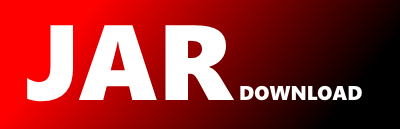
com.capitalone.dashboard.model.Dashboard Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Core package shared by API layer and Microservices
package com.capitalone.dashboard.model;
import org.springframework.data.mongodb.core.mapping.Document;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* A collection of widgets, collectors and application components that represent a software
* project under development and/or in production use.
*
*/
@Document(collection="dashboards")
public class Dashboard extends BaseModel {
private String template;
private String title;
private List widgets = new ArrayList<>();
private String owner;
private DashboardType type;
private Application application;
Dashboard() {
}
public Dashboard(String template, String title, Application application,String owner, DashboardType type) {
this.template = template;
this.title = title;
this.application = application;
this.owner = owner;
this.type = type;
}
public String getTemplate() {
return template;
}
public void setTemplate(String template) {
this.template = template;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public Application getApplication() {
return application;
}
public void setApplication(Application application) {
this.application = application;
}
public List getWidgets() {
return widgets;
}
public String getOwner() {
return owner;
}
public void setOwner(String owner) {
this.owner = owner;
}
public DashboardType getType(){ return this.type; }
public void setType(DashboardType type) { this.type = type; }
/**
* Finds the mapped names for each stage type from the widget options
* @return
*/
public Map findEnvironmentMappings(){
Map environmentMappings = null;
for(Widget widget : this.getWidgets()) {
if (widget.getName().equalsIgnoreCase("pipeline")) {
environmentMappings = (Map) widget.getOptions().get("mappings");
}
}
Map stageTypeToEnvironmentNameMap = new HashMap<>();
if(environmentMappings != null && !environmentMappings.isEmpty()){
for (Map.Entry mapping : environmentMappings.entrySet()) {
stageTypeToEnvironmentNameMap.put(PipelineStageType.fromString((String) mapping.getKey()), (String) mapping.getValue());
}
}
return stageTypeToEnvironmentNameMap;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy