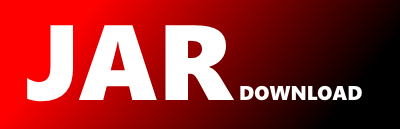
com.capitalone.dashboard.model.Build Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of core Show documentation
Show all versions of core Show documentation
Core package shared by API layer and Microservices
package com.capitalone.dashboard.model;
import org.bson.types.ObjectId;
import org.springframework.data.mongodb.core.mapping.Document;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
/**
* The result of a Continuous Integration build execution. Typically produces binary artifacts.
* Often triggered by one or more SCM commits.
*
* Possible collectors:
* Hudson (in scope)
* Team City
* TFS
* Go
* Bamboo
* TravisCI
*
*/
@Document(collection="builds")
public class Build extends BaseModel {
private ObjectId collectorItemId;
private long timestamp;
private String number;
private String buildUrl;
private long startTime;
private long endTime;
private long duration;
private BuildStatus buildStatus;
private String startedBy;
private String log;
private List codeRepos = new ArrayList<>();
private List sourceChangeSet = new ArrayList<>();
private List stages = new LinkedList<>();
private Map deployMetadata = new HashMap<>();
private Map additionalData = new HashMap<>();
public ObjectId getCollectorItemId() {
return collectorItemId;
}
public void setCollectorItemId(ObjectId collectorItemId) {
this.collectorItemId = collectorItemId;
}
public void setSourceChangeSet(List sourceChangeSet) {
this.sourceChangeSet = sourceChangeSet;
}
public long getTimestamp() {
return timestamp;
}
public void setTimestamp(long timestamp) {
this.timestamp = timestamp;
}
public String getNumber() {
return number;
}
public void setNumber(String number) {
this.number = number;
}
public String getBuildUrl() {
return buildUrl;
}
public void setBuildUrl(String buildUrl) {
this.buildUrl = buildUrl;
}
public long getStartTime() {
return startTime;
}
public void setStartTime(long startTime) {
this.startTime = startTime;
}
public long getEndTime() {
return endTime;
}
public void setEndTime(long endTime) {
this.endTime = endTime;
}
public long getDuration() {
return duration;
}
public void setDuration(long duration) {
this.duration = duration;
}
public BuildStatus getBuildStatus() {
return buildStatus;
}
public void setBuildStatus(BuildStatus buildStatus) {
this.buildStatus = buildStatus;
}
public String getStartedBy() {
return startedBy;
}
public void setStartedBy(String startedBy) {
this.startedBy = startedBy;
}
public String getLog() {
return log;
}
public void setLog(String log) {
this.log = log;
}
public List getSourceChangeSet() {
return sourceChangeSet;
}
public void addSourceChangeSet(SCM scm) {
getSourceChangeSet().add(scm);
}
public List getCodeRepos() {
return codeRepos;
}
public void setCodeRepos(List codeRepos) {
this.codeRepos = codeRepos;
}
public List getStages() {
return stages;
}
public void setStages(List stages) {
this.stages = stages;
}
public Map getDeployMetadata() { return deployMetadata; }
public void setDeployMetadata(Map deployMetadata) { this.deployMetadata = deployMetadata; }
public Map getAdditionalData() {
return additionalData;
}
public void setAdditionalData(Map additionalData) {
this.additionalData = additionalData;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy