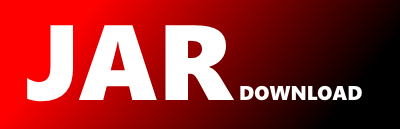
com.capitalone.dashboard.service.GitHubServiceImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of github-graphql-scm-collector Show documentation
Show all versions of github-graphql-scm-collector Show documentation
Github Collector Microservice collecting stats from Github using graphql
package com.capitalone.dashboard.service;
import com.capitalone.dashboard.collector.GitHubCollectorTask;
import com.capitalone.dashboard.model.Collector;
import com.capitalone.dashboard.model.GitHubRepo;
import com.capitalone.dashboard.repository.BaseCollectorRepository;
import com.capitalone.dashboard.repository.GitHubRepoRepository;
import org.apache.commons.collections.CollectionUtils;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.stereotype.Component;
import java.util.List;
import java.util.Objects;
@Component
public class GitHubServiceImpl implements GitHubService {
private static final Log LOG = LogFactory.getLog(GitHubServiceImpl.class);
private final BaseCollectorRepository collectorRepository;
private final GitHubRepoRepository gitHubRepoRepository;
private static final String GITHUB_COLLECTOR_NAME = "GitHub";
@Autowired
public GitHubServiceImpl(BaseCollectorRepository collectorRepository,
GitHubRepoRepository gitHubRepoRepository,
GitHubCollectorTask gitHubCollectorTask) {
this.collectorRepository = collectorRepository;
this.gitHubRepoRepository = gitHubRepoRepository;
}
public ResponseEntity cleanup() {
Collector collector = collectorRepository.findByName(GITHUB_COLLECTOR_NAME);
if (Objects.isNull(collector))
return ResponseEntity.status(HttpStatus.OK).body(GITHUB_COLLECTOR_NAME + " collector is not found");
List repos = gitHubRepoRepository.findObsoleteGitHubRepos(collector.getId());
if (CollectionUtils.isEmpty(repos))
return ResponseEntity.status(HttpStatus.OK).body("No more Obsolete GitHub repo found");
int count = repos.size();
gitHubRepoRepository.delete(repos);
LOG.info(GITHUB_COLLECTOR_NAME + " cleanup - " + count + " obsolete GitHub repo's deleted. ");
return ResponseEntity
.status(HttpStatus.OK)
.body(GITHUB_COLLECTOR_NAME + " cleanup - " + count + " obsolete GitHub repo's deleted. ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy