 -> this.hasAsserts()
else -> hasFieldInExtensions(fieldNumber)
}
public override fun equalsMessage(other: TestCase): Boolean {
if (name != other.name) return false
if (!metadata.contentEquals(other.metadata)) return false
if (!steps.contentEquals(other.steps)) return false
if (!asserts.contentEquals(other.asserts)) return false
return true
}
public override fun computeHashCode(): Int {
var result = this.javaClass.hashCode()
if (hasName()) {
result = result * 37 + 1
result = result * 31 + this.name.hashCode()
}
for ((key, value) in metadata) {
result = result * 37 + 2
result = result * 31 + key.hashCode()
result = result * 31 + value.hashCode()
}
for (value in steps) {
result = result * 37 + 3
result = result * 31 + value.hashCode()
}
for (value in asserts) {
result = result * 37 + 4
result = result * 31 + value.hashCode()
}
return result
}
public override fun writeFields(writer: Writer): Unit {
if (hasName()) {
writer.tag(10).string(this.name)
}
this.metadata.forEach { (k, v) ->
writer.tag(18).beginLd().tag(10).string(k).tag(18).string(v).endLd() }
this.steps.forEach { writer.tag(26).message(it) }
this.asserts.forEach { writer.tag(34).string(it) }
}
@InternalProtoApi
public override fun readField(
reader: Reader,
`field`: Int,
wire: Int,
): Boolean {
when(field) {
1 -> this.name = reader.string()
2 -> reader.mapEntry({ it.string() }, { it.string() }) { k, v -> this.metadata[k] = v }
3 -> this.steps += TestStep.newMutable().apply { readFrom(reader) }
4 -> this.asserts += reader.string()
else -> return false
}
return true
}
}
public open class TestCaseSupport internal constructor() :
MessageSupport() {
public override val name: String
get() = ".sisyphus.test.TestCase"
public override val parent: FileSupport
get() = CaseMetadata
public override val descriptor: DescriptorProto by lazy {
CaseMetadata.descriptor.messageType.first{ it.name == "TestCase" }
}
@InternalProtoApi
public override fun newMutable(): MutableTestCase = TestCaseImpl()
}
public interface MutableTestStep : MutableMessage, TestStep {
public override var id: String
public override var name: String
public override val precondition: MutableList
public override var authority: String
public override var method: String
public override var input: Message<*, *>?
public override val inputScript: MutableList
public override val metadata: MutableMap
public override val metadataScript: MutableList
public override var insensitive: Boolean
public override val asserts: MutableList
public override var timeout: Duration?
public override var retryCount: Int
public override val retryCondition: MutableList
public fun clearId(): String?
public fun clearName(): String?
public fun clearPrecondition(): List
public fun clearAuthority(): String?
public fun clearMethod(): String?
public fun clearInput(): Message<*, *>?
public fun clearInputScript(): List
public fun clearMetadata(): Map
public fun clearMetadataScript(): List
public fun clearInsensitive(): Boolean?
public fun clearAsserts(): List
public fun clearTimeout(): Duration?
public fun clearRetryCount(): Int?
public fun clearRetryCondition(): List
}
@InternalProtoApi
internal class TestStepImpl : AbstractMutableMessage(), MutableTestStep {
private var _hasId: Boolean = false
public override var id: String = ""
get() = if(_hasId) field else ""
set(`value`) {
field = value
_hasId = true
}
private var _hasName: Boolean = false
public override var name: String = ""
get() = if(_hasName) field else ""
set(`value`) {
field = value
_hasName = true
}
public override val precondition: MutableList = mutableListOf()
private var _hasAuthority: Boolean = false
public override var authority: String = ""
get() = if(_hasAuthority) field else ""
set(`value`) {
field = value
_hasAuthority = true
}
private var _hasMethod: Boolean = false
public override var method: String = ""
get() = if(_hasMethod) field else ""
set(`value`) {
field = value
_hasMethod = true
}
public override var input: Message<*, *>? = null
set(`value`) {
field = value
}
public override val inputScript: MutableList = mutableListOf()
public override val metadata: MutableMap = mutableMapOf()
public override val metadataScript: MutableList = mutableListOf()
private var _hasInsensitive: Boolean = false
public override var insensitive: Boolean = false
get() = if(_hasInsensitive) field else false
set(`value`) {
field = value
_hasInsensitive = true
}
public override val asserts: MutableList = mutableListOf()
public override var timeout: Duration? = null
set(`value`) {
field = value
}
private var _hasRetryCount: Boolean = false
public override var retryCount: Int = 0
get() = if(_hasRetryCount) field else 0
set(`value`) {
field = value
_hasRetryCount = true
}
public override val retryCondition: MutableList = mutableListOf()
public override fun hasId(): Boolean = _hasId
public override fun clearId(): String? {
if (!hasId()) return null
return id.also {
id = ""
_hasId = false
}
}
public override fun hasName(): Boolean = _hasName
public override fun clearName(): String? {
if (!hasName()) return null
return name.also {
name = ""
_hasName = false
}
}
public override fun hasPrecondition(): Boolean = precondition.isNotEmpty()
public override fun clearPrecondition(): List = precondition.toList().also {
precondition.clear()
}
public override fun hasAuthority(): Boolean = _hasAuthority
public override fun clearAuthority(): String? {
if (!hasAuthority()) return null
return authority.also {
authority = ""
_hasAuthority = false
}
}
public override fun hasMethod(): Boolean = _hasMethod
public override fun clearMethod(): String? {
if (!hasMethod()) return null
return method.also {
method = ""
_hasMethod = false
}
}
public override fun hasInput(): Boolean = input != null
public override fun clearInput(): Message<*, *>? {
if (!hasInput()) return null
return input.also {
input = null
}
}
public override fun hasInputScript(): Boolean = inputScript.isNotEmpty()
public override fun clearInputScript(): List = inputScript.toList().also {
inputScript.clear()
}
public override fun hasMetadata(): Boolean = metadata.isNotEmpty()
public override fun clearMetadata(): Map = metadata.toMap().also {
metadata.clear()
}
public override fun hasMetadataScript(): Boolean = metadataScript.isNotEmpty()
public override fun clearMetadataScript(): List = metadataScript.toList().also {
metadataScript.clear()
}
public override fun hasInsensitive(): Boolean = _hasInsensitive
public override fun clearInsensitive(): Boolean? {
if (!hasInsensitive()) return null
return insensitive.also {
insensitive = false
_hasInsensitive = false
}
}
public override fun hasAsserts(): Boolean = asserts.isNotEmpty()
public override fun clearAsserts(): List = asserts.toList().also {
asserts.clear()
}
public override fun hasTimeout(): Boolean = timeout != null
public override fun clearTimeout(): Duration? {
if (!hasTimeout()) return null
return timeout.also {
timeout = null
}
}
public override fun hasRetryCount(): Boolean = _hasRetryCount
public override fun clearRetryCount(): Int? {
if (!hasRetryCount()) return null
return retryCount.also {
retryCount = 0
_hasRetryCount = false
}
}
public override fun hasRetryCondition(): Boolean = retryCondition.isNotEmpty()
public override fun clearRetryCondition(): List = retryCondition.toList().also {
retryCondition.clear()
}
public override fun support(): MessageSupport = TestStep
public override fun mergeWith(other: TestStep?): Unit {
other ?: return
val proto = other.toProto()
readFrom(Reader(proto.inputStream()), proto.size)
}
@InternalProtoApi
public override fun cloneMutable(): MutableTestStep = TestStepImpl().apply {
mergeWith(this@TestStepImpl)
}
public override fun clear(): Unit {
this.clearId()
this.clearName()
this.clearPrecondition()
this.clearAuthority()
this.clearMethod()
this.clearInput()
this.clearInputScript()
this.clearMetadata()
this.clearMetadataScript()
this.clearInsensitive()
this.clearAsserts()
this.clearTimeout()
this.clearRetryCount()
this.clearRetryCondition()
}
public override fun clearFieldInCurrent(fieldName: String): Any? = when(fieldName) {
"id" -> this.clearId()
"name" -> this.clearName()
"precondition" -> this.clearPrecondition()
"authority" -> this.clearAuthority()
"method" -> this.clearMethod()
"input" -> this.clearInput()
"input_script", "inputScript" -> this.clearInputScript()
"metadata" -> this.clearMetadata()
"metadata_script", "metadataScript" -> this.clearMetadataScript()
"insensitive" -> this.clearInsensitive()
"asserts" -> this.clearAsserts()
"timeout" -> this.clearTimeout()
"retry_count", "retryCount" -> this.clearRetryCount()
"retry_condition", "retryCondition" -> this.clearRetryCondition()
else -> clearFieldInExtensions(fieldName)
}
public override fun clearFieldInCurrent(fieldNumber: Int): Any? = when(fieldNumber) {
1 -> this.clearId()
2 -> this.clearName()
3 -> this.clearPrecondition()
4 -> this.clearAuthority()
5 -> this.clearMethod()
6 -> this.clearInput()
7 -> this.clearInputScript()
8 -> this.clearMetadata()
9 -> this.clearMetadataScript()
10 -> this.clearInsensitive()
11 -> this.clearAsserts()
12 -> this.clearTimeout()
13 -> this.clearRetryCount()
14 -> this.clearRetryCondition()
else -> clearFieldInExtensions(fieldNumber)
}
public override fun getFieldInCurrent(fieldName: String): T = when(fieldName) {
"id" -> this.id.uncheckedCast()
"name" -> this.name.uncheckedCast()
"precondition" -> this.precondition.uncheckedCast()
"authority" -> this.authority.uncheckedCast()
"method" -> this.method.uncheckedCast()
"input" -> this.input.uncheckedCast()
"input_script", "inputScript" -> this.inputScript.uncheckedCast()
"metadata" -> this.metadata.uncheckedCast()
"metadata_script", "metadataScript" -> this.metadataScript.uncheckedCast()
"insensitive" -> this.insensitive.uncheckedCast()
"asserts" -> this.asserts.uncheckedCast()
"timeout" -> this.timeout.uncheckedCast()
"retry_count", "retryCount" -> this.retryCount.uncheckedCast()
"retry_condition", "retryCondition" -> this.retryCondition.uncheckedCast()
else -> getFieldInExtensions(fieldName)
}
public override fun getFieldInCurrent(fieldNumber: Int): T = when(fieldNumber) {
1 -> this.id.uncheckedCast()
2 -> this.name.uncheckedCast()
3 -> this.precondition.uncheckedCast()
4 -> this.authority.uncheckedCast()
5 -> this.method.uncheckedCast()
6 -> this.input.uncheckedCast()
7 -> this.inputScript.uncheckedCast()
8 -> this.metadata.uncheckedCast()
9 -> this.metadataScript.uncheckedCast()
10 -> this.insensitive.uncheckedCast()
11 -> this.asserts.uncheckedCast()
12 -> this.timeout.uncheckedCast()
13 -> this.retryCount.uncheckedCast()
14 -> this.retryCondition.uncheckedCast()
else -> getFieldInExtensions(fieldNumber)
}
public override fun setFieldInCurrent(fieldName: String, `value`: T): Unit {
when(fieldName) {
"id" -> this.id = value.uncheckedCast()
"name" -> this.name = value.uncheckedCast()
"precondition" -> {
this.precondition.clear()
this.precondition.addAll(value.uncheckedCast())
}
"authority" -> this.authority = value.uncheckedCast()
"method" -> this.method = value.uncheckedCast()
"input" -> this.input = value.uncheckedCast()
"input_script", "inputScript" -> {
this.inputScript.clear()
this.inputScript.addAll(value.uncheckedCast())
}
"metadata" -> {
this.metadata.clear()
this.metadata.putAll(value.uncheckedCast())
}
"metadata_script", "metadataScript" -> {
this.metadataScript.clear()
this.metadataScript.addAll(value.uncheckedCast())
}
"insensitive" -> this.insensitive = value.uncheckedCast()
"asserts" -> {
this.asserts.clear()
this.asserts.addAll(value.uncheckedCast())
}
"timeout" -> this.timeout = value.uncheckedCast()
"retry_count", "retryCount" -> this.retryCount = value.uncheckedCast()
"retry_condition", "retryCondition" -> {
this.retryCondition.clear()
this.retryCondition.addAll(value.uncheckedCast())
}
else -> setFieldInExtensions(fieldName, value)
}
}
public override fun setFieldInCurrent(fieldNumber: Int, `value`: T): Unit {
when(fieldNumber) {
1 -> this.id = value.uncheckedCast()
2 -> this.name = value.uncheckedCast()
3 -> {
this.precondition.clear()
this.precondition.addAll(value.uncheckedCast())
}
4 -> this.authority = value.uncheckedCast()
5 -> this.method = value.uncheckedCast()
6 -> this.input = value.uncheckedCast()
7 -> {
this.inputScript.clear()
this.inputScript.addAll(value.uncheckedCast())
}
8 -> {
this.metadata.clear()
this.metadata.putAll(value.uncheckedCast())
}
9 -> {
this.metadataScript.clear()
this.metadataScript.addAll(value.uncheckedCast())
}
10 -> this.insensitive = value.uncheckedCast()
11 -> {
this.asserts.clear()
this.asserts.addAll(value.uncheckedCast())
}
12 -> this.timeout = value.uncheckedCast()
13 -> this.retryCount = value.uncheckedCast()
14 -> {
this.retryCondition.clear()
this.retryCondition.addAll(value.uncheckedCast())
}
else -> setFieldInExtensions(fieldNumber, value)
}
}
public override fun hasFieldInCurrent(fieldName: String): Boolean = when(fieldName) {
"id" -> this.hasId()
"name" -> this.hasName()
"precondition" -> this.hasPrecondition()
"authority" -> this.hasAuthority()
"method" -> this.hasMethod()
"input" -> this.hasInput()
"input_script", "inputScript" -> this.hasInputScript()
"metadata" -> this.hasMetadata()
"metadata_script", "metadataScript" -> this.hasMetadataScript()
"insensitive" -> this.hasInsensitive()
"asserts" -> this.hasAsserts()
"timeout" -> this.hasTimeout()
"retry_count", "retryCount" -> this.hasRetryCount()
"retry_condition", "retryCondition" -> this.hasRetryCondition()
else -> hasFieldInExtensions(fieldName)
}
public override fun hasFieldInCurrent(fieldNumber: Int): Boolean = when(fieldNumber) {
1 -> this.hasId()
2 -> this.hasName()
3 -> this.hasPrecondition()
4 -> this.hasAuthority()
5 -> this.hasMethod()
6 -> this.hasInput()
7 -> this.hasInputScript()
8 -> this.hasMetadata()
9 -> this.hasMetadataScript()
10 -> this.hasInsensitive()
11 -> this.hasAsserts()
12 -> this.hasTimeout()
13 -> this.hasRetryCount()
14 -> this.hasRetryCondition()
else -> hasFieldInExtensions(fieldNumber)
}
public override fun equalsMessage(other: TestStep): Boolean {
if (id != other.id) return false
if (name != other.name) return false
if (!precondition.contentEquals(other.precondition)) return false
if (authority != other.authority) return false
if (method != other.method) return false
if (input != other.input) return false
if (!inputScript.contentEquals(other.inputScript)) return false
if (!metadata.contentEquals(other.metadata)) return false
if (!metadataScript.contentEquals(other.metadataScript)) return false
if (insensitive != other.insensitive) return false
if (!asserts.contentEquals(other.asserts)) return false
if (timeout != other.timeout) return false
if (retryCount != other.retryCount) return false
if (!retryCondition.contentEquals(other.retryCondition)) return false
return true
}
public override fun computeHashCode(): Int {
var result = this.javaClass.hashCode()
if (hasId()) {
result = result * 37 + 1
result = result * 31 + this.id.hashCode()
}
if (hasName()) {
result = result * 37 + 2
result = result * 31 + this.name.hashCode()
}
for (value in precondition) {
result = result * 37 + 3
result = result * 31 + value.hashCode()
}
if (hasAuthority()) {
result = result * 37 + 4
result = result * 31 + this.authority.hashCode()
}
if (hasMethod()) {
result = result * 37 + 5
result = result * 31 + this.method.hashCode()
}
if (hasInput()) {
result = result * 37 + 6
result = result * 31 + this.input!!.hashCode()
}
for (value in inputScript) {
result = result * 37 + 7
result = result * 31 + value.hashCode()
}
for ((key, value) in metadata) {
result = result * 37 + 8
result = result * 31 + key.hashCode()
result = result * 31 + value.hashCode()
}
for (value in metadataScript) {
result = result * 37 + 9
result = result * 31 + value.hashCode()
}
if (hasInsensitive()) {
result = result * 37 + 10
result = result * 31 + this.insensitive.hashCode()
}
for (value in asserts) {
result = result * 37 + 11
result = result * 31 + value.hashCode()
}
if (hasTimeout()) {
result = result * 37 + 12
result = result * 31 + this.timeout!!.hashCode()
}
if (hasRetryCount()) {
result = result * 37 + 13
result = result * 31 + this.retryCount.hashCode()
}
for (value in retryCondition) {
result = result * 37 + 14
result = result * 31 + value.hashCode()
}
return result
}
public override fun writeFields(writer: Writer): Unit {
if (hasId()) {
writer.tag(10).string(this.id)
}
if (hasName()) {
writer.tag(18).string(this.name)
}
this.precondition.forEach { writer.tag(26).string(it) }
if (hasAuthority()) {
writer.tag(34).string(this.authority)
}
if (hasMethod()) {
writer.tag(42).string(this.method)
}
if (hasInput()) {
writer.tag(50).any(this.input)
}
this.inputScript.forEach { writer.tag(58).string(it) }
this.metadata.forEach { (k, v) ->
writer.tag(66).beginLd().tag(10).string(k).tag(18).string(v).endLd() }
this.metadataScript.forEach { writer.tag(74).string(it) }
if (hasInsensitive()) {
writer.tag(80).bool(this.insensitive)
}
this.asserts.forEach { writer.tag(90).string(it) }
if (hasTimeout()) {
writer.tag(98).message(this.timeout)
}
if (hasRetryCount()) {
writer.tag(104).int32(this.retryCount)
}
this.retryCondition.forEach { writer.tag(114).string(it) }
}
@InternalProtoApi
public override fun readField(
reader: Reader,
`field`: Int,
wire: Int,
): Boolean {
when(field) {
1 -> this.id = reader.string()
2 -> this.name = reader.string()
3 -> this.precondition += reader.string()
4 -> this.authority = reader.string()
5 -> this.method = reader.string()
6 -> this.input = reader.any()
7 -> this.inputScript += reader.string()
8 -> reader.mapEntry({ it.string() }, { it.string() }) { k, v -> this.metadata[k] = v }
9 -> this.metadataScript += reader.string()
10 -> this.insensitive = reader.bool()
11 -> this.asserts += reader.string()
12 -> this.timeout = Duration.newMutable().apply { readFrom(reader) }
13 -> this.retryCount = reader.int32()
14 -> this.retryCondition += reader.string()
else -> return false
}
return true
}
}
public open class TestStepSupport internal constructor() :
MessageSupport() {
public override val name: String
get() = ".sisyphus.test.TestStep"
public override val parent: FileSupport
get() = CaseMetadata
public override val descriptor: DescriptorProto by lazy {
CaseMetadata.descriptor.messageType.first{ it.name == "TestStep" }
}
@InternalProtoApi
public override fun newMutable(): MutableTestStep = TestStepImpl()
}
public interface MutableTestResult : MutableMessage, TestResult {
public override var step: TestStep?
public override var case: TestCase?
public override var method: String
public override var status: Int
public override var message: String
public override var input: Message<*, *>?
public override var output: Message<*, *>?
public override val headers: MutableMap
public override val trailers: MutableMap
public fun clearStep(): TestStep?
public fun clearCase(): TestCase?
public fun clearMethod(): String?
public fun clearStatus(): Int?
public fun clearMessage(): String?
public fun clearInput(): Message<*, *>?
public fun clearOutput(): Message<*, *>?
public fun clearHeaders(): Map
public fun clearTrailers(): Map
}
@InternalProtoApi
internal class TestResultImpl : AbstractMutableMessage(),
MutableTestResult {
public override var step: TestStep? = null
set(`value`) {
field = value
}
public override var case: TestCase? = null
set(`value`) {
field = value
}
private var _hasMethod: Boolean = false
public override var method: String = ""
get() = if(_hasMethod) field else ""
set(`value`) {
field = value
_hasMethod = true
}
private var _hasStatus: Boolean = false
public override var status: Int = 0
get() = if(_hasStatus) field else 0
set(`value`) {
field = value
_hasStatus = true
}
private var _hasMessage: Boolean = false
public override var message: String = ""
get() = if(_hasMessage) field else ""
set(`value`) {
field = value
_hasMessage = true
}
public override var input: Message<*, *>? = null
set(`value`) {
field = value
}
public override var output: Message<*, *>? = null
set(`value`) {
field = value
}
public override val headers: MutableMap = mutableMapOf()
public override val trailers: MutableMap = mutableMapOf()
public override fun hasStep(): Boolean = step != null
public override fun clearStep(): TestStep? {
if (!hasStep()) return null
return step.also {
step = null
}
}
public override fun hasCase(): Boolean = case != null
public override fun clearCase(): TestCase? {
if (!hasCase()) return null
return case.also {
case = null
}
}
public override fun hasMethod(): Boolean = _hasMethod
public override fun clearMethod(): String? {
if (!hasMethod()) return null
return method.also {
method = ""
_hasMethod = false
}
}
public override fun hasStatus(): Boolean = _hasStatus
public override fun clearStatus(): Int? {
if (!hasStatus()) return null
return status.also {
status = 0
_hasStatus = false
}
}
public override fun hasMessage(): Boolean = _hasMessage
public override fun clearMessage(): String? {
if (!hasMessage()) return null
return message.also {
message = ""
_hasMessage = false
}
}
public override fun hasInput(): Boolean = input != null
public override fun clearInput(): Message<*, *>? {
if (!hasInput()) return null
return input.also {
input = null
}
}
public override fun hasOutput(): Boolean = output != null
public override fun clearOutput(): Message<*, *>? {
if (!hasOutput()) return null
return output.also {
output = null
}
}
public override fun hasHeaders(): Boolean = headers.isNotEmpty()
public override fun clearHeaders(): Map = headers.toMap().also {
headers.clear()
}
public override fun hasTrailers(): Boolean = trailers.isNotEmpty()
public override fun clearTrailers(): Map = trailers.toMap().also {
trailers.clear()
}
public override fun support(): MessageSupport = TestResult
public override fun mergeWith(other: TestResult?): Unit {
other ?: return
val proto = other.toProto()
readFrom(Reader(proto.inputStream()), proto.size)
}
@InternalProtoApi
public override fun cloneMutable(): MutableTestResult = TestResultImpl().apply {
mergeWith(this@TestResultImpl)
}
public override fun clear(): Unit {
this.clearStep()
this.clearCase()
this.clearMethod()
this.clearStatus()
this.clearMessage()
this.clearInput()
this.clearOutput()
this.clearHeaders()
this.clearTrailers()
}
public override fun clearFieldInCurrent(fieldName: String): Any? = when(fieldName) {
"step" -> this.clearStep()
"case" -> this.clearCase()
"method" -> this.clearMethod()
"status" -> this.clearStatus()
"message" -> this.clearMessage()
"input" -> this.clearInput()
"output" -> this.clearOutput()
"headers" -> this.clearHeaders()
"trailers" -> this.clearTrailers()
else -> clearFieldInExtensions(fieldName)
}
public override fun clearFieldInCurrent(fieldNumber: Int): Any? = when(fieldNumber) {
1 -> this.clearStep()
2 -> this.clearCase()
3 -> this.clearMethod()
4 -> this.clearStatus()
5 -> this.clearMessage()
6 -> this.clearInput()
7 -> this.clearOutput()
8 -> this.clearHeaders()
9 -> this.clearTrailers()
else -> clearFieldInExtensions(fieldNumber)
}
public override fun getFieldInCurrent(fieldName: String): T = when(fieldName) {
"step" -> this.step.uncheckedCast()
"case" -> this.case.uncheckedCast()
"method" -> this.method.uncheckedCast()
"status" -> this.status.uncheckedCast()
"message" -> this.message.uncheckedCast()
"input" -> this.input.uncheckedCast()
"output" -> this.output.uncheckedCast()
"headers" -> this.headers.uncheckedCast()
"trailers" -> this.trailers.uncheckedCast()
else -> getFieldInExtensions(fieldName)
}
public override fun getFieldInCurrent(fieldNumber: Int): T = when(fieldNumber) {
1 -> this.step.uncheckedCast()
2 -> this.case.uncheckedCast()
3 -> this.method.uncheckedCast()
4 -> this.status.uncheckedCast()
5 -> this.message.uncheckedCast()
6 -> this.input.uncheckedCast()
7 -> this.output.uncheckedCast()
8 -> this.headers.uncheckedCast()
9 -> this.trailers.uncheckedCast()
else -> getFieldInExtensions(fieldNumber)
}
public override fun setFieldInCurrent(fieldName: String, `value`: T): Unit {
when(fieldName) {
"step" -> this.step = value.uncheckedCast()
"case" -> this.case = value.uncheckedCast()
"method" -> this.method = value.uncheckedCast()
"status" -> this.status = value.uncheckedCast()
"message" -> this.message = value.uncheckedCast()
"input" -> this.input = value.uncheckedCast()
"output" -> this.output = value.uncheckedCast()
"headers" -> {
this.headers.clear()
this.headers.putAll(value.uncheckedCast())
}
"trailers" -> {
this.trailers.clear()
this.trailers.putAll(value.uncheckedCast())
}
else -> setFieldInExtensions(fieldName, value)
}
}
public override fun setFieldInCurrent(fieldNumber: Int, `value`: T): Unit {
when(fieldNumber) {
1 -> this.step = value.uncheckedCast()
2 -> this.case = value.uncheckedCast()
3 -> this.method = value.uncheckedCast()
4 -> this.status = value.uncheckedCast()
5 -> this.message = value.uncheckedCast()
6 -> this.input = value.uncheckedCast()
7 -> this.output = value.uncheckedCast()
8 -> {
this.headers.clear()
this.headers.putAll(value.uncheckedCast())
}
9 -> {
this.trailers.clear()
this.trailers.putAll(value.uncheckedCast())
}
else -> setFieldInExtensions(fieldNumber, value)
}
}
public override fun hasFieldInCurrent(fieldName: String): Boolean = when(fieldName) {
"step" -> this.hasStep()
"case" -> this.hasCase()
"method" -> this.hasMethod()
"status" -> this.hasStatus()
"message" -> this.hasMessage()
"input" -> this.hasInput()
"output" -> this.hasOutput()
"headers" -> this.hasHeaders()
"trailers" -> this.hasTrailers()
else -> hasFieldInExtensions(fieldName)
}
public override fun hasFieldInCurrent(fieldNumber: Int): Boolean = when(fieldNumber) {
1 -> this.hasStep()
2 -> this.hasCase()
3 -> this.hasMethod()
4 -> this.hasStatus()
5 -> this.hasMessage()
6 -> this.hasInput()
7 -> this.hasOutput()
8 -> this.hasHeaders()
9 -> this.hasTrailers()
else -> hasFieldInExtensions(fieldNumber)
}
public override fun equalsMessage(other: TestResult): Boolean {
if (step != other.step) return false
if (case != other.case) return false
if (method != other.method) return false
if (status != other.status) return false
if (message != other.message) return false
if (input != other.input) return false
if (output != other.output) return false
if (!headers.contentEquals(other.headers)) return false
if (!trailers.contentEquals(other.trailers)) return false
return true
}
public override fun computeHashCode(): Int {
var result = this.javaClass.hashCode()
if (hasStep()) {
result = result * 37 + 1
result = result * 31 + this.step!!.hashCode()
}
if (hasCase()) {
result = result * 37 + 2
result = result * 31 + this.case!!.hashCode()
}
if (hasMethod()) {
result = result * 37 + 3
result = result * 31 + this.method.hashCode()
}
if (hasStatus()) {
result = result * 37 + 4
result = result * 31 + this.status.hashCode()
}
if (hasMessage()) {
result = result * 37 + 5
result = result * 31 + this.message.hashCode()
}
if (hasInput()) {
result = result * 37 + 6
result = result * 31 + this.input!!.hashCode()
}
if (hasOutput()) {
result = result * 37 + 7
result = result * 31 + this.output!!.hashCode()
}
for ((key, value) in headers) {
result = result * 37 + 8
result = result * 31 + key.hashCode()
result = result * 31 + value.hashCode()
}
for ((key, value) in trailers) {
result = result * 37 + 9
result = result * 31 + key.hashCode()
result = result * 31 + value.hashCode()
}
return result
}
public override fun writeFields(writer: Writer): Unit {
if (hasStep()) {
writer.tag(10).message(this.step)
}
if (hasCase()) {
writer.tag(18).message(this.case)
}
if (hasMethod()) {
writer.tag(26).string(this.method)
}
if (hasStatus()) {
writer.tag(32).int32(this.status)
}
if (hasMessage()) {
writer.tag(42).string(this.message)
}
if (hasInput()) {
writer.tag(50).any(this.input)
}
if (hasOutput()) {
writer.tag(58).any(this.output)
}
this.headers.forEach { (k, v) ->
writer.tag(66).beginLd().tag(10).string(k).tag(18).string(v).endLd() }
this.trailers.forEach { (k, v) ->
writer.tag(74).beginLd().tag(10).string(k).tag(18).string(v).endLd() }
}
@InternalProtoApi
public override fun readField(
reader: Reader,
`field`: Int,
wire: Int,
): Boolean {
when(field) {
1 -> this.step = TestStep.newMutable().apply { readFrom(reader) }
2 -> this.case = TestCase.newMutable().apply { readFrom(reader) }
3 -> this.method = reader.string()
4 -> this.status = reader.int32()
5 -> this.message = reader.string()
6 -> this.input = reader.any()
7 -> this.output = reader.any()
8 -> reader.mapEntry({ it.string() }, { it.string() }) { k, v -> this.headers[k] = v }
9 -> reader.mapEntry({ it.string() }, { it.string() }) { k, v -> this.trailers[k] = v }
else -> return false
}
return true
}
}
public open class TestResultSupport internal constructor() :
MessageSupport() {
public override val name: String
get() = ".sisyphus.test.TestResult"
public override val parent: FileSupport
get() = CaseMetadata
public override val descriptor: DescriptorProto by lazy {
CaseMetadata.descriptor.messageType.first{ it.name == "TestResult" }
}
@InternalProtoApi
public override fun newMutable(): MutableTestResult = TestResultImpl()
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy