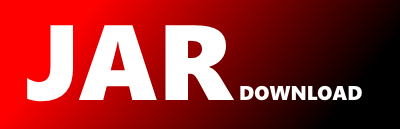
com.capitalone.dashboard.ApiSettings Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of api-audit Show documentation
Show all versions of api-audit Show documentation
Hygieia Audit Rest API Layer
package com.capitalone.dashboard;
import org.apache.commons.collections4.CollectionUtils;
import org.apache.commons.lang3.StringUtils;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.stereotype.Component;
import java.util.ArrayList;
import java.util.List;
import java.util.stream.Collectors;
@Component
@ConfigurationProperties
public class ApiSettings {
/**
* TODO The property name 'key' is too vague. This key is used only for encryption. Would suggest to rename it to
* encryptionKey to be specific. For now (for backwards compatibility) keeping it as it was.
*/
private String key;
@Value("${corsEnabled:false}")
private boolean corsEnabled;
private String corsWhitelist;
private String peerReviewContexts;
private String peerReviewApprovalText;
private List serviceAccountOU;
private String commitLogIgnoreAuditRegEx;
@Value("${maxDaysRangeForQuery:60}") // 60 days max
private long maxDaysRangeForQuery;
@Value("${logRequest:false}")
private boolean logRequest;
private String featureIDPattern;
@Value("${traceabilityThreshold:80.0}")
private double traceabilityThreshold;
@Value("${testResultThreshold:95.0}")
private double testResultThreshold;
private String featureTestResultThreshold;
private List validStoryStatus;
@Value("${testResultSuccessPriority:Low}")
public String testResultSuccessPriority;
@Value("${testResultFailurePriority:High}")
public String testResultFailurePriority;
@Value("${testResultSkippedPriority:High}")
public String testResultSkippedPriority;
private String serviceAccountRegEx;
@Value("${highSecurityVulnerabilitiesAge:0}")
private int highSecurityVulnerabilitiesAge;
@Value("${criticalSecurityVulnerabilitiesAge:0}")
private int criticalSecurityVulnerabilitiesAge;
@Value("${highLicenseVulnerabilitiesAge:0}")
private int highLicenseVulnerabilitiesAge;
@Value("${criticalLicenseVulnerabilitiesAge:0}")
private int criticalLicenseVulnerabilitiesAge;
private List buildStageRegEx;
private List ldapdnCheckIgnoredAuthorTypes = new ArrayList<>();
private String thirdPartyRegex;
private List ignoreEndPoints = new ArrayList();
private List ignoreApiUsers = new ArrayList();
private List mergeCommitFromTargetBranchRegEx;
private List validStaticSecurityScanTypes = new ArrayList<>();
@Value("${contextFactory:com.sun.jndi.ldap.LdapCtxFactory}")
private String contextFactory;
@Value("${contextProtocol:ssl}")
private String contextProtocol;
@Value("${contextSecurityAuthentication:simple}")
private String contextSecurityAuthentication;
@Value("${contextConnectTimeout:15000}")
private String contextConnectTimeout;
@Value("${enrichCommits:false}")
private boolean enrichCommits;
public String getKey() {
return key;
}
public void setKey(final String key) {
this.key = key;
}
public boolean isCorsEnabled() {
return corsEnabled;
}
public void setCorsEnabled(boolean corsEnabled) {
this.corsEnabled = corsEnabled;
}
public String getCorsWhitelist() {
return corsWhitelist;
}
public void setCorsWhitelist(String corsWhitelist) {
this.corsWhitelist = corsWhitelist;
}
public String getPeerReviewContexts() {
return peerReviewContexts;
}
public void setPeerReviewContexts(String peerReviewContexts) {
this.peerReviewContexts = peerReviewContexts;
}
public boolean isLogRequest() {
return logRequest;
}
public void setLogRequest(boolean logRequest) {
this.logRequest = logRequest;
}
public long getMaxDaysRangeForQuery() {
return maxDaysRangeForQuery;
}
public void setMaxDaysRangeForQuery(long maxDaysRangeForQuery) {
this.maxDaysRangeForQuery = maxDaysRangeForQuery;
}
public String getPeerReviewApprovalText() {
return peerReviewApprovalText;
}
public void setPeerReviewApprovalText(String peerReviewApprovalText) {
this.peerReviewApprovalText = peerReviewApprovalText;
}
public List getServiceAccountOU() {
return serviceAccountOU;
}
public void setServiceAccountOU(List serviceAccountOU) {
this.serviceAccountOU = serviceAccountOU;
}
public String getFeatureIDPattern() {
return featureIDPattern;
}
public void setFeatureIDPattern(String featureIDPattern) {
this.featureIDPattern = featureIDPattern;
}
public double getTraceabilityThreshold() {
return traceabilityThreshold;
}
public void setTraceabilityThreshold(double traceabilityThreshold) {
this.traceabilityThreshold = traceabilityThreshold;
}
public List getValidStoryStatus() {
return validStoryStatus;
}
public void setValidStoryStatus(List validStoryStatus) {
this.validStoryStatus = validStoryStatus;
}
public String getCommitLogIgnoreAuditRegEx() {
return commitLogIgnoreAuditRegEx;
}
public void setCommitLogIgnoreAuditRegEx(String commitLogIgnoreAuditRegEx) {
this.commitLogIgnoreAuditRegEx = commitLogIgnoreAuditRegEx;
}
public String getServiceAccountRegEx() {
return serviceAccountRegEx;
}
public void setServiceAccountRegEx(String serviceAccountRegEx) {
this.serviceAccountRegEx = serviceAccountRegEx;
}
public void setTestResultSuccessPriority(String testResultSuccessPriority) {
this.testResultSuccessPriority = testResultSuccessPriority;
}
public String getTestResultSuccessPriority() {
return testResultSuccessPriority;
}
public void setTestResultFailurePriority(String testResultFailurePriority) {
this.testResultFailurePriority = testResultFailurePriority;
}
public void setTestResultSkippedPriority(String testResultSkippedPriority) {
this.testResultSkippedPriority = testResultSkippedPriority;
}
public String getTestResultFailurePriority() {
return testResultFailurePriority;
}
public String getTestResultSkippedPriority() {
return testResultSkippedPriority;
}
public double getTestResultThreshold() {
return testResultThreshold;
}
public void setTestResultThreshold(double testResultThreshold) {
this.testResultThreshold = testResultThreshold;
}
public int getHighSecurityVulnerabilitiesAge() {
return highSecurityVulnerabilitiesAge;
}
public void setHighSecurityVulnerabilitiesAge(int highSecurityVulnerabilitiesAge) {
this.highSecurityVulnerabilitiesAge = highSecurityVulnerabilitiesAge;
}
public int getCriticalSecurityVulnerabilitiesAge() {
return criticalSecurityVulnerabilitiesAge;
}
public void setCriticalSecurityVulnerabilitiesAge(int criticalSecurityVulnerabilitiesAge) {
this.criticalSecurityVulnerabilitiesAge = criticalSecurityVulnerabilitiesAge;
}
public int getHighLicenseVulnerabilitiesAge() {
return highLicenseVulnerabilitiesAge;
}
public void setHighLicenseVulnerabilitiesAge(int highLicenseVulnerabilitiesAge) {
this.highLicenseVulnerabilitiesAge = highLicenseVulnerabilitiesAge;
}
public int getCriticalLicenseVulnerabilitiesAge() {
return criticalLicenseVulnerabilitiesAge;
}
public void setCriticalLicenseVulnerabilitiesAge(int criticalLicenseVulnerabilitiesAge) {
this.criticalLicenseVulnerabilitiesAge = criticalLicenseVulnerabilitiesAge;
}
public List getBuildStageRegEx() {
return buildStageRegEx;
}
public void setBuildStageRegEx(List buildStageRegEx) {
this.buildStageRegEx = buildStageRegEx;
}
public List getLdapdnCheckIgnoredAuthorTypes() {
return ldapdnCheckIgnoredAuthorTypes;
}
public void setLdapdnCheckIgnoredAuthorTypes(List ldapdnCheckIgnoredAuthorTypes) {
this.ldapdnCheckIgnoredAuthorTypes = ldapdnCheckIgnoredAuthorTypes;
}
public String getThirdPartyRegex() {
return thirdPartyRegex;
}
public void setThirdPartyRegex(String thirdPartyRegex) {
this.thirdPartyRegex = thirdPartyRegex;
}
public List getIgnoreEndPoints() { return ignoreEndPoints; }
public void setIgnoreEndPoints(List ignoreEndPoints) { this.ignoreEndPoints = ignoreEndPoints; }
public List getIgnoreApiUsers() { return ignoreApiUsers; }
public void setIgnoreApiUsers(List ignoreApiUsers) { this.ignoreApiUsers = ignoreApiUsers; }
public boolean checkIgnoreEndPoint(String endPointURI) {
if(CollectionUtils.isEmpty(this.ignoreEndPoints)) return false;
List matchingElements = ignoreEndPoints.parallelStream().filter (str -> StringUtils.contains(endPointURI.toLowerCase(), str)).collect(Collectors.toList());
return CollectionUtils.isNotEmpty(matchingElements);
}
public boolean checkIgnoreApiUser(String apiUser) {
if(CollectionUtils.isEmpty(this.ignoreApiUsers)) return false;
List matchingElements = ignoreApiUsers.parallelStream().filter (str -> StringUtils.equalsIgnoreCase(apiUser, str)).collect(Collectors.toList());
return CollectionUtils.isNotEmpty(matchingElements);
}
public List getMergeCommitFromTargetBranchRegEx() { return mergeCommitFromTargetBranchRegEx; }
public void setMergeCommitFromTargetBranchRegEx(List mergeCommitFromTargetBranchRegEx) { this.mergeCommitFromTargetBranchRegEx = mergeCommitFromTargetBranchRegEx; }
public List getValidStaticSecurityScanTypes() { return validStaticSecurityScanTypes; }
public void setValidStaticSecurityScanTypes(List validStaticSecurityScanTypes) { this.validStaticSecurityScanTypes = validStaticSecurityScanTypes; }
public String getContextFactory() {
return contextFactory;
}
public void setContextFactory(String contextFactory) {
this.contextFactory = contextFactory;
}
public String getContextProtocol() {
return contextProtocol;
}
public void setContextProtocol(String contextProtocol) {
this.contextProtocol = contextProtocol;
}
public String getContextSecurityAuthentication() {
return contextSecurityAuthentication;
}
public void setContextSecurityAuthentication(String contextSecurityAuthentication) {
this.contextSecurityAuthentication = contextSecurityAuthentication;
}
public boolean isEnrichCommits() {
return enrichCommits;
}
public void setEnrichCommits(boolean enrichCommits) {
this.enrichCommits = enrichCommits;
}
public String getContextConnectTimeout() {
return contextConnectTimeout;
}
public void setContextConnectTimeout(String contextConnectTimeout) {
this.contextConnectTimeout = contextConnectTimeout;
}
public String getFeatureTestResultThreshold() {
return featureTestResultThreshold;
}
public void setFeatureTestResultThreshold(String featureTestResultThreshold) {
this.featureTestResultThreshold = featureTestResultThreshold;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy