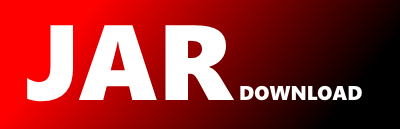
com.ca.apim.gateway.cagatewayconfig.BuildEnvironmentBundleTask Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gateway-policy-plugin Show documentation
Show all versions of gateway-policy-plugin Show documentation
The gateway-policy-plugin enables developing gateway configuration.
The newest version!
/*
* Copyright (c) 2018 CA. All rights reserved.
* This software may be modified and distributed under the terms
* of the MIT license. See the LICENSE file for details.
*/
package com.ca.apim.gateway.cagatewayconfig;
import com.ca.apim.gateway.cagatewayconfig.beans.BundleConfig;
import com.ca.apim.gateway.cagatewayconfig.environment.BundleCache;
import com.ca.apim.gateway.cagatewayconfig.environment.EnvironmentBundleCreator;
import com.ca.apim.gateway.cagatewayconfig.environment.MissingEnvironmentException;
import com.ca.apim.gateway.cagatewayconfig.util.environment.EnvironmentConfigurationUtils;
import com.ca.apim.gateway.cagatewayconfig.util.injection.InjectionRegistry;
import org.apache.commons.lang3.StringUtils;
import org.gradle.api.DefaultTask;
import org.gradle.api.file.DirectoryProperty;
import org.gradle.api.provider.Property;
import org.gradle.api.tasks.*;
import javax.inject.Inject;
import java.io.File;
import java.util.HashMap;
import java.util.Map;
import static com.ca.apim.gateway.cagatewayconfig.environment.EnvironmentBundleCreationMode.PLUGIN;
import static com.ca.apim.gateway.cagatewayconfig.util.file.DocumentFileUtils.*;
import static com.ca.apim.gateway.cagatewayconfig.util.gateway.BuilderUtils.removeAllSpecialChars;
import static com.ca.apim.gateway.cagatewayconfig.util.injection.InjectionRegistry.getInstance;
import static org.apache.commons.lang3.StringUtils.EMPTY;
/**
* The BuildEnvironmentBundle task will grab provided environment properties and build a bundle.
*/
public class BuildEnvironmentBundleTask extends DefaultTask {
private final DirectoryProperty into;
private final EnvironmentConfigurationUtils environmentConfigurationUtils;
private final DirectoryProperty configFolder;
private final Property configName;
private final Property
© 2015 - 2024 Weber Informatics LLC | Privacy Policy