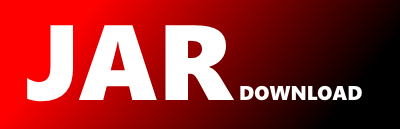
org.fabric3.fabric.container.wire.TransformerInterceptor Maven / Gradle / Ivy
The newest version!
/*
* Fabric3
* Copyright (c) 2009-2015 Metaform Systems
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.fabric3.fabric.container.wire;
import org.fabric3.api.host.Fabric3Exception;
import org.fabric3.spi.container.invocation.Message;
import org.fabric3.spi.container.wire.Interceptor;
import org.fabric3.spi.transform.Transformer;
import org.oasisopen.sca.ServiceRuntimeException;
/**
* Converts the input parameters of an invocation to a target format and the output parameters from the target format by delegating to underlying transformers.
*/
public class TransformerInterceptor implements Interceptor {
private Transformer
© 2015 - 2025 Weber Informatics LLC | Privacy Policy