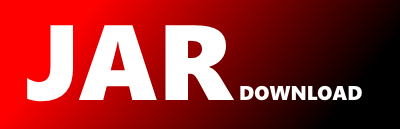
com.bytekast.sweettalk.SweetTalk.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sweet-talk Show documentation
Show all versions of sweet-talk Show documentation
A Sensible NetSuite SuiteTalk API wrapper
The newest version!
package com.bytekast.sweettalk
import com.bytekast.sweettalk.credentials.PasswordCredentials
import com.bytekast.sweettalk.credentials.TokenCredentials
import com.bytekast.sweettalk.search.SearchRequest
import com.bytekast.sweettalk.util.DomUtil
import com.bytekast.sweettalk.util.NamespaceUtil
import groovy.xml.XmlUtil
import wslite.soap.SOAPClient
class SweetTalk {
final XSI_NS = 'http://www.w3.org/2001/XMLSchema-instance'
final MSG_NS = 'urn:messages_2017_1.platform.webservices.netsuite.com'
final CORE_NS = 'urn:core_2017_1.platform.webservices.netsuite.com'
final SALES_NS = 'urn:sales_2017_1.transactions.webservices.netsuite.com'
final COMMON_NS = 'urn:common_2017_1.platform.webservices.netsuite.com'
private TokenCredentials credentials
SweetTalk(TokenCredentials credentials) {
this.credentials = credentials
}
Map login(PasswordCredentials passwordCredentials) {
def response = client.send(SOAPAction: 'login') {
header {
if (passwordCredentials.partnerId) {
partnerInfo('xmlns': MSG_NS) {
partnerId('xmlns': MSG_NS, passwordCredentials.partnerId)
}
}
applicationInfo('xmlns': MSG_NS) {
applicationId('xmlns': MSG_NS, passwordCredentials.applicationId)
}
}
body {
login('xmlns': MSG_NS) {
passport('xmlns': MSG_NS) {
email('xmlns': CORE_NS, passwordCredentials.loginEmail)
password('xmlns': CORE_NS, passwordCredentials.loginPassword)
account('xmlns': CORE_NS, passwordCredentials.accountId)
role('xmlns': CORE_NS, internalId: passwordCredentials.roleId, type: 'contactRole')
}
}
}
}
toMap(response.body.loginResponse)
}
Map getRecord(String type, String internalId) {
def response = client.send(SOAPAction: 'get') {
header {
tokenPassport('xmlns': MSG_NS) {
account(credentials.accountId)
consumerKey(credentials.consumerKey)
token(credentials.tokenId)
nonce(credentials.nonce)
timestamp(credentials.timestamp)
signature(algorithm: credentials.signature.algorithm, credentials.signature.value)
}
}
body {
get('xmlns': CORE_NS) {
baseRef(
internalId: internalId,
type: type,
'xsi:type': 'RecordRef',
'xmlns:xsi': 'http://www.w3.org/2001/XMLSchema-instance')
}
}
}
toMap(response.body.getResponse)
}
Map search(final SearchRequest searchRequest) {
def response = client.send(SOAPAction: 'search') {
header {
tokenPassport('xmlns': MSG_NS) {
account(credentials.accountId)
consumerKey(credentials.consumerKey)
token(credentials.tokenId)
nonce(credentials.nonce)
timestamp(credentials.timestamp)
signature(algorithm: credentials.signature.algorithm, credentials.signature.value)
}
searchPreferences() {
bodyFieldsOnly(false)
}
}
body {
search('xmlns': MSG_NS, 'xmlns:s': SALES_NS, 'xmlns:xsi': XSI_NS) {
searchRecord('xsi:type': "s:TransactionSearch") {
basic('xmlns': SALES_NS) {
if (searchRequest.lastModifiedDate) {
lastModifiedDate('xmlns': COMMON_NS, operator: searchRequest.lastModifiedDate.operator) {
searchRequest.lastModifiedDate.searchValues.each { v ->
searchValue('xmlns': CORE_NS, v)
}
}
}
if (searchRequest.status) {
status('xmlns': COMMON_NS, operator: searchRequest.status.operator) {
searchRequest.status.searchValues.each { v ->
searchValue('xmlns': CORE_NS, v)
}
}
}
if (searchRequest.type) {
type('xmlns': COMMON_NS, operator: searchRequest.type.operator) {
searchRequest.type.searchValues.each { v ->
searchValue('xmlns': CORE_NS, v)
}
}
}
}
}
}
}
}
toMap(response.body.searchResponse)
}
@Lazy
private SOAPClient client = {
def wsClient = new SOAPClient('https://webservices.netsuite.com/services/NetSuitePort_2017_1')
return wsClient
}()
private Map toMap(def xmlObj) {
xmlObj ? DomUtil.instance.xmlToMap(NamespaceUtil.instance.removeXmlNamespaces(XmlUtil.serialize(xmlObj))) : null
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy