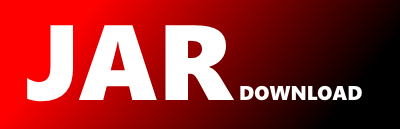
com.bytekast.sweettalk.credentials.TokenCredentials.groovy Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sweet-talk Show documentation
Show all versions of sweet-talk Show documentation
A Sensible NetSuite SuiteTalk API wrapper
The newest version!
package com.bytekast.sweettalk.credentials
import groovy.transform.CompileStatic
import groovy.transform.Immutable
import groovy.transform.ToString
import groovy.transform.builder.Builder
import groovy.transform.builder.SimpleStrategy
import javax.crypto.Mac
import javax.crypto.spec.SecretKeySpec
import java.time.Instant
import java.util.concurrent.ThreadLocalRandom
@CompileStatic
@Builder(builderStrategy = SimpleStrategy, prefix = '')
@ToString(includePackage = false, includeFields = true)
@Immutable
class TokenCredentials {
String roleId
String applicationId
String accountId
String consumerKey
String consumerSecret
String tokenId
String tokenSecret
@Lazy String nonce = { buildNonce(20) }()
@Lazy String timestamp = { Instant.now().epochSecond }()
Signature getSignature() {
def sigString = "${accountId}&${consumerKey}&${tokenId}&${nonce}&${timestamp}"
def keyString = "${consumerSecret}&${tokenSecret}"
Mac mac = Mac.getInstance("HmacSHA256")
SecretKeySpec secretKeySpec = new SecretKeySpec(keyString.getBytes(), "HmacSHA256")
mac.init(secretKeySpec)
byte[] digest = mac.doFinal(sigString.getBytes())
def encodedDigest = digest.encodeBase64()
new Signature().value(encodedDigest.toString()).algorithm("HMAC_SHA256")
}
private static String buildNonce(int size) {
StringBuilder builder = new StringBuilder()
while (size > 0) {
int c = ThreadLocalRandom.current().nextInt(0, ALPHA_NUMERIC_STRING.length())
builder.append(ALPHA_NUMERIC_STRING[c])
size--
}
return builder.toString()
}
private static final String ALPHA_NUMERIC_STRING = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ0123456789"
}
@CompileStatic
@Builder(builderStrategy = SimpleStrategy, prefix = '')
@ToString(includePackage = false, includeFields = true)
@Immutable
class Signature {
String value
String algorithm
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy